filmov
tv
Partition to K Equal Sum Subsets - Backtracking - Leetcode 698 - Python
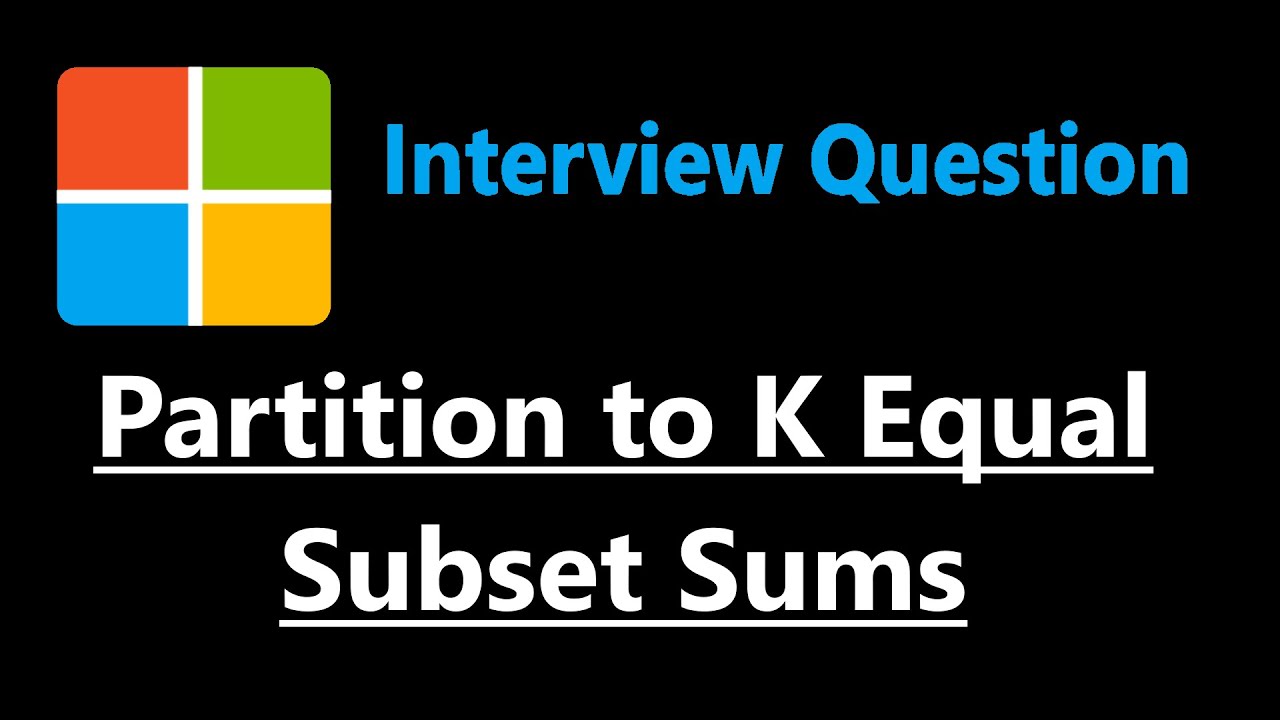
Показать описание
Problem Link: Partition to K Equal Sum Subsets
0:00 - Read the problem
3:09 - Explain Solution #1
5:42 - Explain Solution #2
9:32 - Coding Explanation
leetcode 698
#sorted #array #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Partition to K Equal Sum Subsets - Backtracking - Leetcode 698 - Python
Leetcode - Partition to K Equal Sum Subsets (Python)
Partition To K Equal Sum Subsets From An Array of Integers - The Backtracking Approach
Partition to K Equal Sum Subsets | LeetCode 698 | Coders Camp
Partition Equal Subset Sum - Dynamic Programming - Leetcode 416 - Python
Partition to K Equal Sum Subsets - source code & running time recurrence relation
LeetCode 698. Partition to K Equal Sum Subsets
Day 30/30 | Partition to K Equal Sum Subsets | September LeetCoding Challenge 2021 | DP | BitMasking
698. Partition to K Equal Sum Subsets - Day 30/30 Leetcode September Challenge
Partition to K Equal Sum Subsets | Leetcode 698 | Live coding session 🔥🔥🔥
Partition to K Equal Sum Subsets #leetcode
Ep16 - Partition to K equal sum subsets | DSA | Codes available in description
DP 15. Partition Equal Subset Sum | DP on Subsequences
LeetCode in Java - Partition to K Equal Sum Subsets
Partition equal subset sum | Equal sum partition | Dynamic Programming | Leetcode #416
Partition of a set into K subsets with equal sum using BitMask and DP
LeetCode 698. Partition to K Equal Sum Subsets || medium level problem || Easy Method
Partition to K Equal Sum Subsets
Partition array into k equal sum subsets | Backtracking | Detailed solution | Love Babbar DSA sheet
Partition Problem - 2 subsets of equal sum, as closely as possible - tutorial and source code
Partition to K Equal Sum Subsets || 30 September leecode week 5 solution || today leetcode solution
698. Partition to K Equal Sum Subsets | Leetcode
LeetCode - 698. Partition to K Equal Sum Subsets | Backtracking | Java
leetcode 698. Partition K equal sum subsets
Комментарии