filmov
tv
Fixing the NameError in Your Python String Encryption Program
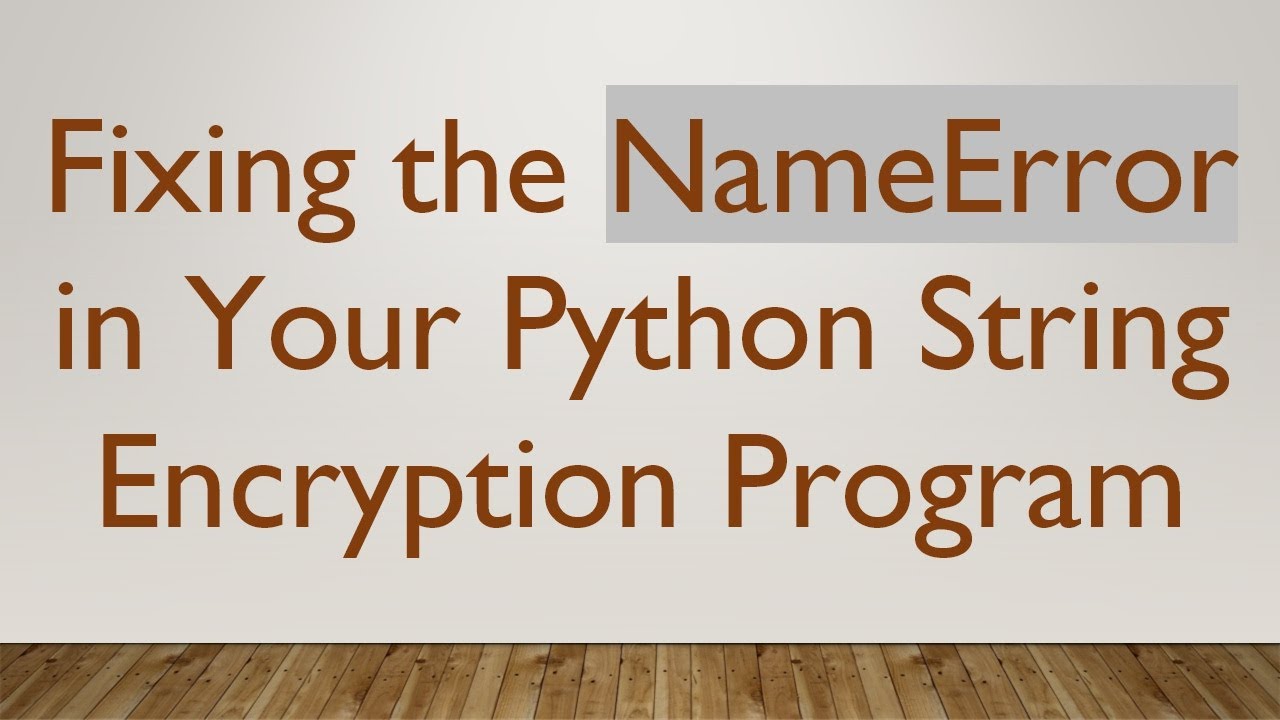
Показать описание
Discover how to resolve the common `NameError` in Python by properly defining variables within your encryption program. Learn to improve your code with user input for better flexibility.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Why are these errors showing up?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting Python Errors: Fixing the NameError in Your String Encryption Program
When programming in Python, encountering errors like NameError can be frustrating, especially when you're working on an interesting project, such as encrypting a string into cipher text. If you’ve come across the error message stating "name 'plaintext' is not defined," this guide will guide you through understanding the problem, why it occurs, and what steps you can take to fix it.
Understanding the Issue
The crux of the problem arises in the following line of code:
[[See Video to Reveal this Text or Code Snippet]]
In this line, the variable plaintext is used, but it hasn’t been defined anywhere in your code. Hence, Python doesn’t know what plaintext refers to, throwing a NameError.
Solution to the NameError
To resolve this issue, you have a couple of options to consider. Here's a step-by-step breakdown of how you can fix it.
Option 1: Define the Variable in the main Function
The most straightforward way to fix this error is to define the plaintext variable directly within the main function:
[[See Video to Reveal this Text or Code Snippet]]
In this option, plaintext is assigned a default string value when you call main().
Option 2: Pass plaintext as a Parameter
Another way to resolve the issue is to modify the main function to accept plaintext as an argument:
[[See Video to Reveal this Text or Code Snippet]]
This option allows you to initialize plaintext when you call the function, making your program more flexible.
Enhancing User Interaction
If you'd like to improve your program further, consider allowing users to input text that they want to encrypt. Instead of using a hard-coded value, you can replace the string in main with Python's input() function:
[[See Video to Reveal this Text or Code Snippet]]
This will prompt users to enter any text they wish to encrypt whenever they run your program, enhancing interactivity and usability.
Conclusion
Understanding and fixing errors like NameError is crucial for your growth as a programmer. By defining variables properly and incorporating user input, you can create more dynamic and functional programs. Now that you know how to fix this particular misunderstanding, don't hesitate to implement these changes and explore further enhancements to your encryption program!
Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Why are these errors showing up?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting Python Errors: Fixing the NameError in Your String Encryption Program
When programming in Python, encountering errors like NameError can be frustrating, especially when you're working on an interesting project, such as encrypting a string into cipher text. If you’ve come across the error message stating "name 'plaintext' is not defined," this guide will guide you through understanding the problem, why it occurs, and what steps you can take to fix it.
Understanding the Issue
The crux of the problem arises in the following line of code:
[[See Video to Reveal this Text or Code Snippet]]
In this line, the variable plaintext is used, but it hasn’t been defined anywhere in your code. Hence, Python doesn’t know what plaintext refers to, throwing a NameError.
Solution to the NameError
To resolve this issue, you have a couple of options to consider. Here's a step-by-step breakdown of how you can fix it.
Option 1: Define the Variable in the main Function
The most straightforward way to fix this error is to define the plaintext variable directly within the main function:
[[See Video to Reveal this Text or Code Snippet]]
In this option, plaintext is assigned a default string value when you call main().
Option 2: Pass plaintext as a Parameter
Another way to resolve the issue is to modify the main function to accept plaintext as an argument:
[[See Video to Reveal this Text or Code Snippet]]
This option allows you to initialize plaintext when you call the function, making your program more flexible.
Enhancing User Interaction
If you'd like to improve your program further, consider allowing users to input text that they want to encrypt. Instead of using a hard-coded value, you can replace the string in main with Python's input() function:
[[See Video to Reveal this Text or Code Snippet]]
This will prompt users to enter any text they wish to encrypt whenever they run your program, enhancing interactivity and usability.
Conclusion
Understanding and fixing errors like NameError is crucial for your growth as a programmer. By defining variables properly and incorporating user input, you can create more dynamic and functional programs. Now that you know how to fix this particular misunderstanding, don't hesitate to implement these changes and explore further enhancements to your encryption program!
Happy coding!