filmov
tv
DP 45. Longest String Chain | Longest Increasing Subsequence | LIS
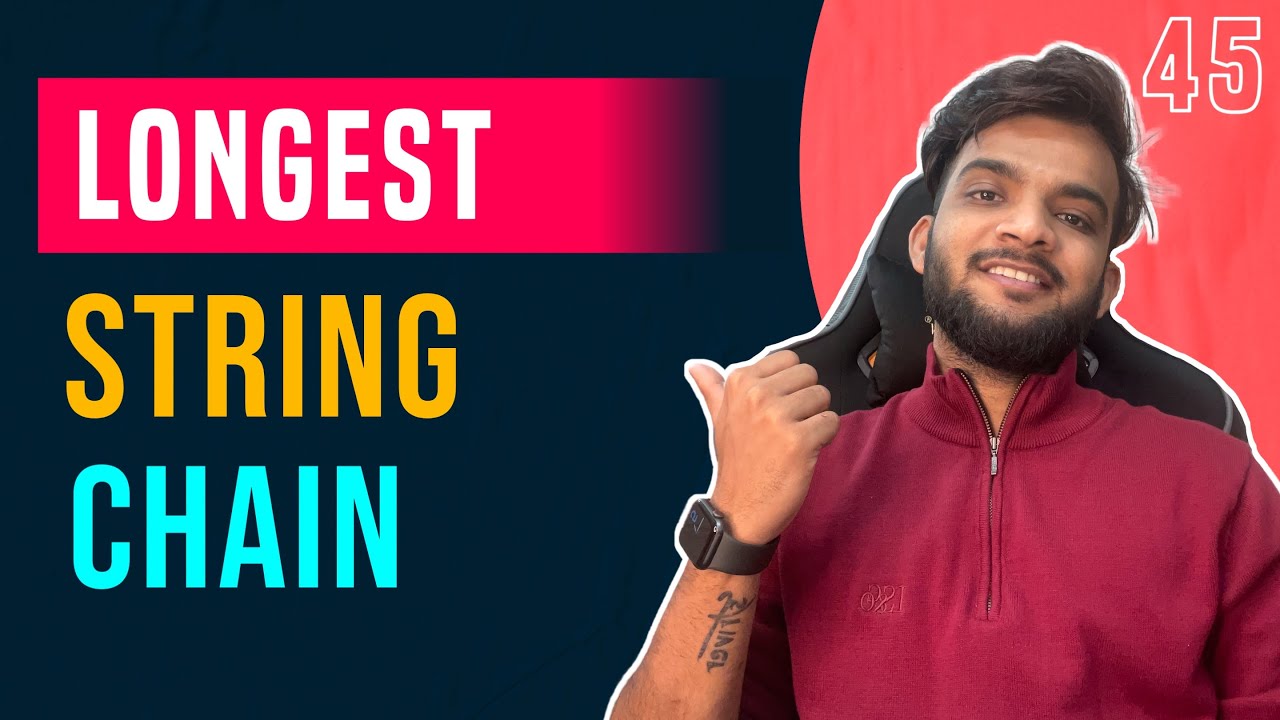
Показать описание
Please watch the video at 1.25x for a better experience.
a
In this video, we solve the Longest String Chain, prior to this please solve dp 41 and dp 42.
DP 45. Longest String Chain | Longest Increasing Subsequence | LIS
Longest String Chain - Leetcode 1048 - Python
Longest String Chain | Same as LIS | FULL INTUITION | DP Concepts & Qns - 14 | Leetcode-1048
Leetcode - Longest String Chain (Python)
Longest String Chain with Dynamic Programming - LeetCode #1048 | Python, JavaScript, Java, C++
Leetcode 1048. Longest String Chain
Longest String Chain | Live Coding with Explanation | Leetcode - 1048
Leetcode 1048. Longest String Chain - Dynamic Programming
Unlocking LeetCode 1048. Longest String Chain: Dive Deep with Dynamic Programming | Python
1048. Longest String Chain - Day 23/30 Leetcode September Challenge
Longest String Chain | Leetcode 1048 | Dynamic Programming
Coding Interview Problem - Longest String Chain
Longest String Chain | LeetCode 1048 | Coders Camp
Leetcode 1048 Longest String Chain | DP | Checkout Coding Decoded SDE Sheet | Live coding session
How to Solve the Longest String Chain Problem with Golang
1048 - Longest String Chain || 23 September 2023 || Leetcode Daily Challenge
1048. Longest String Chain | Leetcode Dynamic Programming | LEETCODE
Longest word chain | LEETCODE 1048 | Dynamic Programming
Собеседование в IT | LeetCode | 1048. Longest String Chain
Longest String Chain | Leetcode 1048 | Live coding session | Google | Facebook
LeetCode 1048. Longest String Chain
Leetcode 1048 - Longest String Chain
Разбор задачи 1048 leetcode.com Longest String Chain
GOOGLE CODING INTERVIEW QUESTION - Longest String Chain | Abinash Biswal
Комментарии