filmov
tv
Troubleshooting RLE String Expansion: A Guide to Fixing Undefined Behavior in C Programs
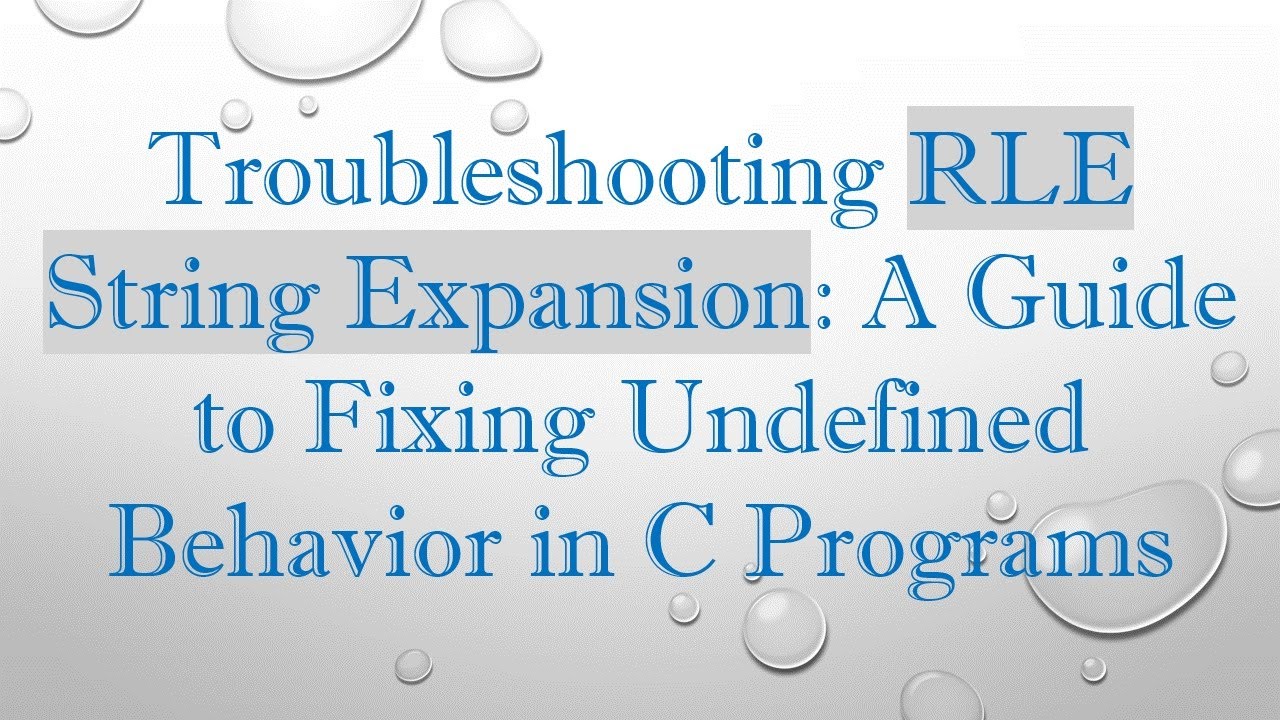
Показать описание
Discover how to resolve issues with Run Length Encoding string expansion in C by understanding undefined behavior and proper memory management techniques.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Strange output when expanding a RLE string in C
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Problem
If you've ever worked with C programming, you're no stranger to the reliance on memory management. A common exercise involves using Run Length Encoding (RLE) to compress and decompress data. However, even experienced developers can stumble upon unexpected behaviors when expanding RLE strings.
Recently, a programmer faced an issue where their RLE expansion function produced strange outputs on certain lines of their ASCII art. Although the compression function worked flawlessly, the expansion produced inconsistent results, prompting a frustrating search for the root cause.
In this post, we'll explore the reasons behind these inconsistencies and provide a structured solution to ensure proper memory management when dealing with string manipulations in C.
The Core Issue: Undefined Behavior
Upon examining the problematic code segment, one of the key culprits was identified as the way strings were manipulated. Specifically, the use of the strncat function posed a significant risk:
[[See Video to Reveal this Text or Code Snippet]]
Why This Leads to Problems
Null-Termination Requirement:
The strncat function requires the destination string (output in this case) to be a proper null-terminated string. This means it should end with a \0 character, signaling the end of the string.
Uninitialized Memory:
When you allocate memory with malloc, the contents of that memory are indeterminate — in layman's terms, they could be anything and might not include a null terminator. When strncat is executed, it references memory locations that may lead to undefined behavior.
The Solution: Proper Memory Initialization
The good news is that there's a straightforward fix for this issue. To ensure that strings are properly initialized, we can replace the use of malloc with calloc. Unlike malloc, calloc initializes the allocated memory to zero, making it safe to treat the memory allocated as a null-terminated string straight away.
Step-by-Step Fix
Change Memory Allocation:
Replace malloc with calloc when allocating memory for the output string:
[[See Video to Reveal this Text or Code Snippet]]
Verify Output Before Appending:
Always ensure that the destination string is indeed null-terminated before attempting to append characters.
Debugging Techniques:
If issues persist, consider using debugging tools to check for memory leaks or buffer overflows.
Implementation
Here’s a brief illustration of how to implement this in your code:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Memory management in C is a critical aspect that can sometimes elude even the best programmers. In our example, the key to resolving the strange outputs in RLE string expansion was recognizing the necessity of proper string initialization and ensuring that all strings are accurately terminated. By using calloc, we can prevent the undefined behavior that leads to unexpected results.
By following these guidelines, you'll be better equipped to handle similar issues in your C programming ventures, while also creating more robust and reliable code. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Strange output when expanding a RLE string in C
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Problem
If you've ever worked with C programming, you're no stranger to the reliance on memory management. A common exercise involves using Run Length Encoding (RLE) to compress and decompress data. However, even experienced developers can stumble upon unexpected behaviors when expanding RLE strings.
Recently, a programmer faced an issue where their RLE expansion function produced strange outputs on certain lines of their ASCII art. Although the compression function worked flawlessly, the expansion produced inconsistent results, prompting a frustrating search for the root cause.
In this post, we'll explore the reasons behind these inconsistencies and provide a structured solution to ensure proper memory management when dealing with string manipulations in C.
The Core Issue: Undefined Behavior
Upon examining the problematic code segment, one of the key culprits was identified as the way strings were manipulated. Specifically, the use of the strncat function posed a significant risk:
[[See Video to Reveal this Text or Code Snippet]]
Why This Leads to Problems
Null-Termination Requirement:
The strncat function requires the destination string (output in this case) to be a proper null-terminated string. This means it should end with a \0 character, signaling the end of the string.
Uninitialized Memory:
When you allocate memory with malloc, the contents of that memory are indeterminate — in layman's terms, they could be anything and might not include a null terminator. When strncat is executed, it references memory locations that may lead to undefined behavior.
The Solution: Proper Memory Initialization
The good news is that there's a straightforward fix for this issue. To ensure that strings are properly initialized, we can replace the use of malloc with calloc. Unlike malloc, calloc initializes the allocated memory to zero, making it safe to treat the memory allocated as a null-terminated string straight away.
Step-by-Step Fix
Change Memory Allocation:
Replace malloc with calloc when allocating memory for the output string:
[[See Video to Reveal this Text or Code Snippet]]
Verify Output Before Appending:
Always ensure that the destination string is indeed null-terminated before attempting to append characters.
Debugging Techniques:
If issues persist, consider using debugging tools to check for memory leaks or buffer overflows.
Implementation
Here’s a brief illustration of how to implement this in your code:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Memory management in C is a critical aspect that can sometimes elude even the best programmers. In our example, the key to resolving the strange outputs in RLE string expansion was recognizing the necessity of proper string initialization and ensuring that all strings are accurately terminated. By using calloc, we can prevent the undefined behavior that leads to unexpected results.
By following these guidelines, you'll be better equipped to handle similar issues in your C programming ventures, while also creating more robust and reliable code. Happy coding!