filmov
tv
String Format Validation | C Programming Example
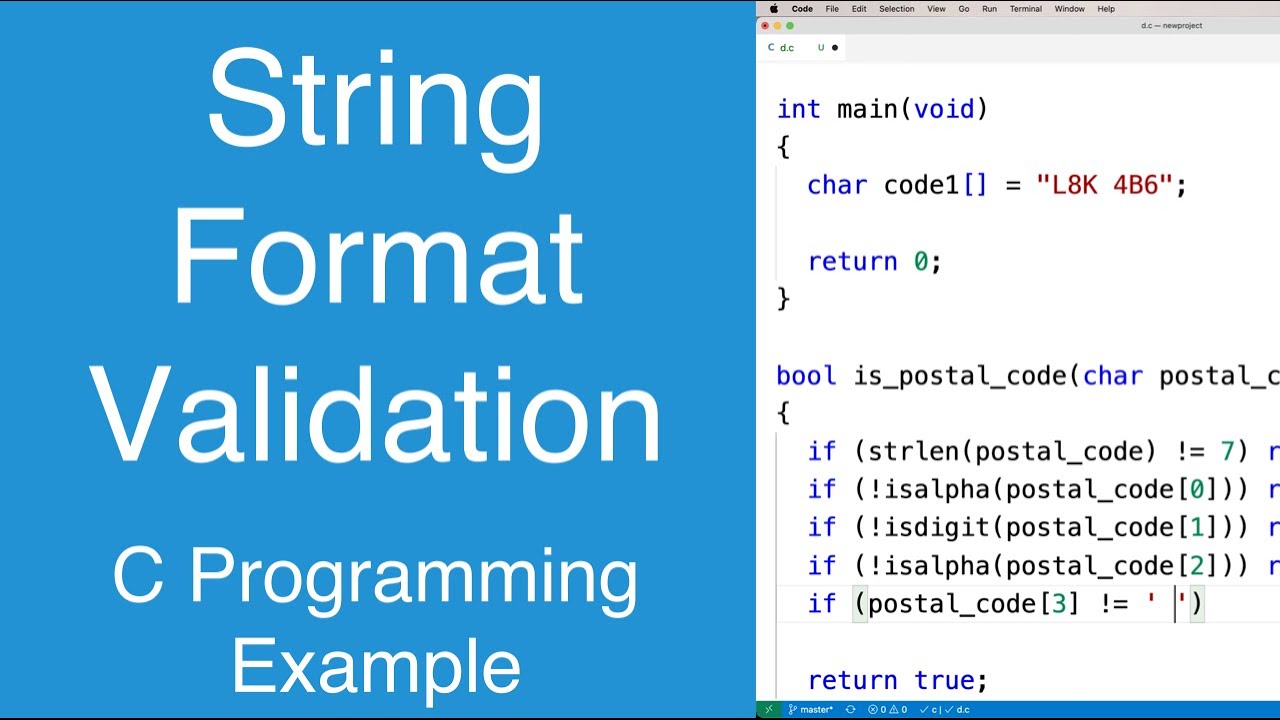
Показать описание
String Format Validation | C Programming Example
Format strings in C
Advanced Integer Input Validation | C Programming Example
A simple Format String exploit example - bin 0x11
C# Validation: Checking If a String Is a Number
Adding compile-time format validation to your string.Format calls
C# - String Validation (#9)
Additional String Validation Checks | C# Coding 2019 | NerdHouseGeeks.com
C# - String Validation [DEMO] (#10)
C# DSA: String Validation (Episode:2)
Simple alphanumeric string validation
User Validation in #c++ with #do-while #loop ? | #programming
User Input Validation With A Do-While Loop | C Programming Example
Fluent Validation in C# - The Powerful Yet Easy Data Validation Tool
C++ Validation
C++ : Email validation in C++
FA20 Code Sprint C: Week 05 - Question from Class - Validation & Type Checking Form Inputs
Integer input validation in C++ || How to Validated Integer Input in C++ || Input Validation in C++
Be Careful When Using scanf() in C
AND Operator Use to Chake Username Password validation in C Programming #c #coding #programming
MediatR Validation and the Chain of Responsibility Pattern
C++ Input Validation
C49 - C program to validate numerical values | number validation in c
Check Email Validation Using C++ [ With Source Code ]
Комментарии