filmov
tv
Coding Challenge #89: Langton's Ant
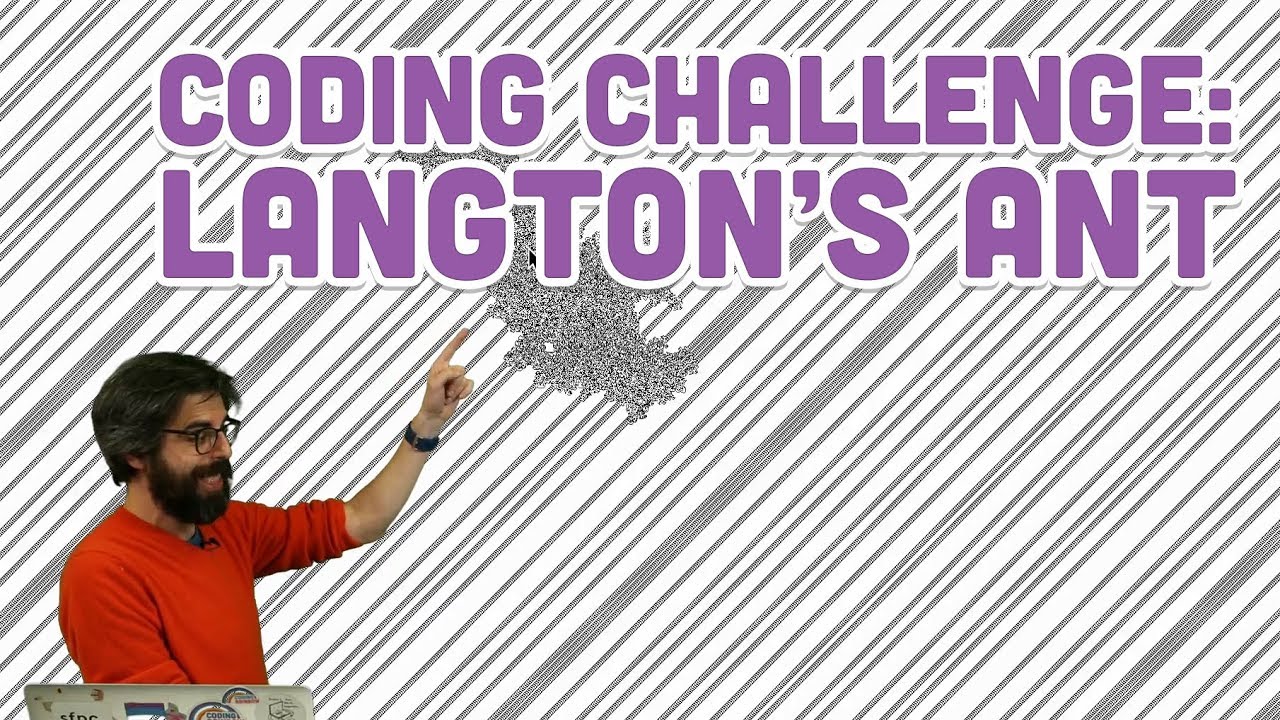
Показать описание
References:
Videos:
Related Coding Challenges:
Timestamps:
0:00 Introduction and setup code!
1:46 Writing the algorithm for the motion of Langton's ant
9:09 Visualizing Langton's Ant
12:33 Debugging and testing!
14:53 Refactoring the code!
20:06 Things to try!
20:46 Outro!
Editing by Mathieu Blanchette
Animations by Jason Heglund
Music from Epidemic Sound
#langtonsant #cellularautomata #processing #java
Coding Challenge #89: Langton's Ant
Langton's Ant
Faster Langton's Ant Full Adder
Langton's ant a cellular automaton maths simulation in flutter
Langton's ant LLRR 235 billion iterations
Langton’s ant [gnuplot]
Code Langton’s Ant With JavaScript
Langton's ant
Langton's Ant in Pygame
Broken leg Langton's Ant; N=11167
Bauten von Langton-Ameisen
Python Langton's Ant in five seconds
Langton's Ant
Coding 'Langton's Ant' Cellular Automaton in C++/ SFML
Langton's ant RLR 4 billion iterations
Langton's ant
Langton's Ant (RRLLLRLLLRRR - Moving Triangle)
How to Code Langton's Ant! || Scratch Tutorial
Livestream #4: Langtons Ant
Langton's Ant in C
Langtons Ant | Showcase
Langton's ant colony on excel.
Langtons Ant and Others in Processing
Langton's Ant - Cellular Automaton in Unity
Комментарии