filmov
tv
Introduction to Functions in Arduino: Defining and Calling Functions
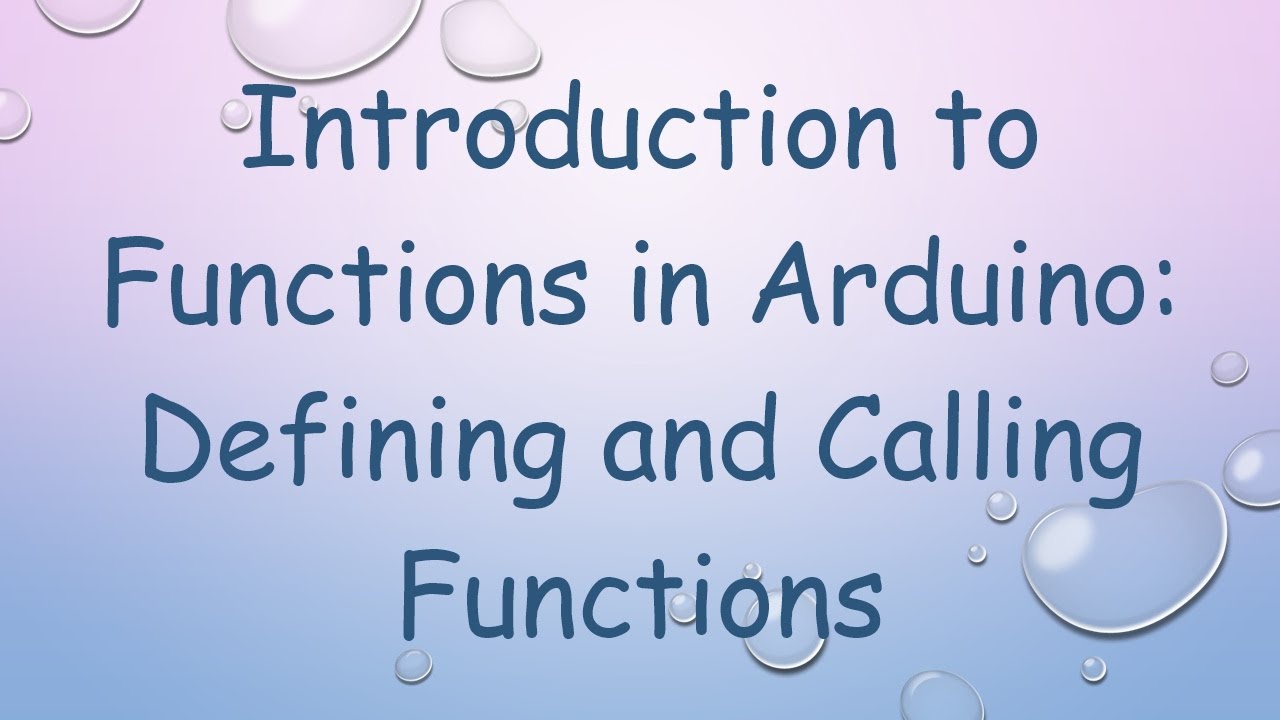
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to define and call functions in Arduino sketches to organize your code and improve readability and maintainability of your projects. This guide covers the basics of creating functions in Arduino code, including syntax and best practices.
---
Arduino programming involves writing code to control microcontroller-based projects. As projects grow in complexity, organizing code becomes crucial for readability and maintainability. One way to achieve this is by using functions. Functions in Arduino serve as blocks of code that can be called upon to perform specific tasks, making the code more modular and easier to manage.
Defining Functions
To define a function in Arduino, you need to specify its return type, name, and parameters (if any). Here's the basic syntax:
[[See Video to Reveal this Text or Code Snippet]]
return_type: Specifies the type of value that the function returns, such as void for functions that don't return anything, or data types like int, float, char, etc.
function_name: The name of the function, which you use to call it from other parts of your code.
parameters: Optional input values that the function can accept. These are like placeholders that store values passed to the function when it's called.
Let's look at an example of a simple function that prints "Hello, World!" to the serial monitor:
[[See Video to Reveal this Text or Code Snippet]]
In this example:
void is the return type, indicating that the function doesn't return any value.
printHello is the name of the function.
The function doesn't accept any parameters.
Calling Functions
Once you've defined a function, you can call it from anywhere within your sketch. To call a function, simply use its name followed by parentheses ():
[[See Video to Reveal this Text or Code Snippet]]
In this example, printHello() is called within the setup() function. This means that when the Arduino board starts up, it will print "Hello, World!" to the serial monitor.
Benefits of Using Functions
Using functions offers several advantages:
Modularity: Functions allow you to break down your code into smaller, reusable chunks, making it easier to understand and maintain.
Readability: By giving meaningful names to your functions, you can make your code self-documenting and easier to comprehend.
Code Reusability: Once you've written a function, you can call it multiple times throughout your sketch or in different sketches altogether.
Debugging: Functions help isolate specific parts of your code, making it easier to identify and fix errors.
Conclusion
Functions are a fundamental concept in Arduino programming. By defining and calling functions, you can organize your code more effectively, leading to better readability and maintainability. Whether you're a beginner or an experienced Arduino enthusiast, mastering functions will greatly enhance your ability to create complex projects.
Happy coding!
---
Summary: Learn how to define and call functions in Arduino sketches to organize your code and improve readability and maintainability of your projects. This guide covers the basics of creating functions in Arduino code, including syntax and best practices.
---
Arduino programming involves writing code to control microcontroller-based projects. As projects grow in complexity, organizing code becomes crucial for readability and maintainability. One way to achieve this is by using functions. Functions in Arduino serve as blocks of code that can be called upon to perform specific tasks, making the code more modular and easier to manage.
Defining Functions
To define a function in Arduino, you need to specify its return type, name, and parameters (if any). Here's the basic syntax:
[[See Video to Reveal this Text or Code Snippet]]
return_type: Specifies the type of value that the function returns, such as void for functions that don't return anything, or data types like int, float, char, etc.
function_name: The name of the function, which you use to call it from other parts of your code.
parameters: Optional input values that the function can accept. These are like placeholders that store values passed to the function when it's called.
Let's look at an example of a simple function that prints "Hello, World!" to the serial monitor:
[[See Video to Reveal this Text or Code Snippet]]
In this example:
void is the return type, indicating that the function doesn't return any value.
printHello is the name of the function.
The function doesn't accept any parameters.
Calling Functions
Once you've defined a function, you can call it from anywhere within your sketch. To call a function, simply use its name followed by parentheses ():
[[See Video to Reveal this Text or Code Snippet]]
In this example, printHello() is called within the setup() function. This means that when the Arduino board starts up, it will print "Hello, World!" to the serial monitor.
Benefits of Using Functions
Using functions offers several advantages:
Modularity: Functions allow you to break down your code into smaller, reusable chunks, making it easier to understand and maintain.
Readability: By giving meaningful names to your functions, you can make your code self-documenting and easier to comprehend.
Code Reusability: Once you've written a function, you can call it multiple times throughout your sketch or in different sketches altogether.
Debugging: Functions help isolate specific parts of your code, making it easier to identify and fix errors.
Conclusion
Functions are a fundamental concept in Arduino programming. By defining and calling functions, you can organize your code more effectively, leading to better readability and maintainability. Whether you're a beginner or an experienced Arduino enthusiast, mastering functions will greatly enhance your ability to create complex projects.
Happy coding!