filmov
tv
How To Make A Simple Function Decorator (Python Recipes)
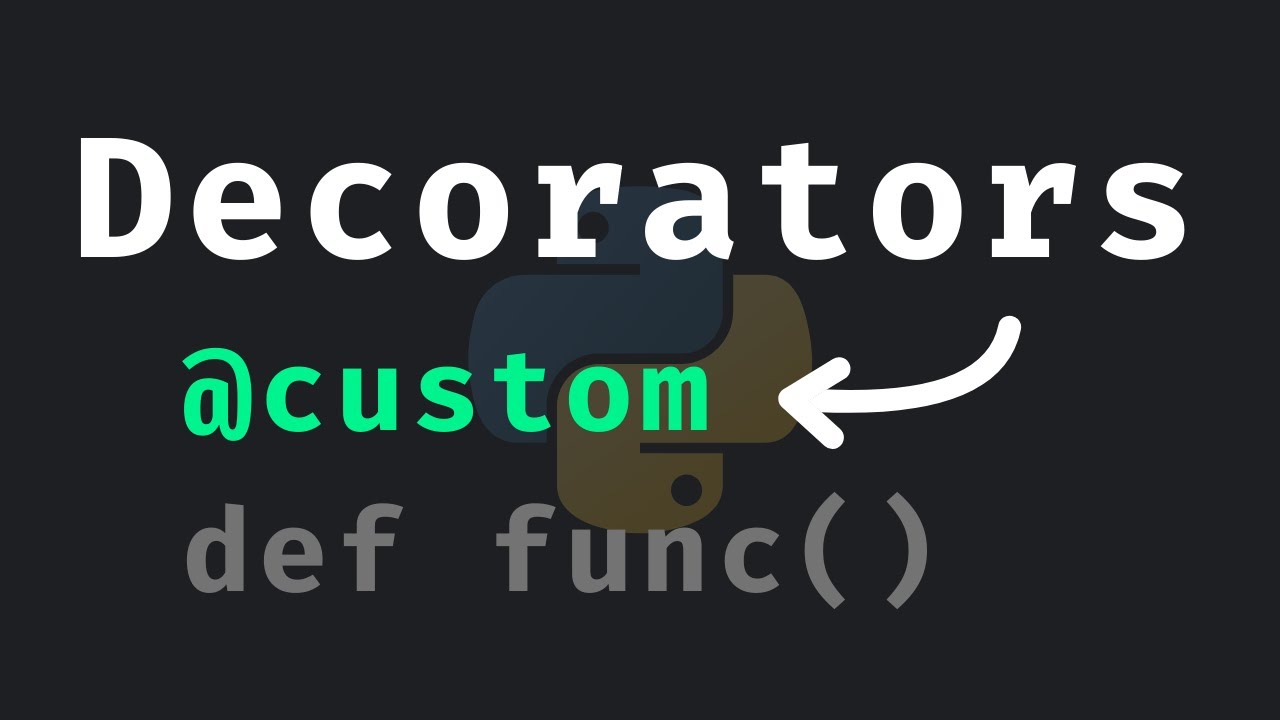
Показать описание
In this video I will be showing you how you can create a simple function decorator in Python! Decorators are some of Python's best sugar syntax which make applying extra functionality much more convenient.
▶ Become job-ready with Python:
▶ Follow me on Instagram:
▶ Become job-ready with Python:
▶ Follow me on Instagram:
how i make a simple 3 egg omelette
NO GLUE SLIME Recipes That ACTUALLY WORK! 😱🤫 *How to Make Slime WITHOUT Glue and Activator DIY*...
How To Make Simple Pencil Welding Machine At Home for soldering | practical invention
Let’s Make a Simple Miso Soup
How to Make Simple Syrup | Patrón Tequila
How To Make Simple Pencil Welding Machine At Home With Blade | practical invention
Make a Cute and Simple Sanrio Bracelet!#shorts
Laziest Way to Make Money Online For Beginners ($100+/Day)
How to make simple easy block ✅✅⭐
How To Make Stunningly Simple Macarons
How to make a simple welding machine from SPARK PLUG at home! Genius invention
How to make a simple paper plane || Paper plane 286
How to Make Simple Syrup | Black Tie Kitchen
How to Make a Simple Pop Pop Boat
How to make lavender simple syrup #coffee
Perfect Pancake/ Fluffy Pancake Recipe #shorts
How to make a simple flying toy |
DIY how to make small dairy ll mini note book ll cute notebook ll 😘🥰
WATER AND SUGAR SLIME/HOW TO MAKE WATER AND SUGAR SLIME WITHOUT GLUE BORAX/SLIME MAKING AT HOME EASY
Easy Coffee Cake Recipe, Simple and Quick - You will make this every day! Coffee and Walnut cake
No Borax No Glue Slime/How to make Slime at home/DIY Fluffy Slime/Flour Slime/Slime making #slime
DIY crafts - How to Make Simple Easy Bow/ Ribbon Hair Bow Tutorial // DIY beauty and easy
How to Make Simple Sautéed Spinach
No glue, No borax, Toothpaste slime | Diy toothpaste slime | How to make slime with toothpaste
Комментарии