filmov
tv
python maximum recursion depth exceeded
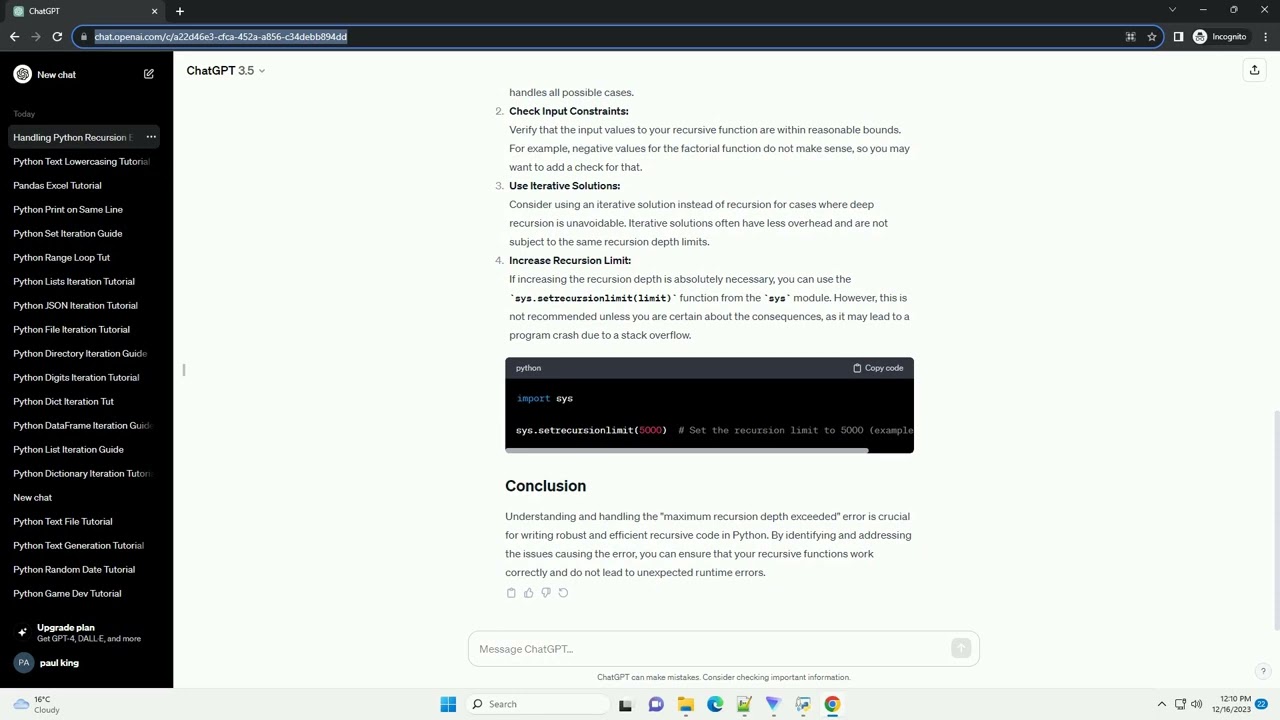
Показать описание
Title: Understanding and Handling "Maximum Recursion Depth Exceeded" in Python
Recursion is a powerful programming technique where a function calls itself in order to solve a problem. However, in Python, there is a limit to how deep recursion can go. When this limit is reached, the interpreter raises a RecursionError with the message "maximum recursion depth exceeded." In this tutorial, we'll explore the reasons behind this error, how to identify it, and strategies for handling it.
The "maximum recursion depth exceeded" error occurs when the depth of the function calls exceeds the maximum limit set by Python. This limit is in place to prevent infinite recursion, which could lead to a stack overflow.
To identify the issue causing the recursion error, look for a function that calls itself without a proper termination condition or a termination condition that is never met.
Let's consider an example where a function calculates the factorial of a number using recursion. This code intentionally contains a flaw to demonstrate the recursion depth error.
In this example, the factorial function calls itself with n - 1. However, if the input is not carefully controlled, it may lead to an infinite recursion.
Check the Termination Condition:
Ensure that your recursive function has a proper termination condition. In the factorial example, the termination condition is if n == 0:. Make sure it is correct and handles all possible cases.
Check Input Constraints:
Verify that the input values to your recursive function are within reasonable bounds. For example, negative values for the factorial function do not make sense, so you may want to add a check for that.
Use Iterative Solutions:
Consider using an iterative solution instead of recursion for cases where deep recursion is unavoidable. Iterative solutions often have less overhead and are not subject to the same recursion depth limits.
Increase Recursion Limit:
Understanding and handling the "maximum recursion depth exceeded" error is crucial for writing robust and efficient recursive code in Python. By identifying and addressing the issues causing the error, you can ensure that your recursive functions work correctly and do not lead to unexpected runtime
Recursion is a powerful programming technique where a function calls itself in order to solve a problem. However, in Python, there is a limit to how deep recursion can go. When this limit is reached, the interpreter raises a RecursionError with the message "maximum recursion depth exceeded." In this tutorial, we'll explore the reasons behind this error, how to identify it, and strategies for handling it.
The "maximum recursion depth exceeded" error occurs when the depth of the function calls exceeds the maximum limit set by Python. This limit is in place to prevent infinite recursion, which could lead to a stack overflow.
To identify the issue causing the recursion error, look for a function that calls itself without a proper termination condition or a termination condition that is never met.
Let's consider an example where a function calculates the factorial of a number using recursion. This code intentionally contains a flaw to demonstrate the recursion depth error.
In this example, the factorial function calls itself with n - 1. However, if the input is not carefully controlled, it may lead to an infinite recursion.
Check the Termination Condition:
Ensure that your recursive function has a proper termination condition. In the factorial example, the termination condition is if n == 0:. Make sure it is correct and handles all possible cases.
Check Input Constraints:
Verify that the input values to your recursive function are within reasonable bounds. For example, negative values for the factorial function do not make sense, so you may want to add a check for that.
Use Iterative Solutions:
Consider using an iterative solution instead of recursion for cases where deep recursion is unavoidable. Iterative solutions often have less overhead and are not subject to the same recursion depth limits.
Increase Recursion Limit:
Understanding and handling the "maximum recursion depth exceeded" error is crucial for writing robust and efficient recursive code in Python. By identifying and addressing the issues causing the error, you can ensure that your recursive functions work correctly and do not lead to unexpected runtime