filmov
tv
Subsets - Backtracking - Leetcode 78
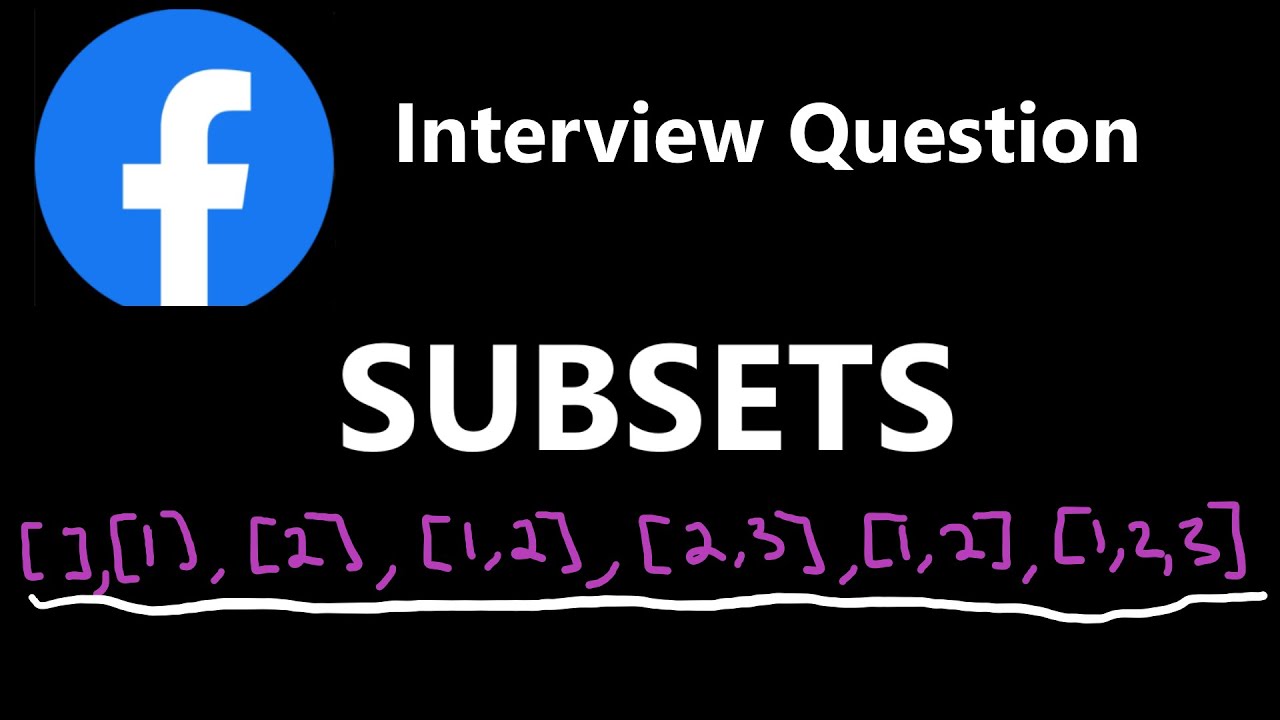
Показать описание
0:00 - Read the problem
1:15 - Drawing explanation
5:30 - Coding explanation
leetcode 78
#subset #permutation #python
Subsets - Backtracking - Leetcode 78
Subsets (LeetCode 78) | Full solution with backtracking examples | Interview | Study Algorithms
Subsets - Leetcode 78 - Recursive Backtracking (Python)
Subsets II - Backtracking - Leetcode 90 - Python
Facebook Backtracking Interview Question - Subsets - Leetcode 78
[Java] Leetcode 78/90. Subsets I/II [Backtracking #6]
Leetcode 78. Subsets [ Backtracking Algorithm + Code Explained] TC - O( N * 2^N)
(Old) Generate Subsets Given Array | Leetcode 78 | Backtracking
Subsets - Backtracking - Leetcode 78 | #dsa #backtracking #coding #leetcode
8 patterns to solve 80% Leetcode problems
Partition to K Equal Sum Subsets - Backtracking - Leetcode 698 - Python
Subsets | Leet code 78 | Theory explained + Python code | July Leet code day 11
How to generate subsets of an array - Inside code
Subsets | LeetCode 78 | C++, Java, Python | Power Set
Subsets | Leetcode 78 | Array | Recursion | Backtracking
Subsets - LeetCode 78 - Python
Generate All Possible Subsets | Return Power Set | Leetcode 78. | Bit Manipulation | Two for loops
3 Tips I’ve learned after 2000 hours of Leetcode
(Remade) Subsets I | Leetcode 78 | Backtracking
Leetcode 2597: The Number of Beautiful Subsets | Backtracking | Subsets
Leetcode 78 | Subsets | Backtracking
Subsets in JavaScript | Backtracking | Leetcode 78 Medium | FAANG tastic
Subsets - Backtracking And Recursion (Leetcode 78)
78. Subsets | Backtracking Series | INTRODUCTION | LEETCODE
Комментарии