filmov
tv
Find Closest Number to Zero - Leetcode 2239 - Arrays & Strings (Python)
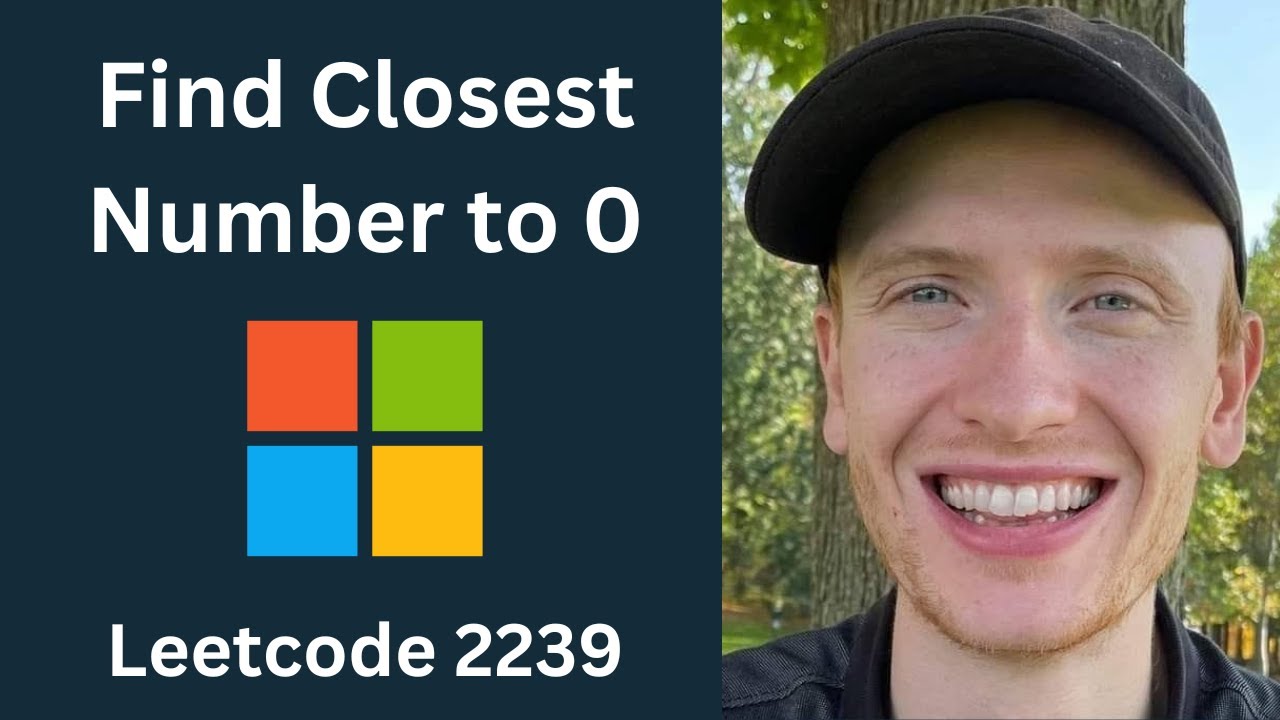
Показать описание
Please check my playlists for free DSA problem solutions:
My Favorite Courses:
Data Structures & Algorithms:
Python:
Web Dev / Full Stack:
Cloud Development:
Game Development:
SQL & Data Science:
Machine Learning & AI:
Find Closest Number to Zero - Leetcode 2239 - Arrays & Strings (Python)
2239. Find Closest Number to Zero | LEETCODE EASY | ARRAY
[SOLVED!] Find Closest Number to Zero - LeetCode #2239 - Java
2239. Find Closest Number to Zero || Leetcode BiWeekly Contest 76 || Leetcode 2239
2239. Find Closest Number to Zero (Leetcode Easy)
2239. Find Closest Number to Zero
Leetcode Biweekly contest 76 -Find Closest Number to Zero
Find Closest Number to Zero - How to Think and Solve || Leetcode - 2239 || Python Solution || FAANG
Barbie Tri-Zero Read to Print (almost)
leetcode_dart: 2239. Find Closest Number to Zero
2239. Find Closest Number to Zero || solution
Leetcode 2239 - Find Closest Number to Zero
LeetCode 2239 | Find Closest Number to Zero | Java
2239.Find Closest Number to Zero LeetCode solution #leetcode
Find Closest Number to Zero - LeetCode 2239 - Java
Find the Closest Number to ZERO in an array | Leetcode #2239 #shorts
Finding Number Close To Zero || Java Interview Question Explained in Hindi
Array-LeetCode 2239-Find Closest Number to Zero
Find Closest Number to Zero | Leetcode 2239 | Amazon Google Facebook interview question
5 K Closest Numbers
leetcode 2239 Find Closest Number to Zero
Find The Two Array Elements Whose Sum Is Closest To Zero | C Programming Example
Two Elements Whose Sum Is Closest To Zero in Java Video 6
Sum of two elements with sum nearest to zero || GeeksforGeeks || Problem of the Day || Must Watch
Комментарии