filmov
tv
Understanding the append() Function: Why list(x) Makes a Difference
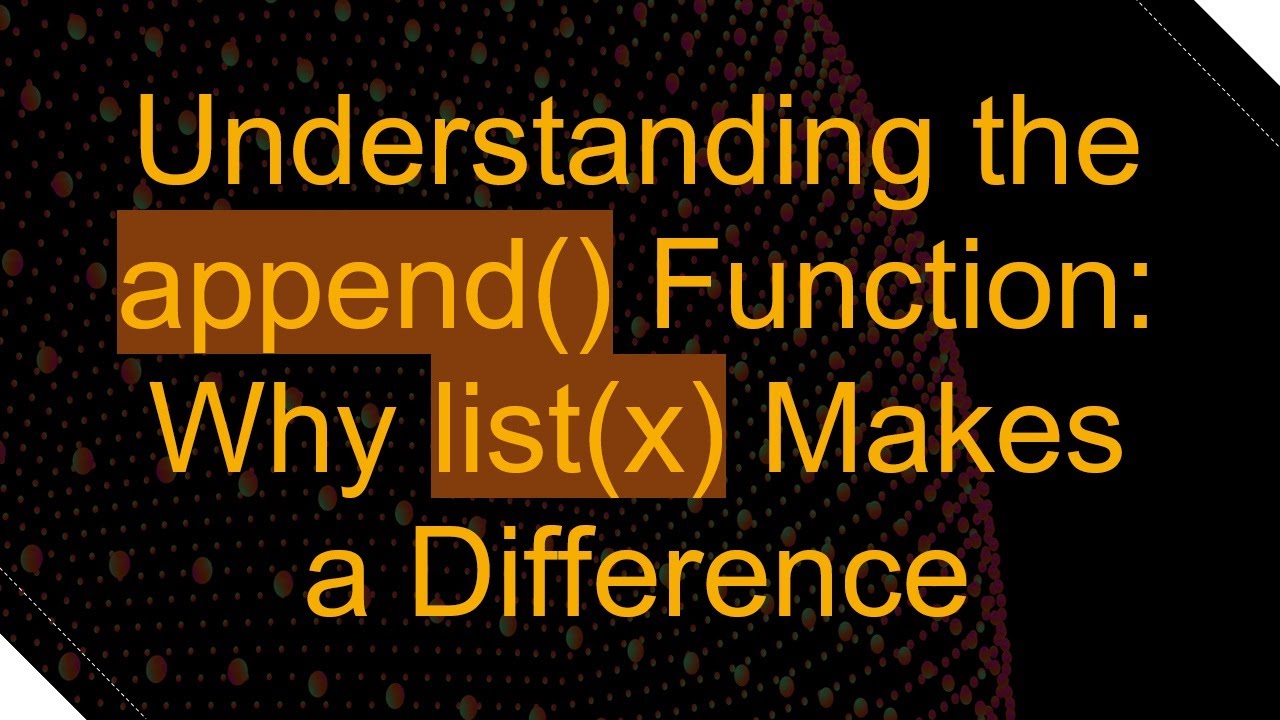
Показать описание
Discover why using `list(x)` instead of `x` in the `append()` method changes the behavior of your Python code—leading to unexpected results.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Unexpected behaviour of append
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the append() Function: Why list(x) Makes a Difference
When coding in Python, it's not uncommon to encounter unexpected behavior, especially when working with lists. A common issue arises when using the append() method in conjunction with variable references. In this guide, we will dive into a specific programming conundrum involving list references and how making seemingly minor changes can lead to drastically different outputs.
The Problem at Hand
Consider the following Python code snippet, which is designed to generate all combinations of characters for a given string that contains placeholders:
[[See Video to Reveal this Text or Code Snippet]]
The Unexpected Outputs
When you run this piece of code, you end up with the following output:
[[See Video to Reveal this Text or Code Snippet]]
On the surface, this appears to be incorrect, given the intent of generating all combinations. The problem stems from how the list x is being passed and modified within the append() method. Let's explore why this happens.
Understanding List References
In the code, the critical line causing confusion is:
[[See Video to Reveal this Text or Code Snippet]]
When you append x to ans, you're not copying the values of x; rather, you're referencing x directly. This means that if you subsequently modify x, all references in ans reflect those changes. This leads to unexpected results because all appended lists reference the same original list.
Example to Illustrate
To further clarify, let's look at a simpler example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, when we modify a, the contents of b also update, leading to unexpected values being printed. This highlights the fact that both a and b[0] reference the same list.
The Solution: Using list(x)
The correct way to preserve the state of x at the moment of appending is to create a copy of x by using the list(x) function. Let's modify the earlier code accordingly:
[[See Video to Reveal this Text or Code Snippet]]
Output Explanation
When the above code is executed, the output becomes:
[[See Video to Reveal this Text or Code Snippet]]
This output now accurately reflects all combinations of the string ??, as each instance of list(x) represents a snapshot of the list x at the moment of appending.
Conclusion
In summary, when working with lists in Python, it's crucial to understand the distinction between appending a reference of a list and appending a copy. Using list(x) ensures that you maintain the intended state of your list at the time it is appended to another list, preserving the expected outcomes. Always remember: modifying a referenced list will affect all references pointing to it, while appending a copy will only affect the original.
By grasping these fundamental concepts, you enhance your Python coding skills and prevent confusing bugs in your applications.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Unexpected behaviour of append
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the append() Function: Why list(x) Makes a Difference
When coding in Python, it's not uncommon to encounter unexpected behavior, especially when working with lists. A common issue arises when using the append() method in conjunction with variable references. In this guide, we will dive into a specific programming conundrum involving list references and how making seemingly minor changes can lead to drastically different outputs.
The Problem at Hand
Consider the following Python code snippet, which is designed to generate all combinations of characters for a given string that contains placeholders:
[[See Video to Reveal this Text or Code Snippet]]
The Unexpected Outputs
When you run this piece of code, you end up with the following output:
[[See Video to Reveal this Text or Code Snippet]]
On the surface, this appears to be incorrect, given the intent of generating all combinations. The problem stems from how the list x is being passed and modified within the append() method. Let's explore why this happens.
Understanding List References
In the code, the critical line causing confusion is:
[[See Video to Reveal this Text or Code Snippet]]
When you append x to ans, you're not copying the values of x; rather, you're referencing x directly. This means that if you subsequently modify x, all references in ans reflect those changes. This leads to unexpected results because all appended lists reference the same original list.
Example to Illustrate
To further clarify, let's look at a simpler example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, when we modify a, the contents of b also update, leading to unexpected values being printed. This highlights the fact that both a and b[0] reference the same list.
The Solution: Using list(x)
The correct way to preserve the state of x at the moment of appending is to create a copy of x by using the list(x) function. Let's modify the earlier code accordingly:
[[See Video to Reveal this Text or Code Snippet]]
Output Explanation
When the above code is executed, the output becomes:
[[See Video to Reveal this Text or Code Snippet]]
This output now accurately reflects all combinations of the string ??, as each instance of list(x) represents a snapshot of the list x at the moment of appending.
Conclusion
In summary, when working with lists in Python, it's crucial to understand the distinction between appending a reference of a list and appending a copy. Using list(x) ensures that you maintain the intended state of your list at the time it is appended to another list, preserving the expected outcomes. Always remember: modifying a referenced list will affect all references pointing to it, while appending a copy will only affect the original.
By grasping these fundamental concepts, you enhance your Python coding skills and prevent confusing bugs in your applications.