filmov
tv
How to control a DC motor with an encoder
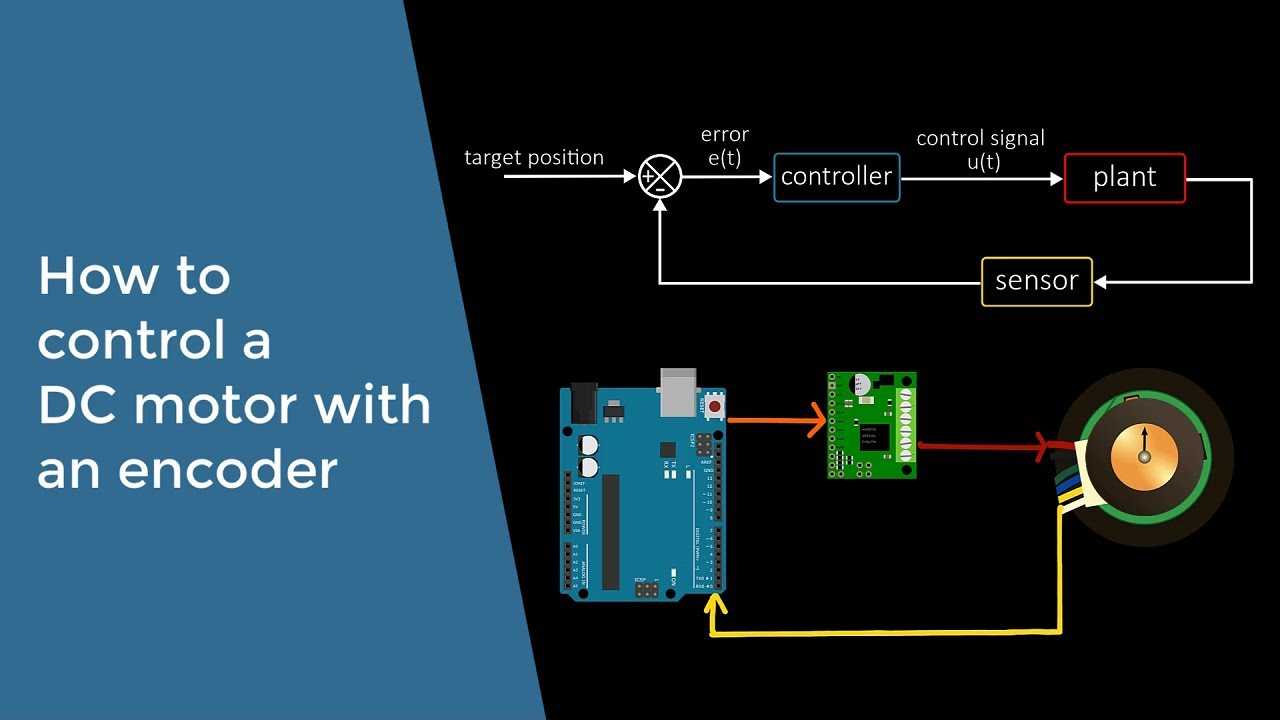
Показать описание
If your platform does not have access to "atomic.h" (and so you get an error message), you can use the alternative version of the code that has been uploaded to the repository. It is labeled "_NoAtomic".
An encoder makes it possible to control the position of a DC motor. In this video, I illustrate how an encoder works, and then use a PID control algorithm to control the motor position. All of the steps are included so that you will be easily able to make the system yourself.
Parts used in this video:
1. DC Motor - 19:1 Metal Gearmotor 37Dx68L mm 12V with 64 CPR Encoder:
2. Motor Driver - TB67H420FTG Dual/Single Motor Driver Carrier:
3. Microcontroller - Arduino Uno:
(okay actually I used an Elegoo Uno, but the Arduino descriptions are better :)
How to control DC & AC motors
How to control a DC motor with an encoder
Control a DC Motor with Arduino (Lesson #16)
How PWM works | Controlling a DC motor with a homemade circuit
PWM DC Motor Speed Control Module 2A (1.8V-12V) | How to motor speed control | POWER-GEN
Arduino DC Motor Control Tutorial - L298N | H-Bridge | PWM | Robot Car
how to make Simple dc motor speed control circuit, electronics projects , banggood
Arduino Tutorial 37: Understanding How to Control DC Motors in Projects
DC motor hacks#shorts
How to Make 3.7v Dc Motor and Fan Speed Controller Circuit
Speed Control - AC and DC Motors
How to control multiple DC motors with encoders
How to control a DC motor with L298N driver and Arduino Uno
L298N | how to control dc motor with Arduino | Motor speed and direction control
Controlling DC Motors with the L298N H Bridge and Arduino
DC motor's speed Controller | Electronics
dc motor | speed control | Amazing Experiment Projects
High power DC motor speed control circuit
DC motor PID speed control
How To Make Simple DC Motor Speed Controller Circuit
Speed Control of DC Motor - DC Motor Speed control
Driving DC Motors with Microcontrollers
DC motor forward reverse #shorts
Raspberry Pi How to Control a DC Motor With an L298N Driver
Комментарии