filmov
tv
Python Tutorial: Write a simple unit test using pytest
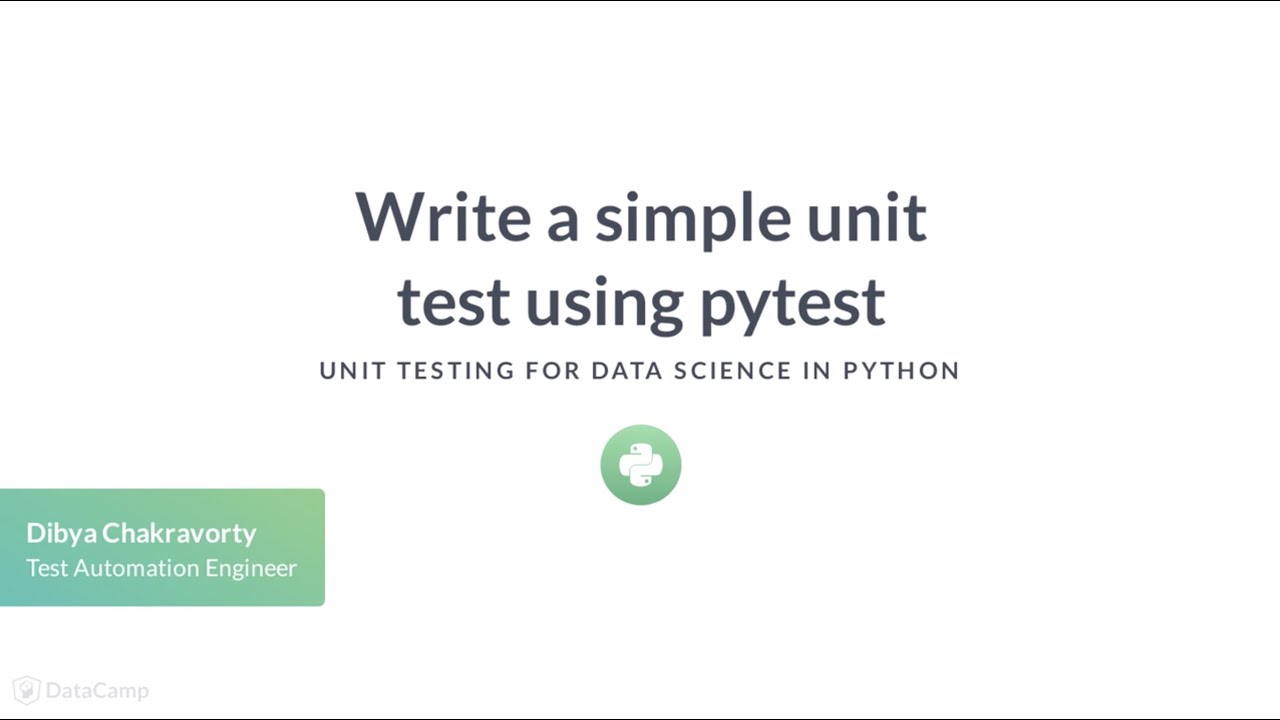
Показать описание
---
In the last exercise, you went through a function's life cycle.
At every step, you tested it by calling row_to_list() on different arguments and checked if the return values are correct. This was repetitive, tedious and time consuming. In this lesson, we will learn to write unit tests and improve this process.
There are many Python libraries for writing unit tests such as pytest, unittest, nosetests, and doctest. We will use pytest for this course, because pytest has all essential features, is easiest to use, and is the most popular testing library in Python.
When pytest sees a filename starting with "test_", it understands that this is not an usual Python file, but a special one containing unit tests. We must make sure to follow this naming convention.
Files holding unit tests are also called test modules, and we just created our first test module.
In the test module, we first import pytest. Then we import the function under test.
A unit test is written as a Python function, whose name starts with a "test_", just like the test module. This way, pytest can tell that it is a unit test and not an ordinary function.
The unit test usually corresponds to exactly one entry in the argument and return value table for row_to_list(). The unit test checks whether row_to_list() has the expected return value when called on this particular argument. This particular argument is a clean row, so we call the unit test test_for_clean_row().
The actual check is done via an assert statement, and every test must contain one.
The assert statement has a required first argument, which can be any boolean expression. If the expression is True, the assert statement passes, giving us a blank output. If the expression is False, it raises an AssertionError.
In this case, we want to check if row_to_list() returns the correct list when called on the clean row. The expression we use is row_to_list() called on the argument equal equals the correct list. If the function works, this will evaluate to True and the assert statement will pass. This will make the test pass. If the function has a bug, it will evaluate to False, the assert statement will raise an AssertionError, and the test will fail.
For the second row in the table, we create a unit test called test_on_missing_area() because the argument has missing area data. Then we assert that the return value for this argument is None.
Note that the correct way to check if a variable's value is None is to use the boolean expression var is None and not var equal equals None.
For the third row in the table, we create a unit test called test_for_missing_tab(), because the argument is missing the tab separating area and price. The assert statement is similar to the second test.
To test whether row_to_list() is working at any time in its life cycle, we simply run the test module.
The standard way to run tests is to open a command line and type pytest followed by the test module name.
In the next step or next exercise, we will write the tests to a test module in the background and tell you its file path. This is highlighted in this slide.
Once you know the test module's file path, you can run the tests in the IPython console at the bottom, which is highlighted here.
You can run any command line expression in the IPython console by adding an exclamation mark before the expression. For example, to run the pytest command, you have to use exclamation pytest, as shown in the picture.
Running this command will output the test result report, which contains information about bugs in the function, if any. We will cover the test result report in the next video lesson.
But now, let's practice writing some simple unit tests using pytest.
#Python #PythonTutorial #DataCamp #Unit #Testing #DataScience #pytest