filmov
tv
How to Dynamically Add Multiple Text Fields in Flutter with a Button Click
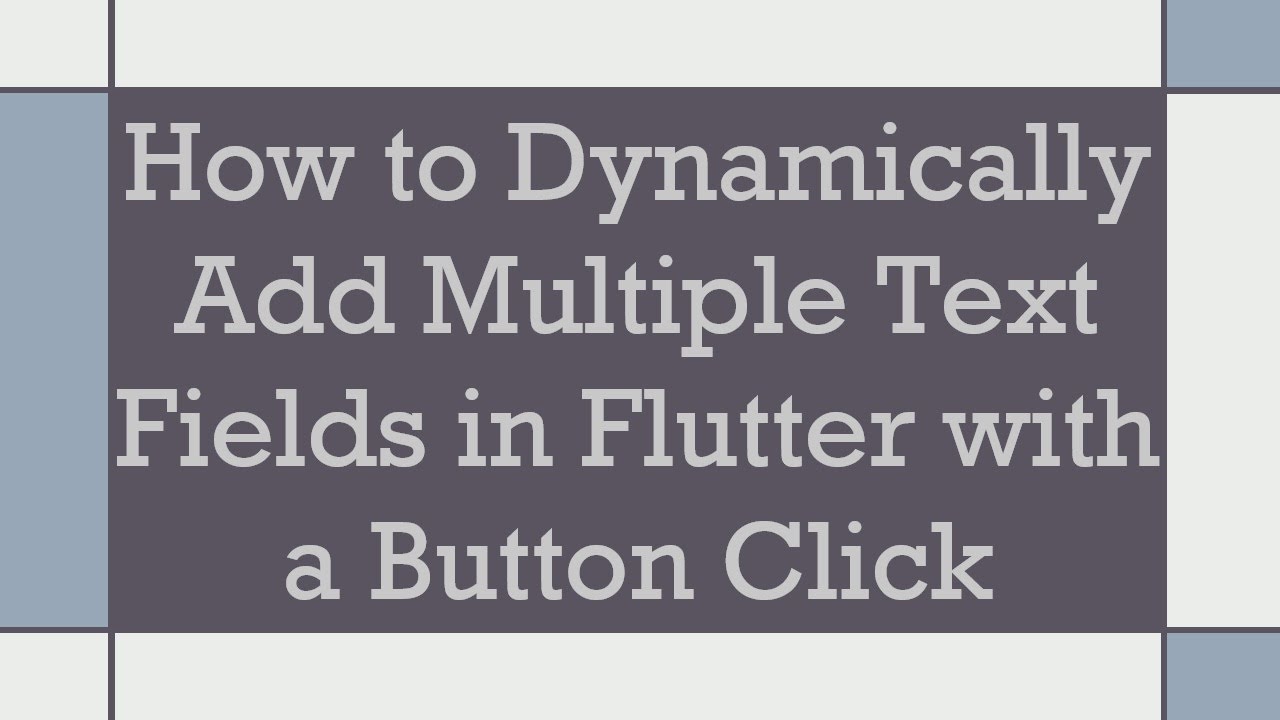
Показать описание
Learn how to add dynamic text fields to your Flutter app. This guide explains how to create a simple interface where users can add text fields by pressing a button!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: create more on same page on clicked button
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Dynamically Add Multiple Text Fields in Flutter with a Button Click
Creating interactive interfaces is a vital part of app development. One common requirement in applications is to add text fields dynamically based on user interaction, such as pressing a button. In this guide, we’ll address a specific use case: how to start with one text field and allow users to add more fields every time they click a button in Flutter.
Problem Statement
You have a Flutter application and want to display a text input field on the main page. Every time a user presses a button, a new text input field should be created dynamically. You'd also like to capture all the values from these text fields so you can pass them to another page when needed.
Solution Overview
To achieve this functionality, we will use a StatefulWidget, which allows you to manage the state of your application. Here's a breakdown of the steps to accomplish this:
Step 1: Create a StatefulWidget
First, make sure that the widget holding the text fields is a StatefulWidget. This is important because we need to manage the number of text fields dynamically.
Step 2: Track the Number of Text Fields
Declare an int variable to keep track of how many text fields are currently displayed. We will start by initializing this variable to 1, as we want to display one text field initially.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Render Text Fields Dynamically
Within the build method of your widget, you will use a for loop to render text fields based on the count stored in numberOfTextFields. Here's how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Increment the Count with a Button Click
Now we need to link our button to an action that increments the count of text fields. This will be done inside the callback method for your button. You will call setState() to notify Flutter that the state has changed and re-render the UI.
[[See Video to Reveal this Text or Code Snippet]]
Step 5: Capturing Values from the Text Fields
To get the values of the text fields, you’ll need to create controllers for each TextField. When navigating to another page, you can gather these values and pass them as arguments. Here's a simple way to do it:
Create a List of TextEditingController to manage text fields:
[[See Video to Reveal this Text or Code Snippet]]
Update your loop to initialize each text field with a controller:
[[See Video to Reveal this Text or Code Snippet]]
When pressing the button to navigate to another page, use the controllers to gather the values:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following the steps outlined in this guide, you can effectively create a dynamic form in Flutter that allows users to add multiple text fields at runtime. This functionality enhances user engagement and makes your application more versatile.
With just a few lines of code, you can significantly elevate the user experience in your Flutter applications. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: create more on same page on clicked button
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Dynamically Add Multiple Text Fields in Flutter with a Button Click
Creating interactive interfaces is a vital part of app development. One common requirement in applications is to add text fields dynamically based on user interaction, such as pressing a button. In this guide, we’ll address a specific use case: how to start with one text field and allow users to add more fields every time they click a button in Flutter.
Problem Statement
You have a Flutter application and want to display a text input field on the main page. Every time a user presses a button, a new text input field should be created dynamically. You'd also like to capture all the values from these text fields so you can pass them to another page when needed.
Solution Overview
To achieve this functionality, we will use a StatefulWidget, which allows you to manage the state of your application. Here's a breakdown of the steps to accomplish this:
Step 1: Create a StatefulWidget
First, make sure that the widget holding the text fields is a StatefulWidget. This is important because we need to manage the number of text fields dynamically.
Step 2: Track the Number of Text Fields
Declare an int variable to keep track of how many text fields are currently displayed. We will start by initializing this variable to 1, as we want to display one text field initially.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Render Text Fields Dynamically
Within the build method of your widget, you will use a for loop to render text fields based on the count stored in numberOfTextFields. Here's how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Increment the Count with a Button Click
Now we need to link our button to an action that increments the count of text fields. This will be done inside the callback method for your button. You will call setState() to notify Flutter that the state has changed and re-render the UI.
[[See Video to Reveal this Text or Code Snippet]]
Step 5: Capturing Values from the Text Fields
To get the values of the text fields, you’ll need to create controllers for each TextField. When navigating to another page, you can gather these values and pass them as arguments. Here's a simple way to do it:
Create a List of TextEditingController to manage text fields:
[[See Video to Reveal this Text or Code Snippet]]
Update your loop to initialize each text field with a controller:
[[See Video to Reveal this Text or Code Snippet]]
When pressing the button to navigate to another page, use the controllers to gather the values:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following the steps outlined in this guide, you can effectively create a dynamic form in Flutter that allows users to add multiple text fields at runtime. This functionality enhances user engagement and makes your application more versatile.
With just a few lines of code, you can significantly elevate the user experience in your Flutter applications. Happy coding!