filmov
tv
Protocols vs ABCs in Python - When to Use Which One?
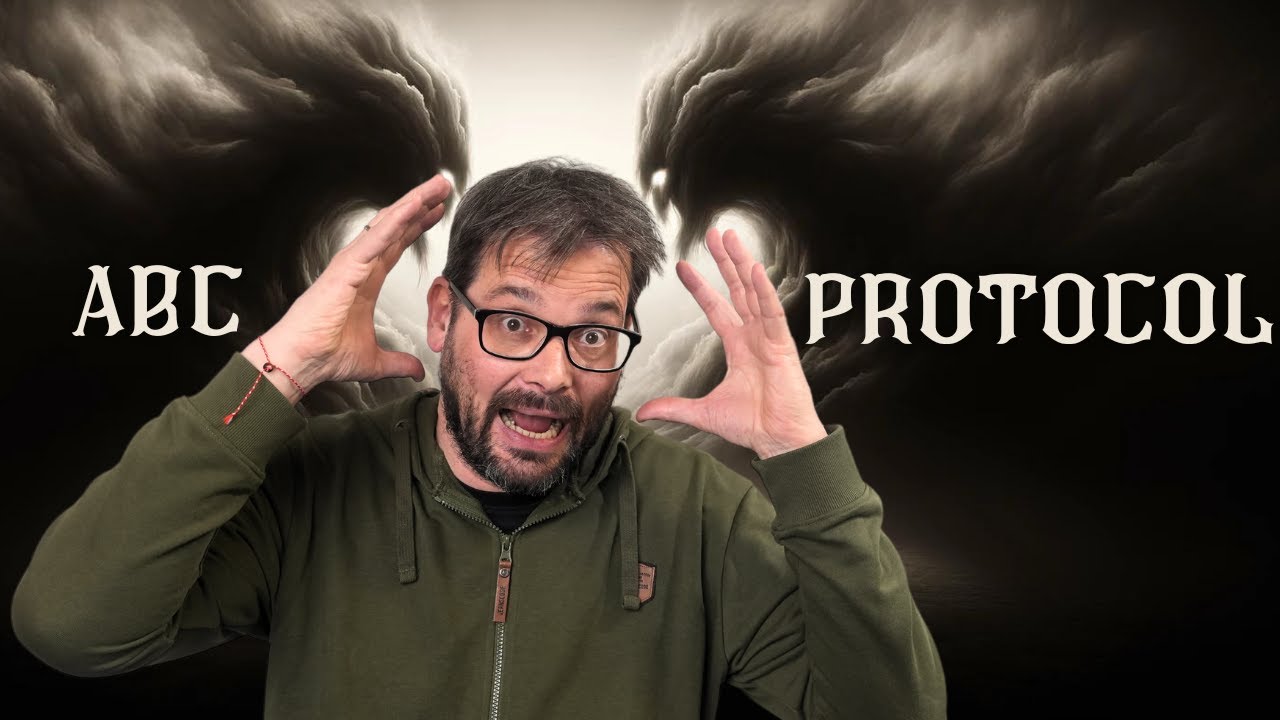
Показать описание
In this video, I’m revisiting Protocols and ABCs in Python, essential for creating abstraction layers by defining interfaces. I covered this a while back, but it deserves a fresh look to clarify: what are the key differences, and when should you use each?
🔖 Chapters:
0:00 Intro
1:03 Short overview
1:53 Abstract Base Classes
5:39 Protocols
9:21 Making Protocols Behave Like ABCs
12:45 Conclusion
14:54 Outro
#arjancodes #softwaredesign #python
Protocols vs ABCs in Python - When to Use Which One?
Protocols vs. ABCs in Python - What’s the Difference?
Protocol Or ABC In Python - When to Use Which One?
What are 'Protocols' In Python? (Tutorial 2023)
Python Interfaces and Abstract Base Class (ABC): A Must-Know for Advanced Programmers
Protocols vs ABCs, Which One Should You Use?
ABC или Protocol в Python? Что лучше и когда стоит использовать?
protocols vs abcs in python when to use which one
You NEED to know about Python protocols
How To Avoid Coupling In Python: ABCs, Protocols, Types
Python's ABC (Abstract Base Class) in 2 Minutes
python typing protocol vs abc
What’s a Protocol Class in Python?
Protocols in Python: Why You Need Them - presented by Rogier van der Geer
protocol vs abstract class in python
structural subtyping in python with Protocol! (intermediate) anthony explains #164
Python abstract base class (ABC) example
The Python ABC's.. Abstract Base Class! #python #programming #coding
Python abstract classes 👻
Python's collections.abc | InvertibleDict
Metaclasses in Python
🤔 The Real Problem with Inheritance
ALPHABET LORE A-Z but everyone crying - ALPHABET LORE ANIMATION MEME - abcdefghijklmnopqrstuvwxyz
When you Over Optimize a Python Function
Комментарии