filmov
tv
Resolving the AttributeError in PySpark RDDs Involving Numpy Subclasses
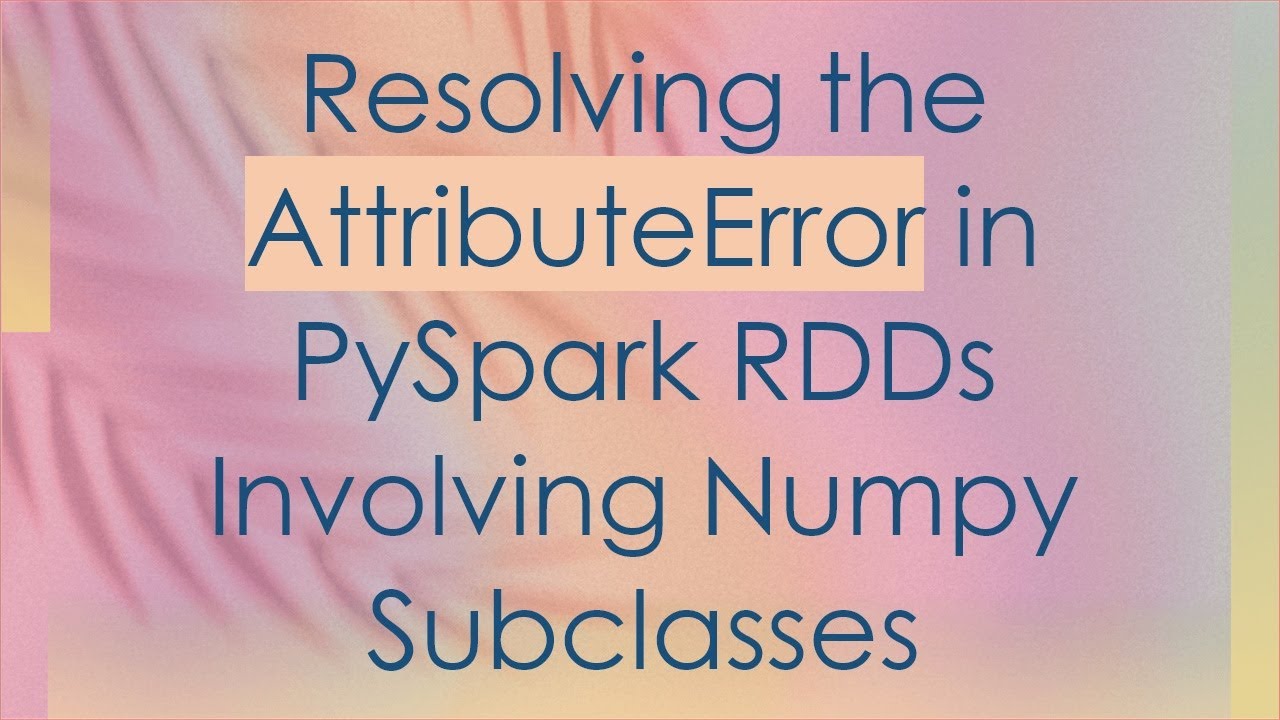
Показать описание
Discover how to effectively manage attributes in Numpy subclasses when working with PySpark RDDs and prevent unexpected behaviors.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: pyspark RDDs strip attributes of numpy subclasses
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting Attribute Errors in PySpark RDDs with Numpy Subclasses
When working with PySpark and Numpy, you might encounter an unexpected behavior where custom attributes of Numpy subclasses seem to vanish during processing in RDDs (Resilient Distributed Datasets). This can lead to frustrating AttributeError messages, which can significantly slow down your development process. In this post, we'll explore a specific problem involving a Numpy ndarray subclass and outline a solution to ensure that these custom attributes are preserved when operating on RDDs.
The Problem
Understanding the Issue
[[See Video to Reveal this Text or Code Snippet]]
When you call a function that constructs this subclass directly, it works as expected:
[[See Video to Reveal this Text or Code Snippet]]
However, when this function is called within a PySpark RDD map transformation, you encounter problems. The output shows that while the instances of MyArray are being created, the extra attribute is stripped away:
[[See Video to Reveal this Text or Code Snippet]]
Diagnosing the Problem
The Solution
Implementing Serialization Methods
To resolve this, you need to implement custom __reduce__ and __setstate__ methods in your subclass to manage the serialization of the extra attribute properly. By adding the following methods to your MyArray class, you can ensure that the extra attribute is preserved:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
__reduce__: This method is responsible for defining how the object should be serialized. By extending this, it's possible to include the extra attribute when the object is prepared for serialization.
__setstate__: This method sets the state of the object when it is deserialized. Here, we ensure that extra is re-assigned after reconstructing the base class attributes.
Final Implementation
Putting it all together, your modified MyArray class should look like this:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By implementing these custom serialization methods, you can maintain the integrity of your Numpy subclass attributes when using PySpark RDDs. This solution simplifies handling the subclass while leveraging the power of distributed computing. Don't let serialization issues impede your progress—adhere to the solutions outlined above for smoother data processing.
With these strategies, you can enhance your coding experience in the PySpark environment and focus more on building your applications instead of wrestling with unexpected errors.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: pyspark RDDs strip attributes of numpy subclasses
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting Attribute Errors in PySpark RDDs with Numpy Subclasses
When working with PySpark and Numpy, you might encounter an unexpected behavior where custom attributes of Numpy subclasses seem to vanish during processing in RDDs (Resilient Distributed Datasets). This can lead to frustrating AttributeError messages, which can significantly slow down your development process. In this post, we'll explore a specific problem involving a Numpy ndarray subclass and outline a solution to ensure that these custom attributes are preserved when operating on RDDs.
The Problem
Understanding the Issue
[[See Video to Reveal this Text or Code Snippet]]
When you call a function that constructs this subclass directly, it works as expected:
[[See Video to Reveal this Text or Code Snippet]]
However, when this function is called within a PySpark RDD map transformation, you encounter problems. The output shows that while the instances of MyArray are being created, the extra attribute is stripped away:
[[See Video to Reveal this Text or Code Snippet]]
Diagnosing the Problem
The Solution
Implementing Serialization Methods
To resolve this, you need to implement custom __reduce__ and __setstate__ methods in your subclass to manage the serialization of the extra attribute properly. By adding the following methods to your MyArray class, you can ensure that the extra attribute is preserved:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
__reduce__: This method is responsible for defining how the object should be serialized. By extending this, it's possible to include the extra attribute when the object is prepared for serialization.
__setstate__: This method sets the state of the object when it is deserialized. Here, we ensure that extra is re-assigned after reconstructing the base class attributes.
Final Implementation
Putting it all together, your modified MyArray class should look like this:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By implementing these custom serialization methods, you can maintain the integrity of your Numpy subclass attributes when using PySpark RDDs. This solution simplifies handling the subclass while leveraging the power of distributed computing. Don't let serialization issues impede your progress—adhere to the solutions outlined above for smoother data processing.
With these strategies, you can enhance your coding experience in the PySpark environment and focus more on building your applications instead of wrestling with unexpected errors.