filmov
tv
[Python Programming Basics to Advanced] : Sets in Python | Lab 23
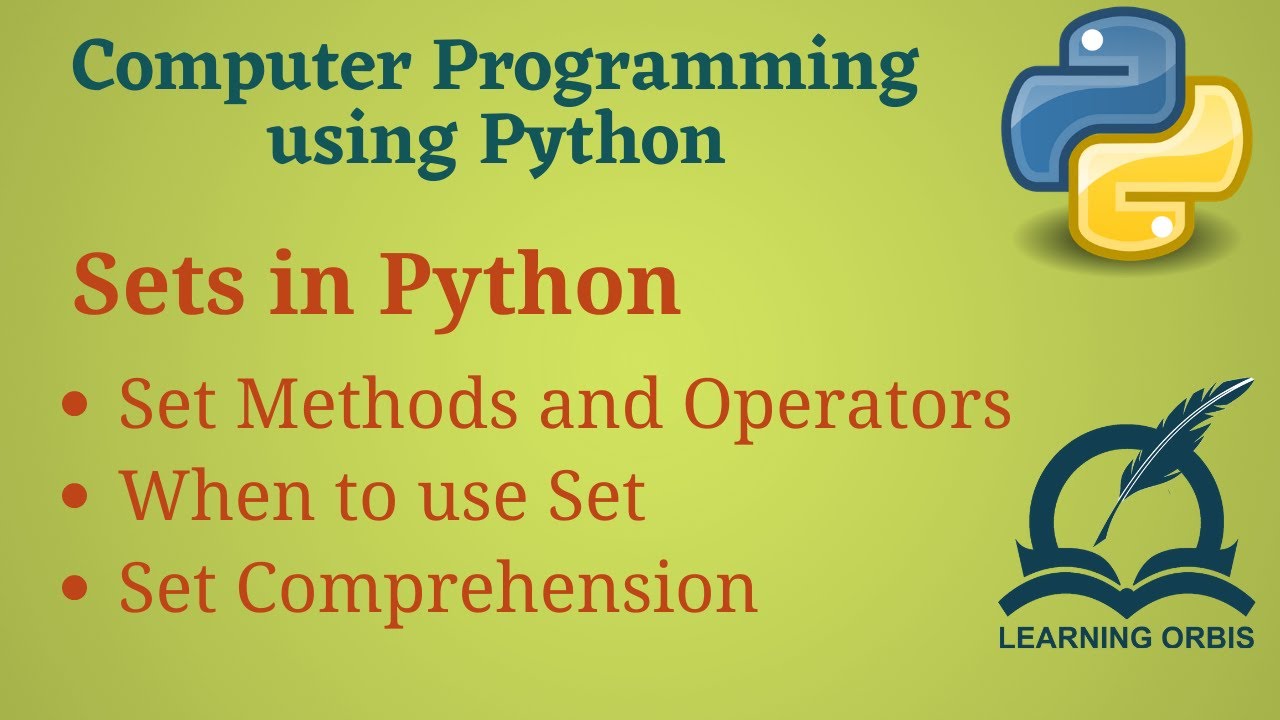
Показать описание
This Python programming playlist is designed to take beginners with zero programming experience to an expert level. The course covers installation, basic syntax, practical scenarios, and efficient logic building. The course material includes PDF handouts, review questions, and covers a wide range of topics, from data types to advanced functions like Lambda and Recursive functions, Generators, and JSON data parsing
In this lesson we will learn about Sets in Python. The data structure Set in Python follows the same rules as we see in Set Theory. A set does not have any order and it can not have the repeated elements.
We will see how we can use sets efficiently in different cases.
Following Tasks are completed:
Strong Password 10:23
Grid Paths 15:31
Finding the second largest number in a List 18:24
Set Comprehension 19:22
Mutable vs Immutable is discussed here:
List Comprehension is Discussed here:
Complete Playlist:
If you have the basic programming knowledge and interested to learn Object-Oriented Programming in Python, check out this playlist:
Lab Manual 23 can be downloaded from here:
Review Questions:
Suppose you have two lists names as a and b containing some numbers. You need another list as common elements between the lists a and b except the first and the last number of the list a.
#PythonProgramming #python #pythontutorial
In this lesson we will learn about Sets in Python. The data structure Set in Python follows the same rules as we see in Set Theory. A set does not have any order and it can not have the repeated elements.
We will see how we can use sets efficiently in different cases.
Following Tasks are completed:
Strong Password 10:23
Grid Paths 15:31
Finding the second largest number in a List 18:24
Set Comprehension 19:22
Mutable vs Immutable is discussed here:
List Comprehension is Discussed here:
Complete Playlist:
If you have the basic programming knowledge and interested to learn Object-Oriented Programming in Python, check out this playlist:
Lab Manual 23 can be downloaded from here:
Review Questions:
Suppose you have two lists names as a and b containing some numbers. You need another list as common elements between the lists a and b except the first and the last number of the list a.
#PythonProgramming #python #pythontutorial
Комментарии