filmov
tv
L22. Top View of Binary Tree | C++ | Java
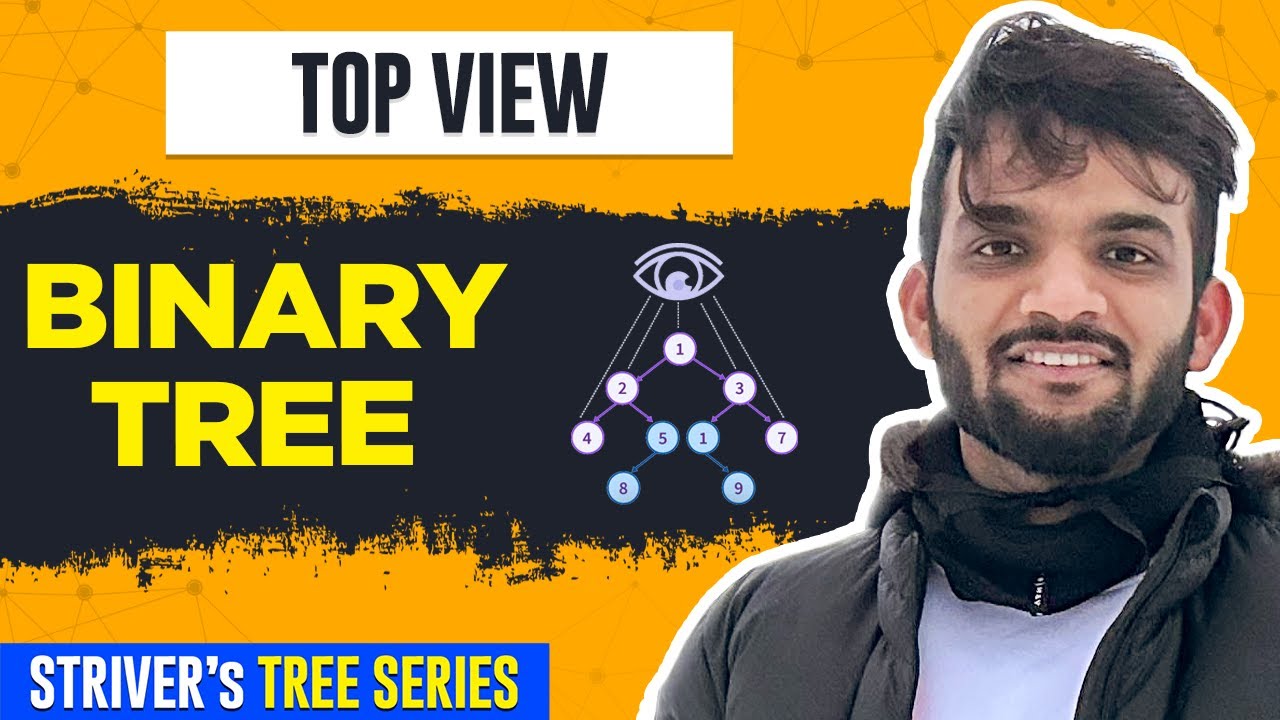
Показать описание
#treeSeries #striver #placements
L22. Top View of Binary Tree | C++ | Java
L22. | Top View of Binary Tree | Binary Trees | Live Coding
Top view of Binary Tree | Bottom view of Binary Tree | Binary Tree Data Structures Java | DSAOne #61
Lecture 64: Binary Tree FAANG Interview Questions || Part-2
Tree: Top view | HackerRank | Data Structure | Interview
Top View of Binary Tree | GeeForGeeks solution in Hindi
126 - Tree: Top View | Trees | Hackerrank Solution | Python
L23. Bottom View of Binary Tree | C++ | Java
L24. Right/Left View of Binary Tree | C++ | Java
Top View of a Tree | Love Babbar DSA Sheet | Amazon | Microsoft | Paytm 🔥 | GFG | Leetcode
Secret code to open Engineering mode
Left view of Binary Tree | Right view of Binary Tree | Left view of Tree | DSA-One Course #60
Keypad mobile me TikTok Kaise chalaye || How to open TikTok in keypad mobile || #shorts #youtube
L21. Vertical Order Traversal of Binary Tree | C++ | Java
Top View Of A Binarytree | Module : Trees | In Hindi | CPP | Video_28
Binary Tree Right Side View | BFS | Amazon | Accolite | Flipkart | Live Coding
Vertical Order Traversal of Binary Tree | Vertical Traversal of a Binary Tree | DSA-One Course #72
Binary Tree Right Side View | DFS | Amazon | Leetcode 199
L20. Boundary Traversal in Binary Tree | C++ | Java
Left View of Binary Trees | C++ Placement Course | Lecture 27.11
L29. Children Sum Property in Binary Tree | O(N) Approach | C++ | Java
Left view or Right View of Binary Tree | Tree Data Structure playlist C++ | Hello World | Hindi
FACULTY051 CS101 KUST20201 L22 V1 Introduction to Computing Hexadecimal Conversion
What are Channel Capacity and Code Rate?
Комментарии