filmov
tv
Python Programming Basics to Advanced | Recursive Functions and Memoization | Lab 28
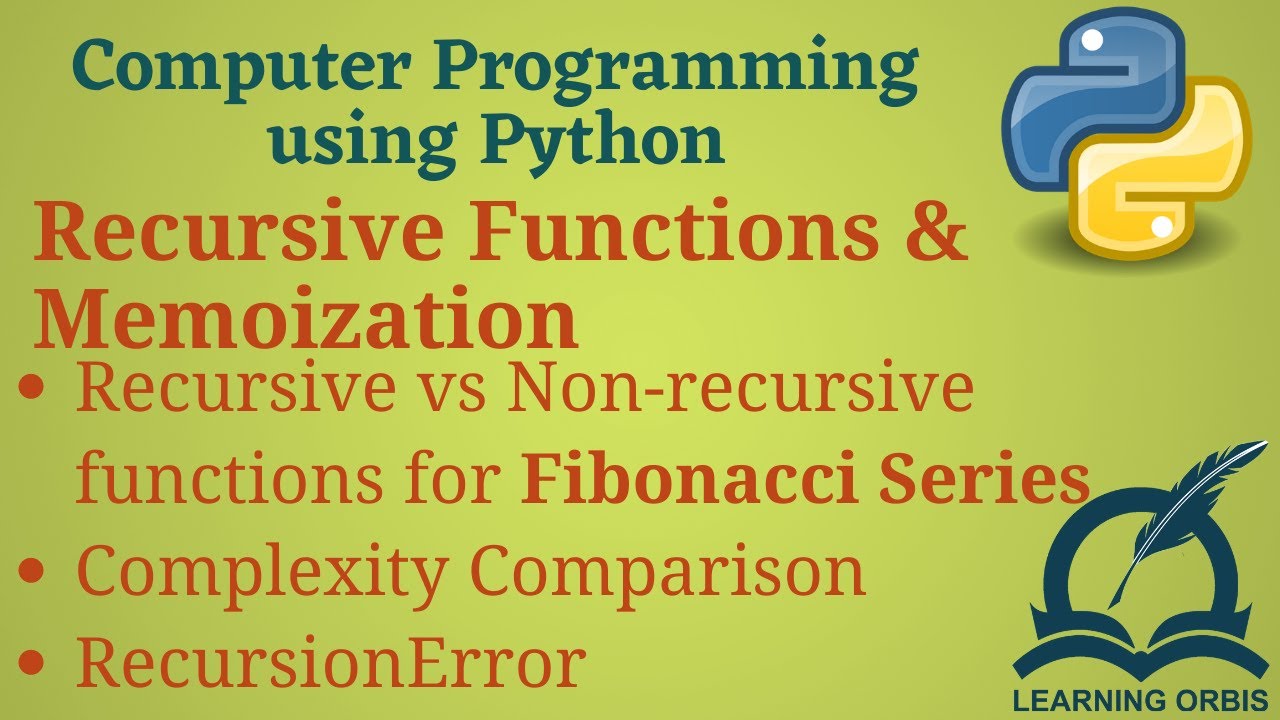
Показать описание
This Python programming playlist is designed to take beginners with zero programming experience to an expert level. The course covers installation, basic syntax, practical scenarios, and efficient logic building. The course material includes PDF handouts, review questions, and covers a wide range of topics, from data types to advanced functions like Lambda and Recursive functions, Generators, and JSON data parsing.
In this lesson we will study about two topics: Recursive Functions and Memoization. Recursive functions are the functions which call itself. We will compare the performance and complexity of Recursive and Non-Recursive functions and will see how we can use the concept of Memoization to improve the performance of any function.
Complete Playlist:
If you have the basic programming knowledge and interested to learn Object-Oriented Programming in Python, check out this playlist:
Lab Manual 28 can be downloaded from here:
Review Question:
1-Do a complete comparison of Recursive and Non-recursive function for the Factorial function. In comparison you have to discus following things:
- Complexity of non recursive and recursive function (without memoization).
- Improvement in complexity with memoization on Recursive function.
- Using lru_cache for memoization, what is minimum value of maxsize that can work.
- For a general number n, what should be minimum recursion limit which will not generate the RecursionError.
2- The Greatest Common Divisor (GCD) of two numbers can be found recursively as:
- Input arguments are two number a and b
- if second number is 0, return a.
- otherwise return GCD of a and a%b
Implement the GCD function with this logic.
#PythonProgramming #python #pythontutorial
In this lesson we will study about two topics: Recursive Functions and Memoization. Recursive functions are the functions which call itself. We will compare the performance and complexity of Recursive and Non-Recursive functions and will see how we can use the concept of Memoization to improve the performance of any function.
Complete Playlist:
If you have the basic programming knowledge and interested to learn Object-Oriented Programming in Python, check out this playlist:
Lab Manual 28 can be downloaded from here:
Review Question:
1-Do a complete comparison of Recursive and Non-recursive function for the Factorial function. In comparison you have to discus following things:
- Complexity of non recursive and recursive function (without memoization).
- Improvement in complexity with memoization on Recursive function.
- Using lru_cache for memoization, what is minimum value of maxsize that can work.
- For a general number n, what should be minimum recursion limit which will not generate the RecursionError.
2- The Greatest Common Divisor (GCD) of two numbers can be found recursively as:
- Input arguments are two number a and b
- if second number is 0, return a.
- otherwise return GCD of a and a%b
Implement the GCD function with this logic.
#PythonProgramming #python #pythontutorial
Комментарии