filmov
tv
Mastering JavaScript: The Ultimate Guide to Deep Copying Objects and Arrays
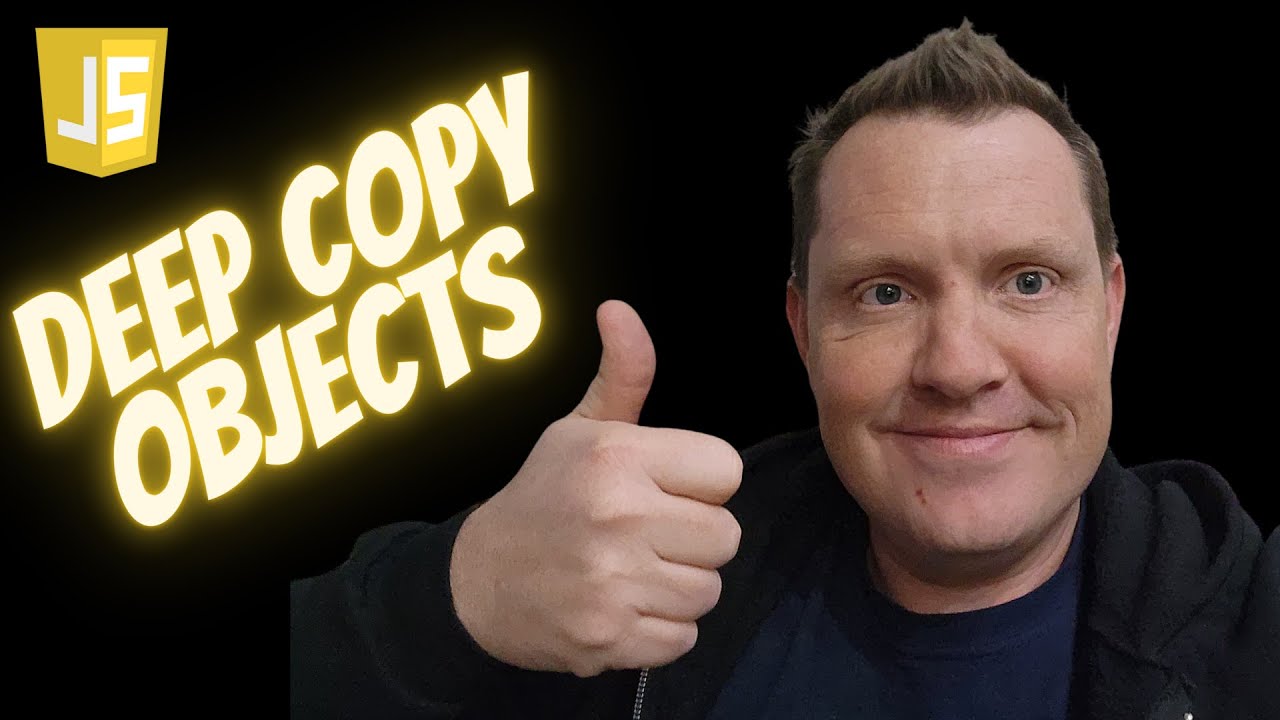
Показать описание
#coding #codingbootcamp #softwaredeveloper
Enter to win free tuition! Enter below!
The usual methods of copying an object or array only make a shallow copy, so deeply-nested references are a problem. You need a deep copy if a JavaScript object contains other objects.
What is a shallow copy?
Making a shallow copy of an array or object means creating new references to the primitive values inside the object, copying them.
That means that changes to the original array will not affect the copied array, which is what would happen if only the reference to the array had been copied (such as would occur with the assignment operator =).
A shallow copy refers to the fact that only one level is copied, and that will work fine for an array or object containing only primitive values.
For objects and arrays containing other objects or arrays, copying these objects requires a deep copy. Otherwise, changes made to the nested references will change the data nested in the original object or array.
Shallow copy using …
1.The spread operator (…) is a convenient way to make a shallow copy of an array or object —when there is no nesting, it works great.
Shallow copy using .slice()
2.For arrays specifically, using the built-in .slice() method works the same as the spread operator — creating a shallow copy of one level.
Shallow copy using .assign()
What is a deep copy?
For objects and arrays containing other objects or arrays, copying these objects requires a deep copy. Otherwise, changes made to the nested references will change the data nested in the original object or array.
This is compared to a shallow copy, which works fine for an object or array containing only primitive values, but will fail for any object or array that has nested references to other objects or arrays.
Understanding the difference between == and === can help visually see the difference between shallow and deep copy, as the strict equality operator (===) shows that the nested references are the same.
Deep copy with lodash
1.The library lodash is the most common way JavaScript developers make a deep copy. Lodash’s name comes from the library being referenced as an underscore (_), a “low dash” or lodash for short.
Deep copy with Ramda
2.The functional programming library Ramda includes the R.clone() method, which makes a deep copy of an object or array.
Deep copy with custom function
3.It is pretty easy to write a recursive JavaScript function that will make a deep copy of nested objects or arrays.
Deep copy with JSON.parse/stringify
4.If your data fits the specifications (see below), then JSON.stringify followed by JSON.parse will deep copy your object.
To demonstrate some reasons why this method is not generally recommended, here is an example of creating a deep copy using JSON.parse(JSON.stringify(object))
Enter to win free tuition! Enter below!
The usual methods of copying an object or array only make a shallow copy, so deeply-nested references are a problem. You need a deep copy if a JavaScript object contains other objects.
What is a shallow copy?
Making a shallow copy of an array or object means creating new references to the primitive values inside the object, copying them.
That means that changes to the original array will not affect the copied array, which is what would happen if only the reference to the array had been copied (such as would occur with the assignment operator =).
A shallow copy refers to the fact that only one level is copied, and that will work fine for an array or object containing only primitive values.
For objects and arrays containing other objects or arrays, copying these objects requires a deep copy. Otherwise, changes made to the nested references will change the data nested in the original object or array.
Shallow copy using …
1.The spread operator (…) is a convenient way to make a shallow copy of an array or object —when there is no nesting, it works great.
Shallow copy using .slice()
2.For arrays specifically, using the built-in .slice() method works the same as the spread operator — creating a shallow copy of one level.
Shallow copy using .assign()
What is a deep copy?
For objects and arrays containing other objects or arrays, copying these objects requires a deep copy. Otherwise, changes made to the nested references will change the data nested in the original object or array.
This is compared to a shallow copy, which works fine for an object or array containing only primitive values, but will fail for any object or array that has nested references to other objects or arrays.
Understanding the difference between == and === can help visually see the difference between shallow and deep copy, as the strict equality operator (===) shows that the nested references are the same.
Deep copy with lodash
1.The library lodash is the most common way JavaScript developers make a deep copy. Lodash’s name comes from the library being referenced as an underscore (_), a “low dash” or lodash for short.
Deep copy with Ramda
2.The functional programming library Ramda includes the R.clone() method, which makes a deep copy of an object or array.
Deep copy with custom function
3.It is pretty easy to write a recursive JavaScript function that will make a deep copy of nested objects or arrays.
Deep copy with JSON.parse/stringify
4.If your data fits the specifications (see below), then JSON.stringify followed by JSON.parse will deep copy your object.
To demonstrate some reasons why this method is not generally recommended, here is an example of creating a deep copy using JSON.parse(JSON.stringify(object))