filmov
tv
Longest Increasing Subsequence in nlogn time
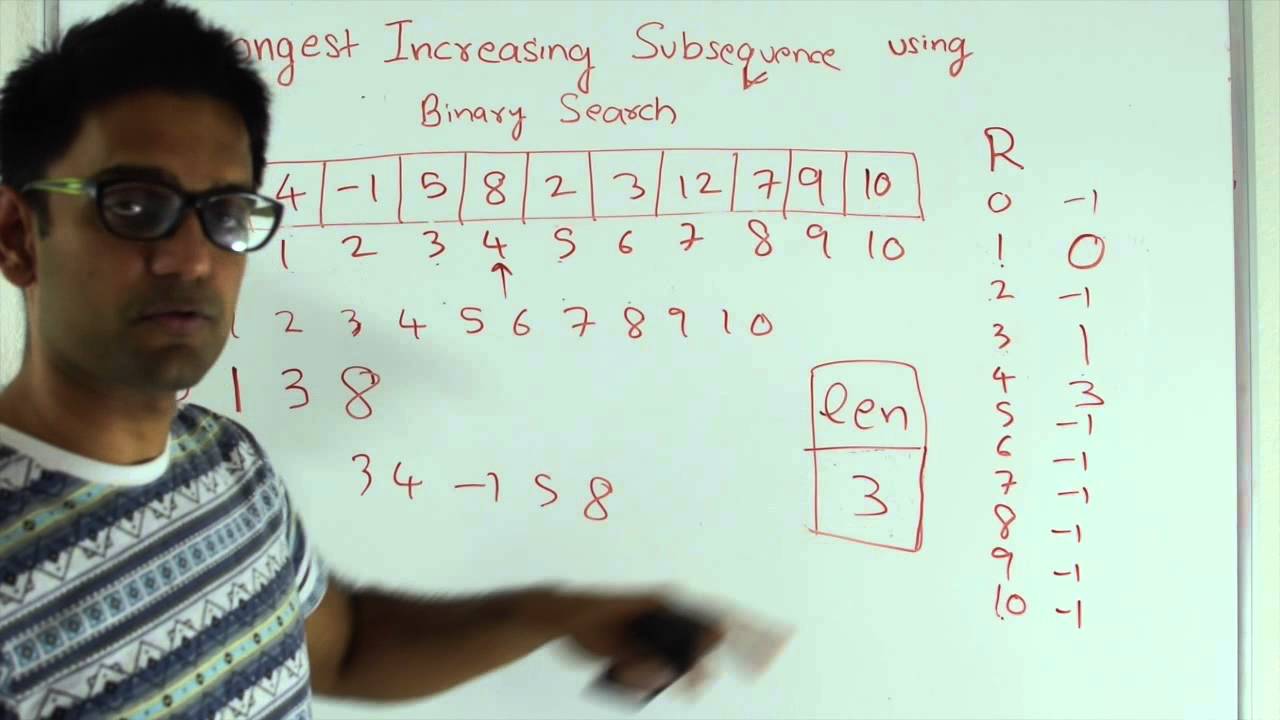
Показать описание
Find the longest increasing subsequence in nlogn time.
Longest Increasing Subsequence in nlogn time
Longest Increasing Subsequence O(n log n) dynamic programming Java source code
DP 43. Longest Increasing Subsequence | Binary Search | Intuition
LeetCode 300. Longest Increasing Subsequence - O(n log n)
Longest Increasing Subsequence (LIS) - O(NlogN) | Searching - Animation - Solutions - inDepth
Longest Increasing Subsequence NlogN approach
Longest Increasing Subsequence NlogN | Leetcode #300 | LIS
Day 322 - Teaching Kids Programming - Greedy to Find Longest Increasing Subsequence in O(NLogN)
LIS in O(nlogn) with printing solution | Day 4 Part 1 | Dynamic Programming workshop | Vivek Gupta
Longest Increasing Subsequence in O(NlogN)
Patience Sort | Longest Increasing Subsequence | O(nlogn) time | Stable Sort | Leetcode 300
Longest Increasing Subsequence - nlogn - Binary Search [LeetCode 300]
Segment tree on values; Longest Increasing Subsequence (LIS) in O(nlogn)
Longest Increasing Subsequence in O(nlogn) | DP + Coordinate Compression Approach | Live Coding C++
Longest Increasing Subsequence - Dynamic Programming - Leetcode 300
Patience Sort | Longest Increasing Subsequence in O(n log n) Time complexity | Coders Camp
Longest Increasing Subsequence | O(NLOGN) | DP-17 | DP Is Easy
Length of Longest Increasing Subsequence - O(nlogn)
Longest Increasing Subsequence in C++ | NlogN vs N^2 | Classic Dynamic Programming
Longest Increasing Subsequence | O(NlogN) | Binary Search | DP | Interview Question(LeetCode #300)
Longest Increasing Subsequence in NlogN time Complexity | DP 42 | Placement Preparation Series |
Longest Increasing Subsequence
Longest Increasing Subsequence O(nlogn)
Longest Increasing Subsequence || O(nlogn) || Binary Search (Pattern 1) || DSAP 20
Комментарии