filmov
tv
Implicit Type Conversion | Python 4 You | Lecture 167
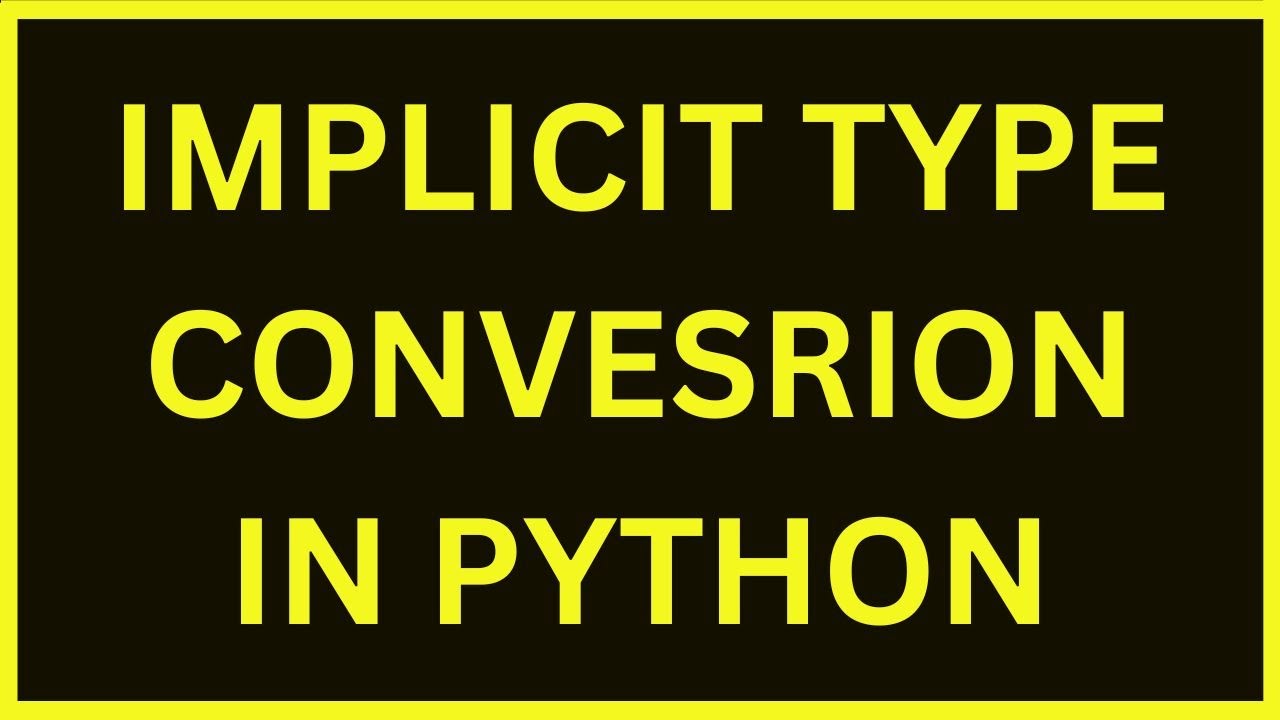
Показать описание
Implicit Type Conversion in Python: A Comprehensive Guide
In Python, data types are a fundamental concept that govern how data is stored and manipulated. Python, as a dynamically typed language, provides powerful features for type conversion, allowing developers to change the data type of variables as needed. Implicit type conversion, also known as type coercion, is a key aspect of this process. In this comprehensive guide, we will explore the concept of implicit type conversion in Python, its mechanisms, and its implications in various scenarios.
Understanding Data Types in Python
Before delving into implicit type conversion, it's essential to grasp the basic idea of data types in Python. Python has a wide variety of data types, including integers, floats, strings, lists, and more. Each data type represents a particular kind of value and has specific operations and properties associated with it. For example:
int: Represents integers, such as 1, -42, or 1000.
float: Represents floating-point numbers, like 3.14 or -0.5.
str: Represents strings, which are sequences of characters enclosed in single or double quotes, like 'hello' or "world".
bool: Represents boolean values, True or False.
list: Represents ordered collections of elements.
dict: Represents dictionaries, which store key-value pairs.
tuple: Represents immutable sequences.
In Python, you don't need to explicitly declare a variable's data type. The interpreter infers the data type based on the value assigned to the variable. This dynamic typing is one of Python's strengths, but it also introduces the need for type conversion when working with different data types.
Implicit Type Conversion (Type Coercion)
Implicit type conversion, often referred to as type coercion, is the process by which Python automatically converts data from one data type to another when required. This conversion is done implicitly, without any explicit instructions from the programmer. Python performs implicit type conversion to ensure that operations involving different data types can proceed without errors. Here's how it works:
Operations Between Different Data Types: When you perform operations (e.g., addition, subtraction, multiplication) between variables of different data types, Python will automatically convert one or both variables to a common data type.
Precedence Rules: Implicit type conversion follows a set of precedence rules to determine the resulting data type. Some data types are considered higher precedence and can coerce others to their type.
Examples of Implicit Type Conversion:
When you add an integer and a float, Python will automatically convert the integer to a float to perform the operation.
When you concatenate a string and a number, Python will convert the number to a string and then perform the concatenation.
When you compare an integer and a string using the equality operator (==), Python will not convert them, and the result will be False because they are of different data types.
Type Coercion Precedence Rules
Implicit type conversion in Python follows certain precedence rules. Some data types can coerce others, and when you perform operations between variables of different types, Python chooses the higher precedence data type for the result. Here is a simplified precedence order:
Complex numbers
Floats
Integers
Booleans
Sequences (strings, lists, and tuples)
In general, operations between more complex data types result in a higher precedence data type, ensuring that data isn't lost during conversion.
Scenarios of Implicit Type Conversion
Implicit type conversion occurs in various scenarios in Python. Some of the common situations include:
Numeric Operations: When performing arithmetic operations between different numeric data types (e.g., int and float), type coercion ensures that both operands are of the same data type.
String Concatenation: When concatenating strings with other data types, Python converts the non-string data to a string for concatenation.
Boolean Operations: Implicit conversion occurs in boolean expressions, where different data types are compared or combined.
Mixed Data Structures: When working with data structures like lists or dictionaries, implicit type conversion can occur during operations that involve different data types.
Examples of Implicit Type Conversion
Let's explore some examples to better understand how implicit type conversion works in Python.
Numeric Operations:
python
Copy code
result = 5 + 3.0
# The integer 5 is implicitly converted to a float.
# result is now 8.0 (a float).
#python4 #pythontutorial #pythonprogramming #python3 #pythonforbeginners #pythonlectures #pythonprograms #pythonlatest #rehanblogger #python4you #pythonlatestversion #pythonlatestversion Learn python3.12.0 and latest version of python3.13. If you are searching for python3.13.0 lessons, you are at the right place as this course will be very helpful for python learners or python beginners.
In Python, data types are a fundamental concept that govern how data is stored and manipulated. Python, as a dynamically typed language, provides powerful features for type conversion, allowing developers to change the data type of variables as needed. Implicit type conversion, also known as type coercion, is a key aspect of this process. In this comprehensive guide, we will explore the concept of implicit type conversion in Python, its mechanisms, and its implications in various scenarios.
Understanding Data Types in Python
Before delving into implicit type conversion, it's essential to grasp the basic idea of data types in Python. Python has a wide variety of data types, including integers, floats, strings, lists, and more. Each data type represents a particular kind of value and has specific operations and properties associated with it. For example:
int: Represents integers, such as 1, -42, or 1000.
float: Represents floating-point numbers, like 3.14 or -0.5.
str: Represents strings, which are sequences of characters enclosed in single or double quotes, like 'hello' or "world".
bool: Represents boolean values, True or False.
list: Represents ordered collections of elements.
dict: Represents dictionaries, which store key-value pairs.
tuple: Represents immutable sequences.
In Python, you don't need to explicitly declare a variable's data type. The interpreter infers the data type based on the value assigned to the variable. This dynamic typing is one of Python's strengths, but it also introduces the need for type conversion when working with different data types.
Implicit Type Conversion (Type Coercion)
Implicit type conversion, often referred to as type coercion, is the process by which Python automatically converts data from one data type to another when required. This conversion is done implicitly, without any explicit instructions from the programmer. Python performs implicit type conversion to ensure that operations involving different data types can proceed without errors. Here's how it works:
Operations Between Different Data Types: When you perform operations (e.g., addition, subtraction, multiplication) between variables of different data types, Python will automatically convert one or both variables to a common data type.
Precedence Rules: Implicit type conversion follows a set of precedence rules to determine the resulting data type. Some data types are considered higher precedence and can coerce others to their type.
Examples of Implicit Type Conversion:
When you add an integer and a float, Python will automatically convert the integer to a float to perform the operation.
When you concatenate a string and a number, Python will convert the number to a string and then perform the concatenation.
When you compare an integer and a string using the equality operator (==), Python will not convert them, and the result will be False because they are of different data types.
Type Coercion Precedence Rules
Implicit type conversion in Python follows certain precedence rules. Some data types can coerce others, and when you perform operations between variables of different types, Python chooses the higher precedence data type for the result. Here is a simplified precedence order:
Complex numbers
Floats
Integers
Booleans
Sequences (strings, lists, and tuples)
In general, operations between more complex data types result in a higher precedence data type, ensuring that data isn't lost during conversion.
Scenarios of Implicit Type Conversion
Implicit type conversion occurs in various scenarios in Python. Some of the common situations include:
Numeric Operations: When performing arithmetic operations between different numeric data types (e.g., int and float), type coercion ensures that both operands are of the same data type.
String Concatenation: When concatenating strings with other data types, Python converts the non-string data to a string for concatenation.
Boolean Operations: Implicit conversion occurs in boolean expressions, where different data types are compared or combined.
Mixed Data Structures: When working with data structures like lists or dictionaries, implicit type conversion can occur during operations that involve different data types.
Examples of Implicit Type Conversion
Let's explore some examples to better understand how implicit type conversion works in Python.
Numeric Operations:
python
Copy code
result = 5 + 3.0
# The integer 5 is implicitly converted to a float.
# result is now 8.0 (a float).
#python4 #pythontutorial #pythonprogramming #python3 #pythonforbeginners #pythonlectures #pythonprograms #pythonlatest #rehanblogger #python4you #pythonlatestversion #pythonlatestversion Learn python3.12.0 and latest version of python3.13. If you are searching for python3.13.0 lessons, you are at the right place as this course will be very helpful for python learners or python beginners.