filmov
tv
Comparing `printf` and `cout` in C++
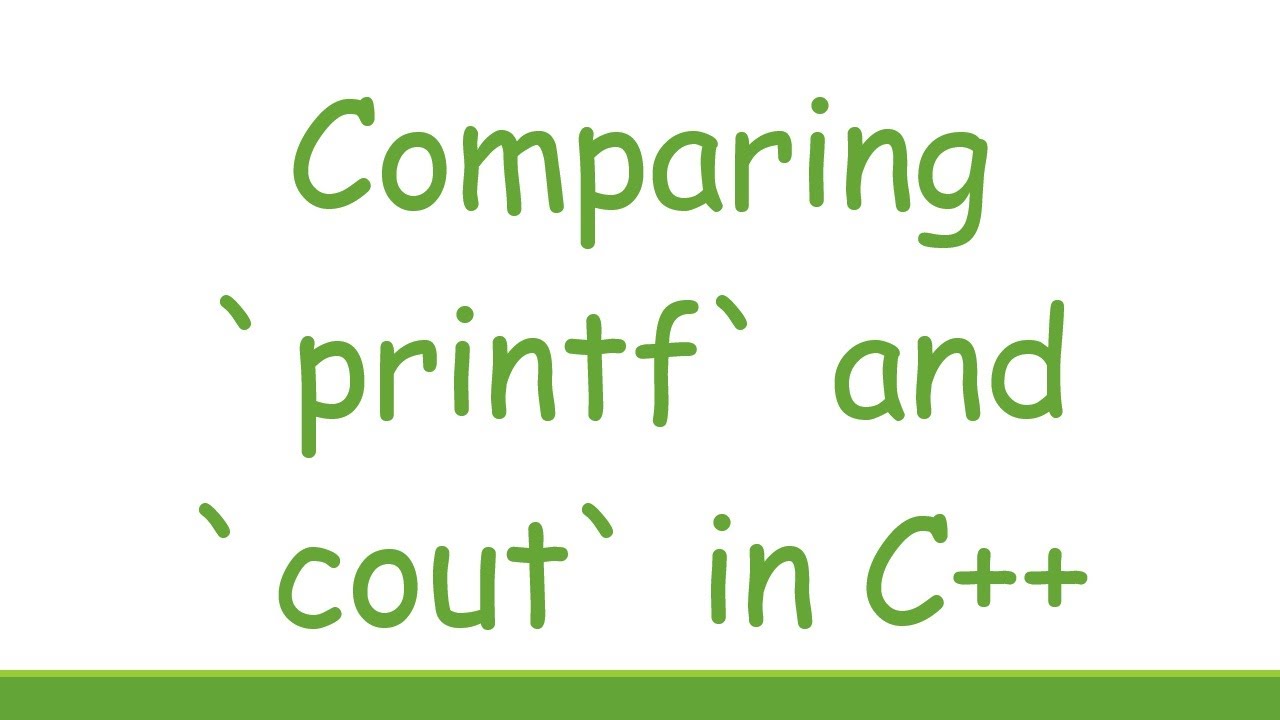
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Explore the differences, advantages, and disadvantages of using `printf` and `cout` for output in C++ programming, providing insights into their syntax, performance, and use cases.
---
When it comes to output in C++, two primary options are available: printf and cout. Each has its own set of characteristics, advantages, and potential drawbacks. Understanding these differences is crucial for making informed decisions based on the specific needs of your project.
Syntax and Ease of Use
printf
printf is a function inherited from the C programming language. It uses format specifiers to control the output format, which can be less intuitive for those unfamiliar with the syntax.
Example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, %s is a placeholder for a string, and %d is a placeholder for an integer. The variables name and numMessages are provided as arguments to replace these placeholders.
cout
cout is part of the C++ Standard Library and uses the insertion operator << for output. This approach is generally more readable and easier to use, especially for those new to programming.
Example:
[[See Video to Reveal this Text or Code Snippet]]
The syntax resembles natural language, which can make the code more readable.
Type Safety
One of the key advantages of cout over printf is type safety. cout handles type conversions automatically, reducing the risk of errors related to mismatched format specifiers and data types.
Example of a potential error with printf:
[[See Video to Reveal this Text or Code Snippet]]
This code compiles but results in undefined behavior at runtime.
With cout, such mistakes are less likely:
[[See Video to Reveal this Text or Code Snippet]]
Performance
Performance can be a consideration in high-performance applications. Generally, printf can be faster than cout because it is a lower-level function with less overhead. cout performs additional type checking and manages more complex object-oriented features.
However, the difference in performance is often negligible for most applications. The readability and safety of cout make it a preferred choice unless specific performance constraints necessitate the use of printf.
Flexibility and Extensibility
printf
printf is less flexible when it comes to extending functionality for user-defined types. You would need to create custom format specifiers or perform manual conversions before using printf.
cout
cout can be easily extended for user-defined types by overloading the << operator, making it more versatile for complex applications.
Example:
[[See Video to Reveal this Text or Code Snippet]]
This code allows instances of MyClass to be output using cout.
Conclusion
Both printf and cout have their places in C++ programming. printf offers a performance edge and familiarity for those with a C background, but at the cost of readability and type safety. cout, on the other hand, provides a more modern, type-safe, and extensible approach, making it a suitable choice for most C++ applications. The choice between the two ultimately depends on the specific needs and constraints of your project.
---
Summary: Explore the differences, advantages, and disadvantages of using `printf` and `cout` for output in C++ programming, providing insights into their syntax, performance, and use cases.
---
When it comes to output in C++, two primary options are available: printf and cout. Each has its own set of characteristics, advantages, and potential drawbacks. Understanding these differences is crucial for making informed decisions based on the specific needs of your project.
Syntax and Ease of Use
printf
printf is a function inherited from the C programming language. It uses format specifiers to control the output format, which can be less intuitive for those unfamiliar with the syntax.
Example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, %s is a placeholder for a string, and %d is a placeholder for an integer. The variables name and numMessages are provided as arguments to replace these placeholders.
cout
cout is part of the C++ Standard Library and uses the insertion operator << for output. This approach is generally more readable and easier to use, especially for those new to programming.
Example:
[[See Video to Reveal this Text or Code Snippet]]
The syntax resembles natural language, which can make the code more readable.
Type Safety
One of the key advantages of cout over printf is type safety. cout handles type conversions automatically, reducing the risk of errors related to mismatched format specifiers and data types.
Example of a potential error with printf:
[[See Video to Reveal this Text or Code Snippet]]
This code compiles but results in undefined behavior at runtime.
With cout, such mistakes are less likely:
[[See Video to Reveal this Text or Code Snippet]]
Performance
Performance can be a consideration in high-performance applications. Generally, printf can be faster than cout because it is a lower-level function with less overhead. cout performs additional type checking and manages more complex object-oriented features.
However, the difference in performance is often negligible for most applications. The readability and safety of cout make it a preferred choice unless specific performance constraints necessitate the use of printf.
Flexibility and Extensibility
printf
printf is less flexible when it comes to extending functionality for user-defined types. You would need to create custom format specifiers or perform manual conversions before using printf.
cout
cout can be easily extended for user-defined types by overloading the << operator, making it more versatile for complex applications.
Example:
[[See Video to Reveal this Text or Code Snippet]]
This code allows instances of MyClass to be output using cout.
Conclusion
Both printf and cout have their places in C++ programming. printf offers a performance edge and familiarity for those with a C background, but at the cost of readability and type safety. cout, on the other hand, provides a more modern, type-safe, and extensible approach, making it a suitable choice for most C++ applications. The choice between the two ultimately depends on the specific needs and constraints of your project.