filmov
tv
How to Properly Map a Component with an Array Parameter in React Typescript
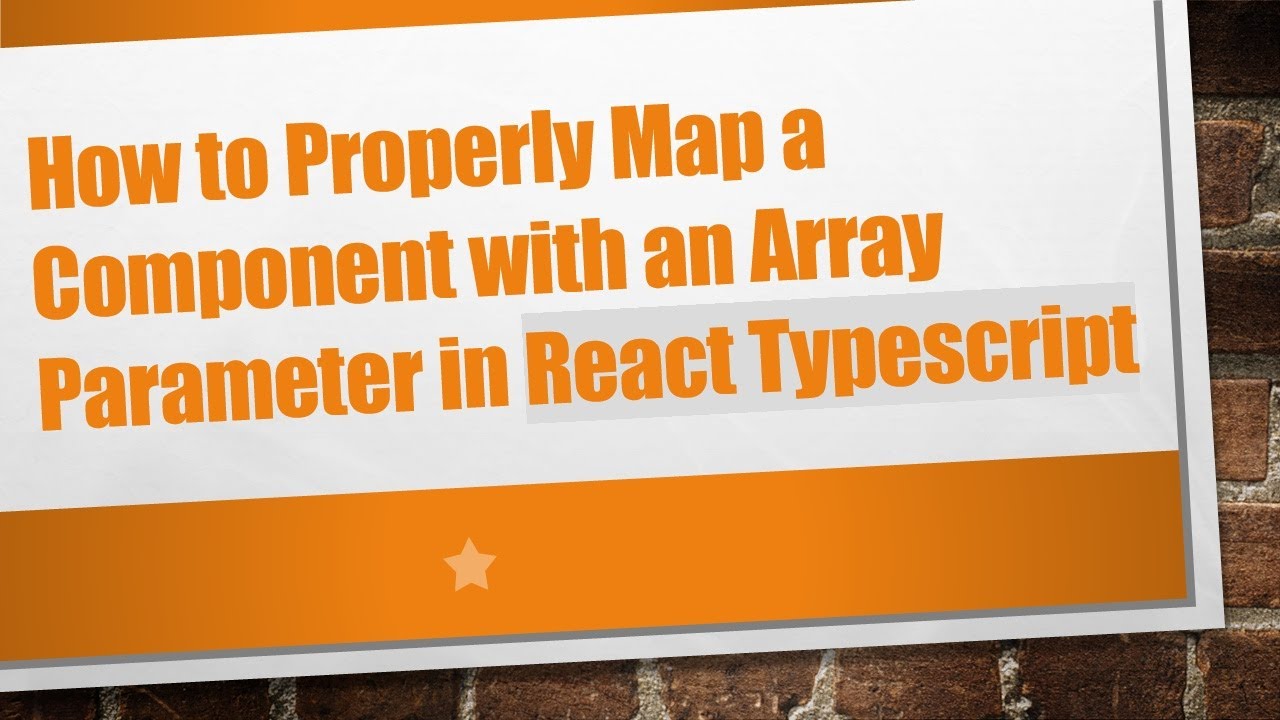
Показать описание
Learn how to map arrays in React with TypeScript effectively, avoiding common type errors and ensuring smooth object handling.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How do I map a component that has an array parameter in React Typescript?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the Problem of Mapping Components with Array Parameters in React Typescript
When working with React and TypeScript, Type Safety is a key concern, especially when dealing with arrays that contain complex objects. One common question developers face is, “How do I map a component that has an array parameter in React Typescript?”
In this guide, we'll explore this issue and walk through a clear solution, ensuring that your code remains type-safe and effective.
Understanding the Problem
The user-inquired situation involves mapping over an array of player objects retrieved from a gamesPlayed variable, which consists of multiple properties, including an array of players.
The Initial Code Snippet
Here’s the relevant section of the initial code:
[[See Video to Reveal this Text or Code Snippet]]
Identifying the Issues
Type Definition: The error regarding score arises because TypeScript does not have sufficient information about what properties the objects in the array will have.
The Solution
To remedy this, let’s break down the solution into simple, clear steps.
Step 1: Define the Player Interface
Firstly, we need to declare a type to represent our player object. This ensures that TypeScript understands the data structure being passed.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Correct Map Syntax
Now, let’s adjust the mapping function by using the correct syntax:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes
Single Parameter in Map: Here, we pass each player directly to the mapping function, eliminating the second argument.
Type-Safe Player Reference: By defining player using the Player interface, TypeScript understands that player has a score property available.
Conclusion
Mapping components with arrays in React TypeScript does not have to be challenging. By correctly defining your object types and using the proper mapping syntax, you ensure that your code remains type-safe and free of errors.
Wrap Up
Now you should be equipped to handle similar situations in your React TypeScript applications confidently! Remember that leveraging TypeScript’s strong typing can significantly enhance the maintainability and reliability of your code.
Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How do I map a component that has an array parameter in React Typescript?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the Problem of Mapping Components with Array Parameters in React Typescript
When working with React and TypeScript, Type Safety is a key concern, especially when dealing with arrays that contain complex objects. One common question developers face is, “How do I map a component that has an array parameter in React Typescript?”
In this guide, we'll explore this issue and walk through a clear solution, ensuring that your code remains type-safe and effective.
Understanding the Problem
The user-inquired situation involves mapping over an array of player objects retrieved from a gamesPlayed variable, which consists of multiple properties, including an array of players.
The Initial Code Snippet
Here’s the relevant section of the initial code:
[[See Video to Reveal this Text or Code Snippet]]
Identifying the Issues
Type Definition: The error regarding score arises because TypeScript does not have sufficient information about what properties the objects in the array will have.
The Solution
To remedy this, let’s break down the solution into simple, clear steps.
Step 1: Define the Player Interface
Firstly, we need to declare a type to represent our player object. This ensures that TypeScript understands the data structure being passed.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Correct Map Syntax
Now, let’s adjust the mapping function by using the correct syntax:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes
Single Parameter in Map: Here, we pass each player directly to the mapping function, eliminating the second argument.
Type-Safe Player Reference: By defining player using the Player interface, TypeScript understands that player has a score property available.
Conclusion
Mapping components with arrays in React TypeScript does not have to be challenging. By correctly defining your object types and using the proper mapping syntax, you ensure that your code remains type-safe and free of errors.
Wrap Up
Now you should be equipped to handle similar situations in your React TypeScript applications confidently! Remember that leveraging TypeScript’s strong typing can significantly enhance the maintainability and reliability of your code.
Happy coding!