filmov
tv
7 Functional Programming Techniques EVERY Developer Should Know
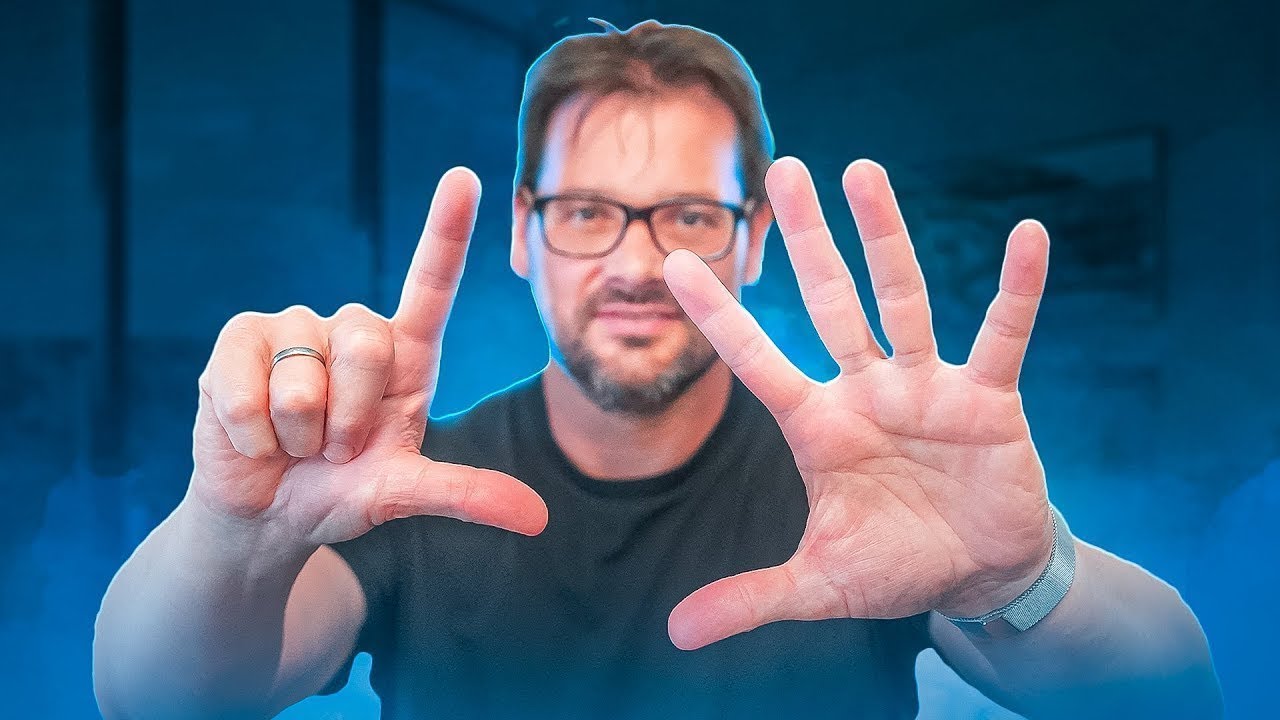
Показать описание
In this video, I'll walk you through 7 functional programming techniques and demonstrate how they work. Although Python is not a purely functional language, functional programming can significantly improve your Python skills.
🔖 Chapters:
0:00 Intro
0:32 #1. Recursion
4:45 #2. Structural Pattern Matching
6:17 #3. Immutability
8:49 #4. Pure Functions
11:11 #5. Higher-Order Functions
15:53 #6. Function Composition
18:42 #7. Lazy Evaluation
20:47 Outro
#arjancodes #softwaredesign #python
7 Functional Programming Techniques EVERY Developer Should Know
The HARDEST part about programming 🤦♂️ #code #programming #technology #tech #software #developer...
Best Programming Languages #programming #coding #javascript
Learn Functional Programming | Full 2-Hour Course
Functional programming - A general introduction
Day in My Life as a Quantum Computing Engineer!
How principled coders outperform the competition
STOP Learning These Programming Languages (for Beginners)
3 Tips I’ve learned after 2000 hours of Leetcode
Functional Programming on .NET - The Best of Both Worlds - Isaac Abraham - NDC Oslo 2024
Functional Programming Techniques in C#
Object-oriented Programming in 7 minutes | Mosh
Best programming language in 2023 || Top programming language from 2000 to 2023 😨🤯||#itdevelopment...
Neuroscientist explains the best exercise to improve brain function
How to Answer Any Question on a Test
Object Oriented Programming vs Functional Programming
32 Secret Combinations on Your Keyboard
Projects Every Programmer Should Try
Functional Programming in 7 Days : The Course Overview | packtpub.com
PHP 7 Functional Programming : Lazy versus Eager Evaluation | packtpub.com
Fastest Way to Learn ANY Programming Language: 80-20 rule
10 Design Patterns Explained in 10 Minutes
Functions & Methods | Java Complete Placement Course | Lecture 7
Developer Last Expression 😂 #shorts #developer #ytshorts #uiux #python #flutterdevelopment
Комментарии