filmov
tv
Understanding 'Maximum Recursion Depth Exceeded' in Python
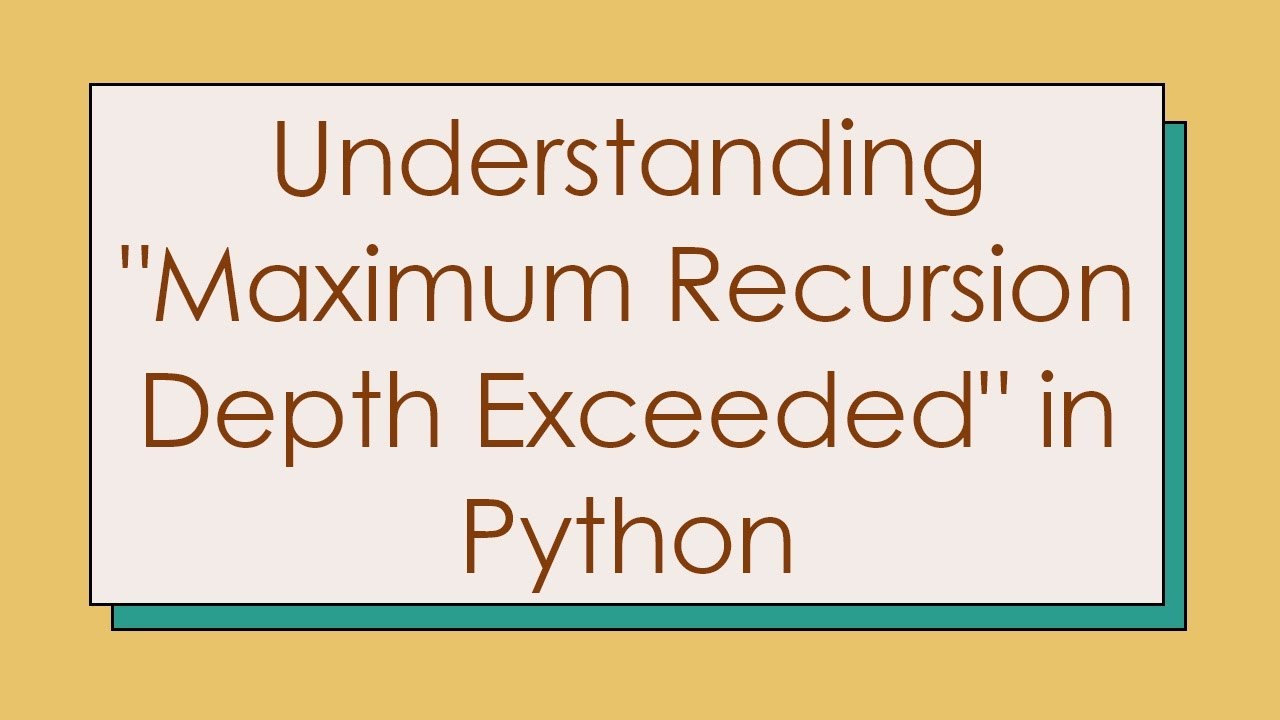
Показать описание
Summary: Learn what causes the "Maximum Recursion Depth Exceeded" error in Python, how to handle it, and various ways to avoid this common issue in your code.
---
Understanding "Maximum Recursion Depth Exceeded" in Python
When working with Python, especially in tasks involving recursion, you might encounter the error message: "maximum recursion depth exceeded while calling a Python object." This error can halt your code's execution and may seem perplexing when faced for the first time. Let's break down what causes this error and explore strategies to manage and prevent it.
What is Recursion?
Recursion is a programming technique where a function calls itself directly or indirectly to solve a problem. It is often used when a problem can be divided into similar sub-problems, making the solution more elegant and understandable. A classic example is the calculation of factorials:
[[See Video to Reveal this Text or Code Snippet]]
Understanding the Error
In Python, the default recursion limit is a safeguard to prevent infinite recursion from causing a stack overflow, which would crash the interpreter. The limit can be checked using the sys module:
[[See Video to Reveal this Text or Code Snippet]]
By default, this limit is usually set to 1000. When your recursive function exceeds this limit, Python raises a RecursionError with the message: "maximum recursion depth exceeded while calling a Python object."
Causes of the Error
The two most common causes of this error are:
Infinite Recursion: This occurs when the base case, which stops the recursive calls, is not properly defined or is missing.
Deep Recursion: Even with a correctly defined base case, the problem might inherently require too many recursive calls, exceeding Python's default limit.
How to Handle the Error
Improving the Base Case
Ensure that the base case in your recursive function is correctly implemented and able to halt the recursion. For instance:
[[See Video to Reveal this Text or Code Snippet]]
Increasing the Recursion Limit
[[See Video to Reveal this Text or Code Snippet]]
Refactoring to Iteration
Whenever possible, refactor your recursive solution into an iterative one, which can typically handle larger inputs without the risk of a recursion limit error:
[[See Video to Reveal this Text or Code Snippet]]
Tail Recursion Optimization
In some programming languages, tail-recursive functions can be optimized by the compiler to avoid increasing the call stack. Python does not perform this optimization natively, but you can simulate tail recursion optimization by using an iterative approach or third-party libraries.
Conclusion
The “maximum recursion depth exceeded while calling a Python object” error is an important reminder of the limitations inherent to recursive functions. Understanding the causes, knowing when to increase the recursion limit, and being able to refactor recursive solutions into iterative ones will help you manage and avoid this common issue. Always strive for the most efficient and maintainable solution for your problem.
---
Understanding "Maximum Recursion Depth Exceeded" in Python
When working with Python, especially in tasks involving recursion, you might encounter the error message: "maximum recursion depth exceeded while calling a Python object." This error can halt your code's execution and may seem perplexing when faced for the first time. Let's break down what causes this error and explore strategies to manage and prevent it.
What is Recursion?
Recursion is a programming technique where a function calls itself directly or indirectly to solve a problem. It is often used when a problem can be divided into similar sub-problems, making the solution more elegant and understandable. A classic example is the calculation of factorials:
[[See Video to Reveal this Text or Code Snippet]]
Understanding the Error
In Python, the default recursion limit is a safeguard to prevent infinite recursion from causing a stack overflow, which would crash the interpreter. The limit can be checked using the sys module:
[[See Video to Reveal this Text or Code Snippet]]
By default, this limit is usually set to 1000. When your recursive function exceeds this limit, Python raises a RecursionError with the message: "maximum recursion depth exceeded while calling a Python object."
Causes of the Error
The two most common causes of this error are:
Infinite Recursion: This occurs when the base case, which stops the recursive calls, is not properly defined or is missing.
Deep Recursion: Even with a correctly defined base case, the problem might inherently require too many recursive calls, exceeding Python's default limit.
How to Handle the Error
Improving the Base Case
Ensure that the base case in your recursive function is correctly implemented and able to halt the recursion. For instance:
[[See Video to Reveal this Text or Code Snippet]]
Increasing the Recursion Limit
[[See Video to Reveal this Text or Code Snippet]]
Refactoring to Iteration
Whenever possible, refactor your recursive solution into an iterative one, which can typically handle larger inputs without the risk of a recursion limit error:
[[See Video to Reveal this Text or Code Snippet]]
Tail Recursion Optimization
In some programming languages, tail-recursive functions can be optimized by the compiler to avoid increasing the call stack. Python does not perform this optimization natively, but you can simulate tail recursion optimization by using an iterative approach or third-party libraries.
Conclusion
The “maximum recursion depth exceeded while calling a Python object” error is an important reminder of the limitations inherent to recursive functions. Understanding the causes, knowing when to increase the recursion limit, and being able to refactor recursive solutions into iterative ones will help you manage and avoid this common issue. Always strive for the most efficient and maintainable solution for your problem.