filmov
tv
DP 27. Longest Common Substring | DP on Strings 🔥
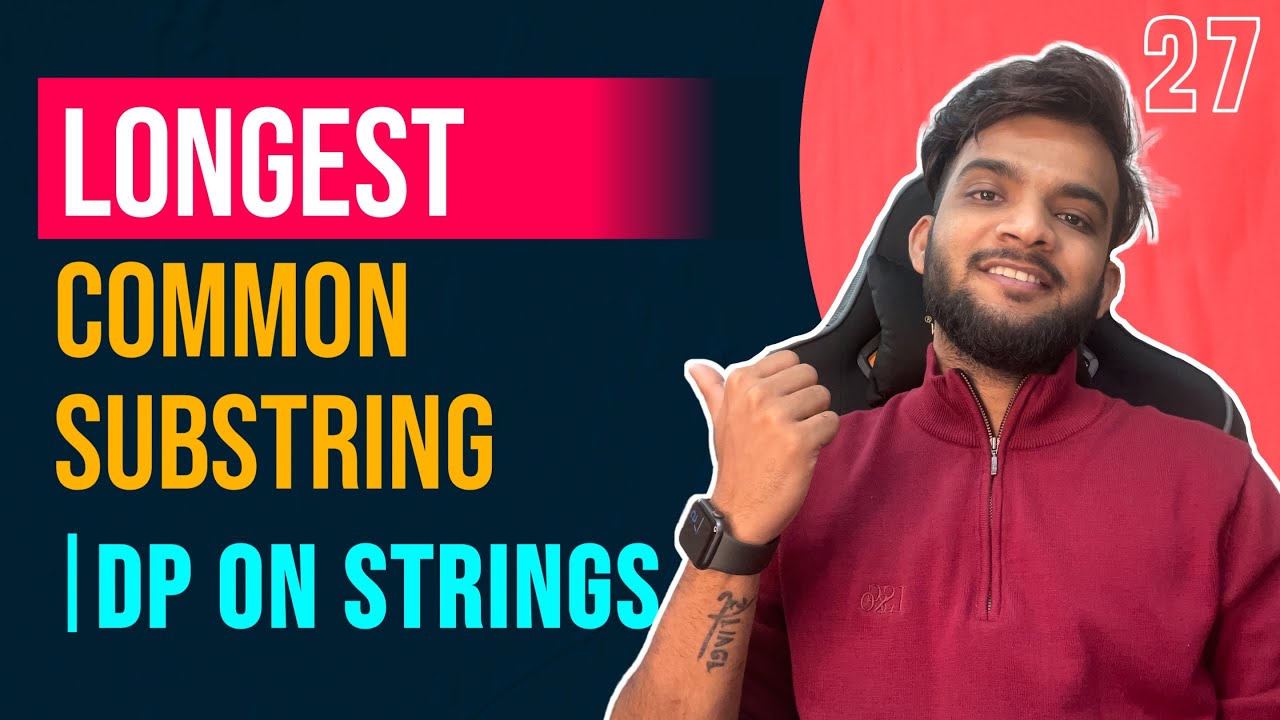
Показать описание
a
In this video, we solve the MCM Dp, this is the first problem on the pattern Partition DP.
DP 27. Longest Common Substring | DP on Strings 🔥
Longest common substring | Dynamic programming
Longest Common Subsequence - Dynamic Programming - Leetcode 1143
Longest Common Substring | Dynamic Programming | Data Structures And Algorithms | Simplilearn
Most Asked FAANG Coding Question! | Longest Common Prefix - Leetcode 14
Longest Common Subsequence | Recursion + Memoisation + Tabulation | DP-27
Longest Common Substring (dynamic programming)
Longest Common Substring PART 2 | Bottom Up DP | Java | Recursion, Dynamic Programming
Longest Common Subsequence - Recursive and Iterative DP (LeetCode Day 26)
Longest common substring #Dynamic Progamming C++ Code
Longest common substring using Dynamic Programming | bottom-up approach
Longest Common Substring | Dynamic Programming | Intuition and explanation w/ animations
Longest Common Substring PART 1 | Memoization | Java | Recursion, Dynamic Programming
Print the longest common substring || Dynamic Programming || Python || Mohit Bodhija
Dynamic Programming Solutions DPV 6.8 Longest Common Substring
Lecture 135: Longest Common Subsequence || DP on Strings
Longest Common Substring - 2D Dynamic Programming - Design and Analysis of Algorithms
5 Simple Steps for Solving Dynamic Programming Problems
Longest Common Substring Dynamic Programming
Longest Common Substring | Recursion + Memoisation + Tabulation | DP-28
Longest Common Substring | DP-29 | Dynamic Programming | Python | Mohit Bodhija
Dp 25. Longest Common Subsequence | Top Down | Bottom-Up | Space Optimised | DP on Strings
Longest Common Substring | DP | Love Babbar DSA Sheet | GFG | Amazon🔥
Longest Common Substring | Recursion and Memoization
Комментарии