filmov
tv
Move zeroes | Leetcode
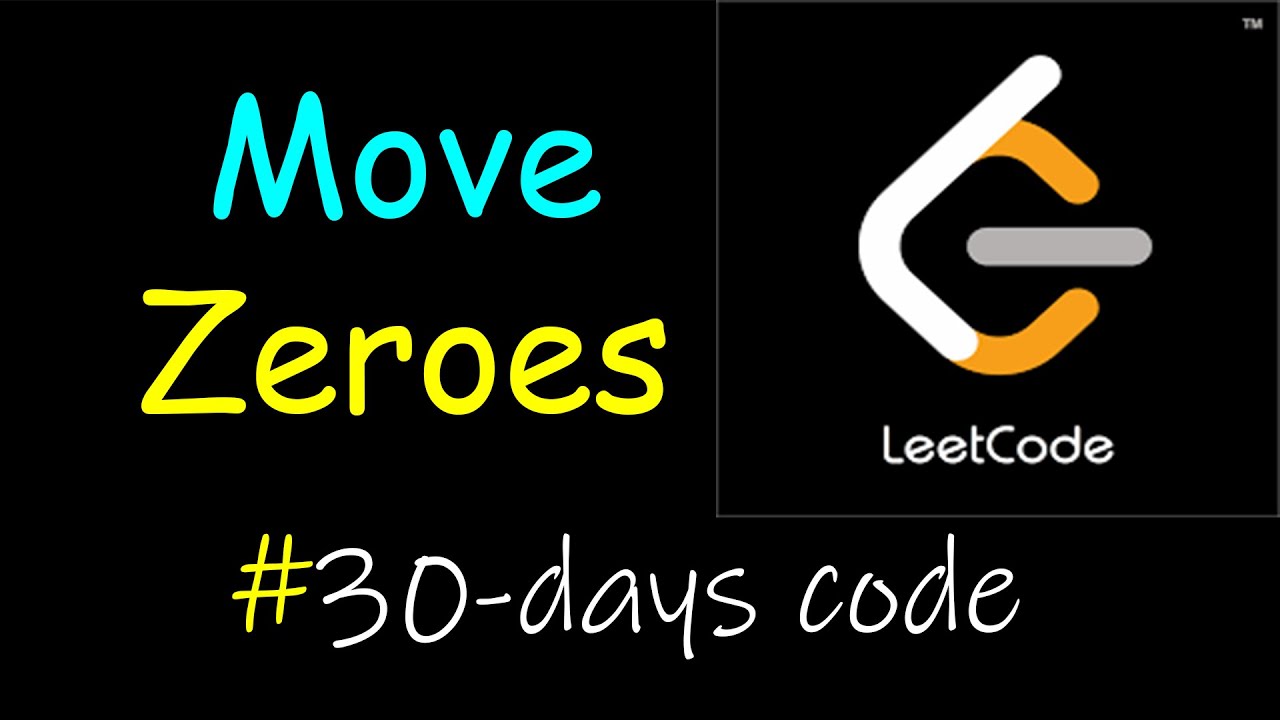
Показать описание
This video explains the day-4 problem of leetcode 30 days coding challenge in april 2020. I have explained the problem with given constraints in mind using example and code. This video first explains the simple approach and then an intuitive approach of solving the problem using two pointer technique. It solves the problem in O(N) time and O(1) extra space with inplace algorithm. As usual, CODE LINK is given below. If you find any difficulty or have any query then do COMMENT below. PLEASE help our channel by SUBSCRIBING and LIKE our video if you found it helpful...CYA :)
Move Zeroes - Leetcode 283 - Python
Move Zeroes (LeetCode 283) | Full Solution explained with examples | Study Algorithms
Move Zeros | LeetCode problem 283
Move Zeroes | Leetcode 283
Move Zeroes - LeetCode 283 - Two Pointers - Coding Interview Questions
MOVE ZEROES | LEETCODE 283 | PYTHON SOLUTION
Move zeroes | Leetcode
LeetCode 283 | Move Zeroes | Solution Explained (Java + Whiteboard)
Leetcode | 283. Move Zeroes | Easy | Java Solution
[Java] Leetcode 283. Move Zeroes [Two Pointers #2]
Solving LeetCode 283 in JavaScript (Move Zeroes)
LeetCode 283: Move Zeroes - Interview Prep Ep 53
Move Zeroes - LeetCode 283 - JavaScript
283. Move Zeroes | LeetCode 75 #10 | Two Pointers
283 Move Zeroes LeetCode (Google Interview Question) JavaScript
Собеседование в IT | LeetCode | 283. Move Zeroes
Move Zeroes - LeetCode 283 - Python #leetcode #leetcode75 #2pointers
Leetcode 283. Move Zeroes
Move Zeroes
Move zeroes LeetCode Java solution in Hindi ( LeetCode 283 )
Python Programming Practice: LeetCode #283 -- Move Zeroes
Rotate Array by K places | Union, Intersection of Sorted Arrays | Move Zeros to End | Arrays Part-2
Move Zeroes | Python | Leetcode - 283
Leetcode 283 | Move zeroes | 10th from LC 75 | 90 days SE prep
Комментарии