filmov
tv
[Java] Leetcode 283. Move Zeroes [Two Pointers #2]
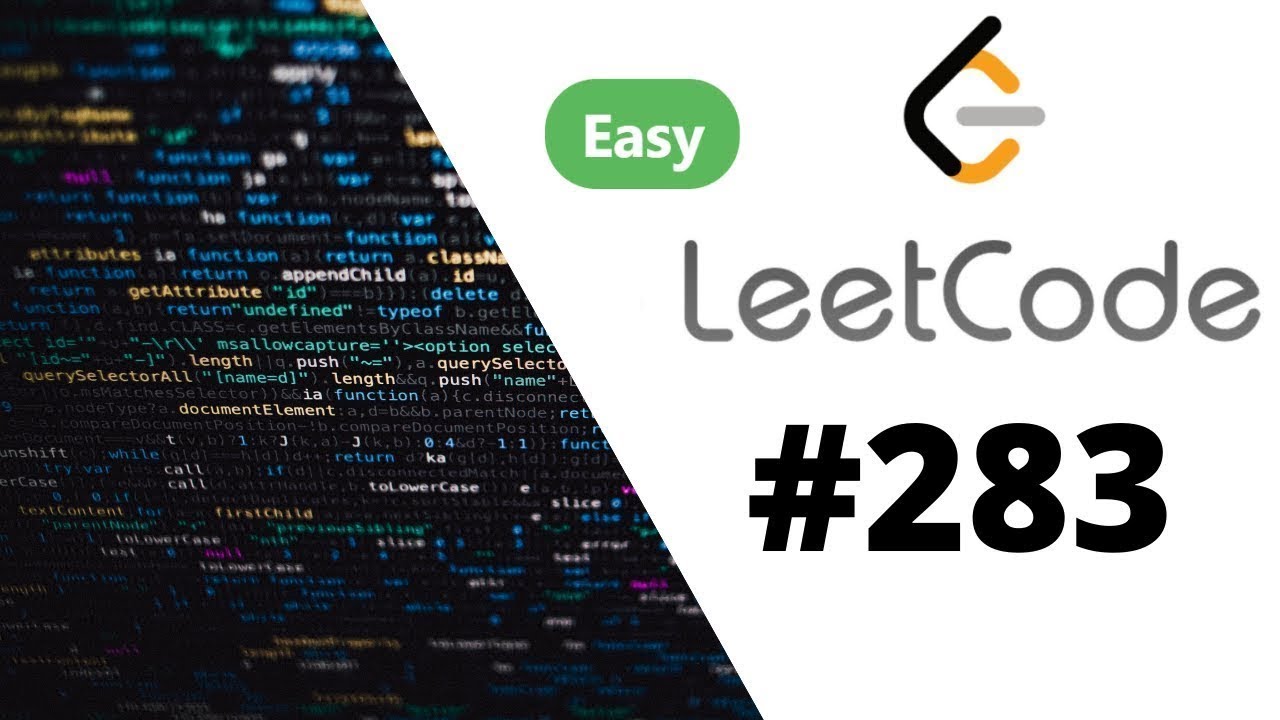
Показать описание
In this video, I'm going to show you how to solve Leetcode 283. Move Zeroes which is related to Two Pointers.
In the end, you’ll have a really good understanding on how to solve Leetcode 283. Move Zeroes and questions that are similar to this Two Pointers.
In the end, you’ll have a really good understanding on how to solve Leetcode 283. Move Zeroes and questions that are similar to this Two Pointers.
[Java] Leetcode 283. Move Zeroes [Two Pointers #2]
Move Zeros | LeetCode problem 283
Leetcode | 283. Move Zeroes | Easy | Java Solution
LeetCode 283 | Move Zeroes | Solution Explained (Java + Whiteboard)
Move Zeroes (LeetCode 283) | Full Solution explained with examples | Study Algorithms
Move Zeroes | Leetcode 283
Move Zeroes - Leetcode 283 - Python
LeetCode Problem: 283. Move Zeroes | Java Solution
LeetCode - 283. Move Zeroes | Two Pointers Technique | Java
LeetCode 283: Move Zeroes - Interview Prep Ep 53
Leetcode 283. Move Zeroes [Java]
Move Zeroes
Собеседование в IT | LeetCode | 283. Move Zeroes
Move Zeroes - LeetCode 283 - Two Pointers - Coding Interview Questions
Move zeroes LeetCode Java solution in Hindi ( LeetCode 283 )
LeetCode Problem: 283. Move Zeroes || Java Solution Walkthrough
LeetCode : 283. Move Zeroes | Java
Leetcode 283 | Move zeroes | 10th from LC 75 | 90 days SE prep
Move Zeroes | Leetcode 283 | in-place algorithm | Java
283. Move Zeroes | Leetcode | DSA | Java
LeetCode Problem 283. Move Zeroes Short
283. Move Zeroes || In Java || Easy || LeetCode Problem #leetcode
Разбор задачи 283 leetcode.com Move Zeroes. Решение на C++
Move zeroes | Leetcode
Комментарии