filmov
tv
Algorithms: Quicksort
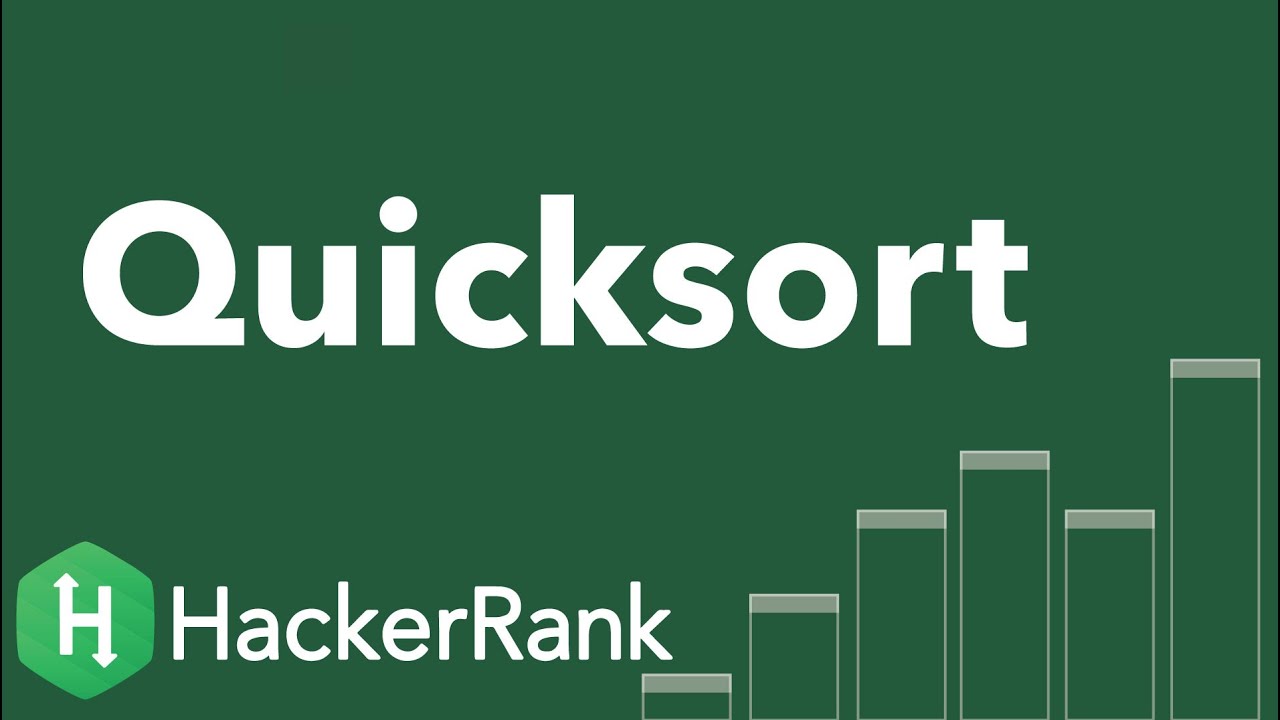
Показать описание
Learn the basics of quicksort. This video is a part of HackerRank's Cracking The Coding Interview Tutorial with Gayle Laakmann McDowell.
Quick sort in 4 minutes
Quick Sort Algorithm
2.8.1 QuickSort Algorithm
Quicksort Algorithmus / Quick Sort Sortierverfahren mit Beispiel (deutsch)
Learn Quick Sort in 13 minutes ⚡
Quick Sort Algorithm Explained!
Quick Sort - Computerphile
Quicksort algorithm
Algorithm ⚡💻 #QuickSort #LearnCoding #SortingAlgorithm #CodeBasics #ProgrammingLife #TechTips'...
Quicksort Sort Algorithm in Java - Full Tutorial With Source
Quicksort: Partitioning an array
Algorithms: Quicksort
Quicksort Algorithmus [mit Animation, Deutsch]
Quuck Sort Algorithm in Data Structures #quicksort #sorting #algorithm #datastructures
L-3.1: How Quick Sort Works | Performance of Quick Sort with Example | Divide and Conquer
Quick Sort (LR pointers)
A Complete Overview of Quicksort (Data Structures & Algorithms #11)
7.6 Quick Sort in Data Structure | Sorting Algorithm | DSA Full Course
Quicksort vs Mergesort in 35 Seconds
Quicksort Algorithm: A Step-by-Step Visualization
Quick Sort #animation
Quicksort
Quicksort In Python Explained (With Example And Code)
Quick Sort | Quick Sort algorithm #algorithm #quicksort #QuickSort #algorithms #quicksortalgorithm
Комментарии