filmov
tv
Mastering Object Cloning in JavaScript: How to Clone Object Without Reference
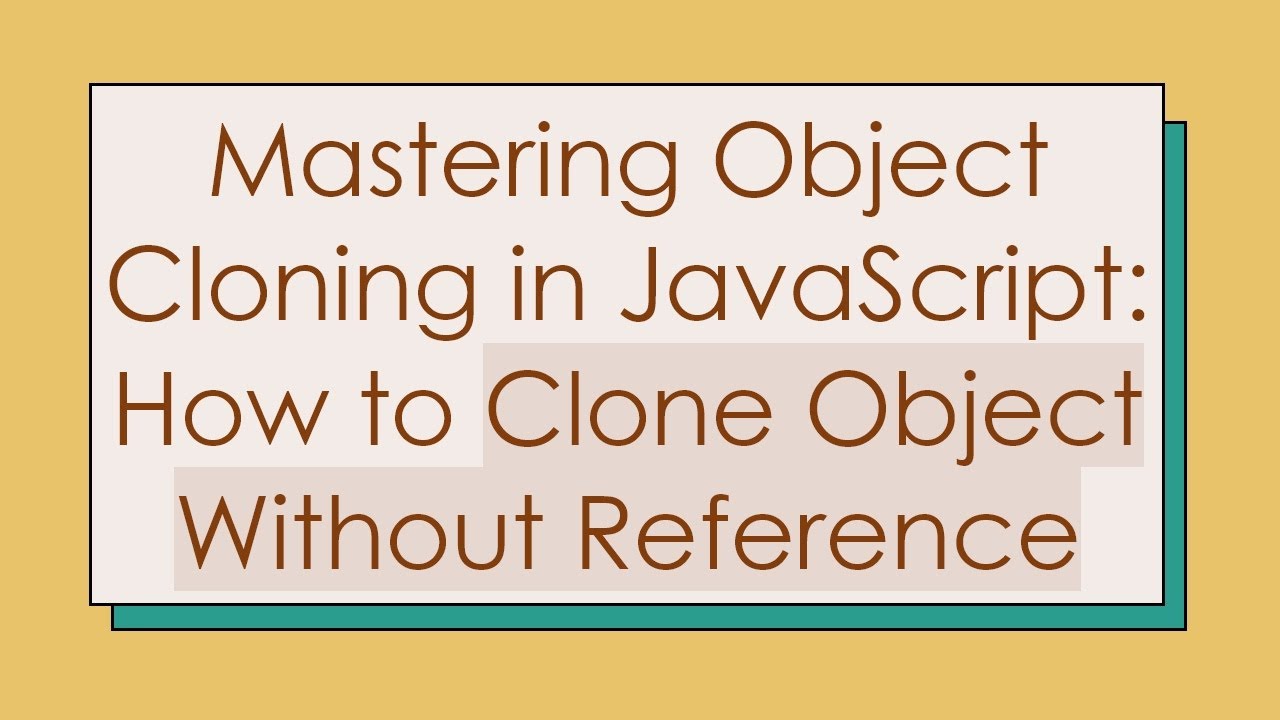
Показать описание
Summary: Learn various methods to clone objects in JavaScript without creating any reference issues. Perfect for developers looking to understand cloning techniques.
---
Mastering Object Cloning in JavaScript: How to Clone Object Without Reference
When working with objects in JavaScript, cloning them without creating references can often be necessary. If not handled properly, the cloned object might end up modifying the original object due to the reference link. This guide delves into effective methods to clone an object without reference in JavaScript.
Shallow Cloning vs. Deep Cloning
Before we dive into solutions, it is essential to understand the difference between shallow and deep cloning.
Shallow Cloning: Only the immediate properties of the object are copied. If the object contains nested objects, shallow cloning will still create references to those nested objects.
Deep Cloning: A full copy of the entire object, including all nested objects, is created. There are no shared references between the original and the cloned object.
Shallow Cloning Techniques
[[See Video to Reveal this Text or Code Snippet]]
Be aware that nested objects will still be referenced.
Using Spread Operator
The spread operator (...) can also be used to achieve shallow cloning.
[[See Video to Reveal this Text or Code Snippet]]
Again, nested objects will retain their references.
Deep Cloning Techniques
JSON parse/stringify Method
A simple yet effective way to deep clone an object is by using the JSON.parse(JSON.stringify()) method.
[[See Video to Reveal this Text or Code Snippet]]
This method works well for objects that can be serialized into JSON, but fails for objects containing functions, undefined, or other non-serializable data types.
Using Recursion
For complete control, you can write a recursive function to handle deep cloning.
[[See Video to Reveal this Text or Code Snippet]]
This technique ensures that every level of the object is cloned, creating no references to the original object.
Libraries for Deep Cloning
Several JavaScript libraries, such as lodash or cloneDeep, provide ready-to-use functions for deep cloning.
[[See Video to Reveal this Text or Code Snippet]]
Choosing the Right Method
The choice of clone method largely depends on the complexity and requirements of your project. For simple, shallow copies, the spread operator is both concise and effective. For deep clones, particularly with complex objects, custom recursive functions or robust libraries are the best bets.
By mastering these cloning techniques, you can more effectively manage object manipulation in JavaScript, ensuring that your copies are truly independent of the originals. Happy coding!
---
Mastering Object Cloning in JavaScript: How to Clone Object Without Reference
When working with objects in JavaScript, cloning them without creating references can often be necessary. If not handled properly, the cloned object might end up modifying the original object due to the reference link. This guide delves into effective methods to clone an object without reference in JavaScript.
Shallow Cloning vs. Deep Cloning
Before we dive into solutions, it is essential to understand the difference between shallow and deep cloning.
Shallow Cloning: Only the immediate properties of the object are copied. If the object contains nested objects, shallow cloning will still create references to those nested objects.
Deep Cloning: A full copy of the entire object, including all nested objects, is created. There are no shared references between the original and the cloned object.
Shallow Cloning Techniques
[[See Video to Reveal this Text or Code Snippet]]
Be aware that nested objects will still be referenced.
Using Spread Operator
The spread operator (...) can also be used to achieve shallow cloning.
[[See Video to Reveal this Text or Code Snippet]]
Again, nested objects will retain their references.
Deep Cloning Techniques
JSON parse/stringify Method
A simple yet effective way to deep clone an object is by using the JSON.parse(JSON.stringify()) method.
[[See Video to Reveal this Text or Code Snippet]]
This method works well for objects that can be serialized into JSON, but fails for objects containing functions, undefined, or other non-serializable data types.
Using Recursion
For complete control, you can write a recursive function to handle deep cloning.
[[See Video to Reveal this Text or Code Snippet]]
This technique ensures that every level of the object is cloned, creating no references to the original object.
Libraries for Deep Cloning
Several JavaScript libraries, such as lodash or cloneDeep, provide ready-to-use functions for deep cloning.
[[See Video to Reveal this Text or Code Snippet]]
Choosing the Right Method
The choice of clone method largely depends on the complexity and requirements of your project. For simple, shallow copies, the spread operator is both concise and effective. For deep clones, particularly with complex objects, custom recursive functions or robust libraries are the best bets.
By mastering these cloning techniques, you can more effectively manage object manipulation in JavaScript, ensuring that your copies are truly independent of the originals. Happy coding!