filmov
tv
Understanding Python Lists in Functions: Tips for Using append vs extend
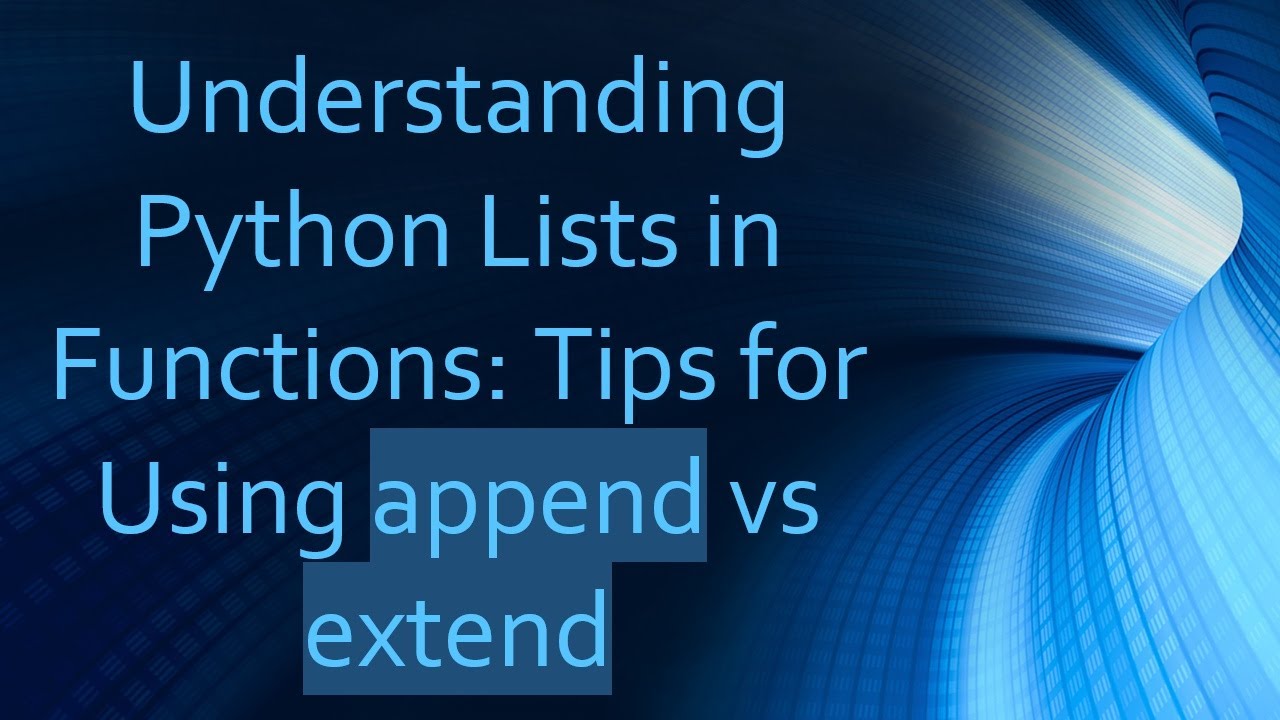
Показать описание
Learn how to effectively use lists in Python functions and understand the difference between `append` and `extend`. Get ready to optimize your code for better results!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: list in def function
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Python Lists in Functions: Tips for Using append vs extend
When you are coding in Python, dealing with lists can be both powerful and sometimes confusing, especially when you're using functions. If you've tried to use lists in function definitions and found unexpected results, this guide is for you. We'll explore the common issue faced when appending elements to lists in functions and the solution to enhance your code.
The Problem
Let's take a closer look at a typical scenario involving a function and lists. Consider the following code sample:
[[See Video to Reveal this Text or Code Snippet]]
Expected Output
You might expect that the output would simply be ['note']. However, the actual output you receive will be somewhat unexpected, leading to confusion:
[[See Video to Reveal this Text or Code Snippet]]
What’s happening here?
The Breakdown
Understanding append:
The append method in Python adds a single element to the end of the list.
This creates a nested list structure: [['note']].
Why It Matters:
This behavior can be detrimental if you're expecting a flat list containing only the items you want. Knowing how to manipulate these structures effectively is key in writing cleaner and more functional Python code.
The Solution: Using extend Instead of append
To achieve the expected output, you would instead use the extend method, which takes each element in the iterable (like a list) and adds them to the list individually.
How to Implement the Solution
Simply replace the append call with extend in the main function:
[[See Video to Reveal this Text or Code Snippet]]
Revised Expected Output
With this change, when you run the code, you'll now see:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding the differences between append and extend in Python is fundamental when working with lists, especially within functions.
Use append when you want to add a single item to a list.
Use extend when you want to combine another iterable (like another list) into your current list.
By using these methods correctly, you can create more predictable and functional code in Python. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: list in def function
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Python Lists in Functions: Tips for Using append vs extend
When you are coding in Python, dealing with lists can be both powerful and sometimes confusing, especially when you're using functions. If you've tried to use lists in function definitions and found unexpected results, this guide is for you. We'll explore the common issue faced when appending elements to lists in functions and the solution to enhance your code.
The Problem
Let's take a closer look at a typical scenario involving a function and lists. Consider the following code sample:
[[See Video to Reveal this Text or Code Snippet]]
Expected Output
You might expect that the output would simply be ['note']. However, the actual output you receive will be somewhat unexpected, leading to confusion:
[[See Video to Reveal this Text or Code Snippet]]
What’s happening here?
The Breakdown
Understanding append:
The append method in Python adds a single element to the end of the list.
This creates a nested list structure: [['note']].
Why It Matters:
This behavior can be detrimental if you're expecting a flat list containing only the items you want. Knowing how to manipulate these structures effectively is key in writing cleaner and more functional Python code.
The Solution: Using extend Instead of append
To achieve the expected output, you would instead use the extend method, which takes each element in the iterable (like a list) and adds them to the list individually.
How to Implement the Solution
Simply replace the append call with extend in the main function:
[[See Video to Reveal this Text or Code Snippet]]
Revised Expected Output
With this change, when you run the code, you'll now see:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding the differences between append and extend in Python is fundamental when working with lists, especially within functions.
Use append when you want to add a single item to a list.
Use extend when you want to combine another iterable (like another list) into your current list.
By using these methods correctly, you can create more predictable and functional code in Python. Happy coding!