filmov
tv
41 Universal Functions and Other Array Manipulations
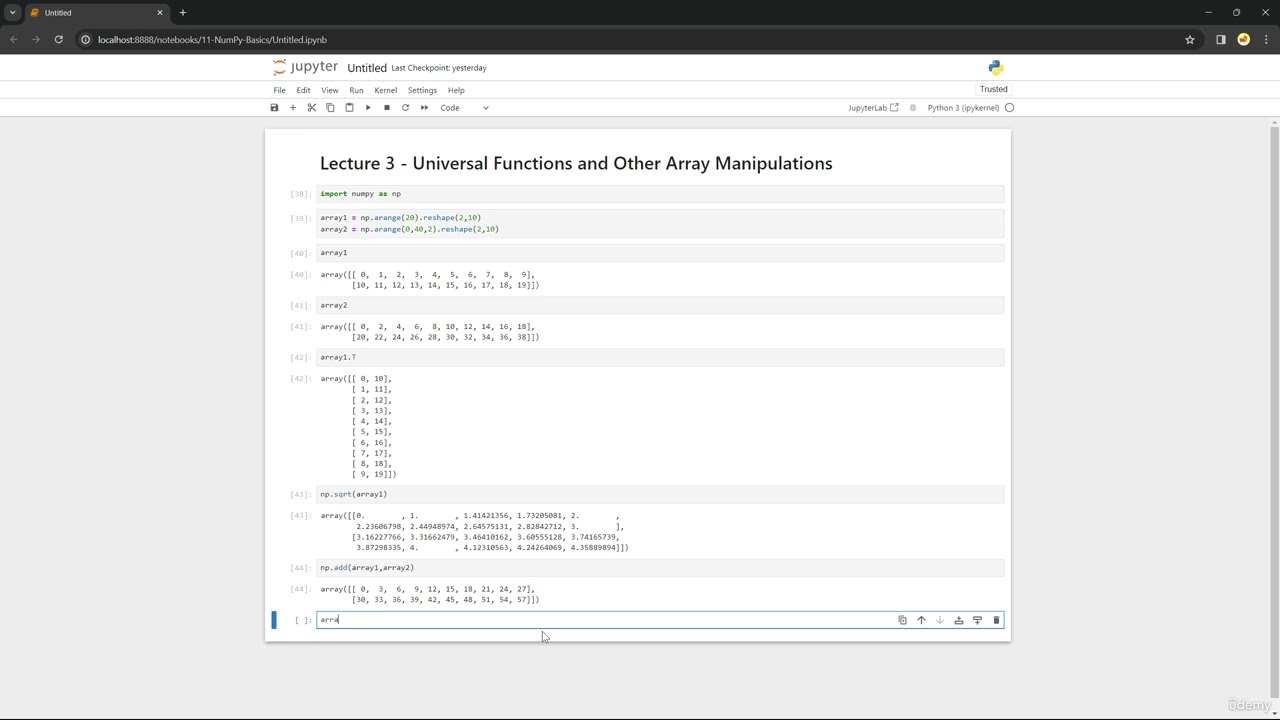
Показать описание
### Universal Functions (ufuncs) and Other Array Manipulations in NumPy
**Universal functions (ufuncs)** are a central feature of NumPy that allow you to perform element-wise operations on arrays efficiently. These functions work element by element, applying the operation to each element in the array without the need for explicit loops.
In addition to ufuncs, NumPy provides various tools for reshaping, concatenating, and performing other array manipulations.
### 1. **Universal Functions (ufuncs)**
Ufuncs are functions that operate on **ndarrays** element-wise. They include basic operations like addition, multiplication, and trigonometric functions, but also more complex operations like logarithms, exponents, and rounding functions.
#### Common Examples of Ufuncs:
- **Arithmetic Operations**: `+`, `-`, `*`, `/`, `//`, `**` (exponentiation)
Example:
```python
import numpy as np
# Square root (ufunc)
print(sqrt_arr) # Output: [1. 2. 3. 4.]
# Exponentiation (ufunc)
print(exp_arr) # Output: [2.71828183e+00 5.45981500e+01 8.10308393e+03 8.88611052e+06]
```
Ufuncs are highly optimized for performance, and their operations are executed much faster than using Python loops, especially on large arrays.
### 2. **Other Array Manipulations**
NumPy provides a wide range of functions to manipulate and reshape arrays. Here are a few common ones:
#### a. **Reshaping Arrays**
```python
print(reshaped_arr)
# Output:
# [[1 2 3]
# [4 5 6]]
```
#### b. **Flattening Arrays**
To convert a multi-dimensional array into a 1D array, you can use `.flatten()` or `.ravel()`.
```python
print(flattened_arr) # Output: [1 2 3 4 5 6]
```
#### c. **Concatenating and Stacking Arrays**
```python
# Concatenate two arrays along an axis (default is axis 0)
print(concatenated_arr) # Output: [1 2 3 4 5 6]
# Stack arrays vertically (row-wise)
print(stacked_arr_v)
# Output:
# [[1 2 3]
# [4 5 6]]
# Stack arrays horizontally (column-wise)
print(stacked_arr_h) # Output: [1 2 3 4 5 6]
```
#### d. **Element-wise Comparisons**
You can compare elements in NumPy arrays using relational operators, which return a boolean array.
```python
# Compare if elements are greater than 2
comparison = arr 2
print(comparison) # Output: [False False True True True]
```
#### e. **Broadcasting**
Broadcasting allows NumPy to perform arithmetic operations on arrays of different shapes. For example, you can add a scalar to an array, or perform operations between arrays with different shapes, as long as they are compatible.
```python
result = arr + 5
print(result) # Output: [6 7 8]
```
Similarly, arrays with different dimensions can be broadcast together if their shapes are compatible:
```python
result = arr2d + arr1d
print(result)
# Output:
# [[2 2 2]
# [5 5 5]]
```
### Conclusion:
- **Universal Functions (ufuncs)** are optimized functions for performing element-wise operations on NumPy arrays, which makes them highly efficient and concise.
- **Array Manipulations** in NumPy include reshaping, flattening, stacking, concatenating, and broadcasting, allowing you to transform and combine arrays in various ways to suit your needs.
These features make NumPy extremely versatile for numerical computing, data analysis, and scientific computing.
**Universal functions (ufuncs)** are a central feature of NumPy that allow you to perform element-wise operations on arrays efficiently. These functions work element by element, applying the operation to each element in the array without the need for explicit loops.
In addition to ufuncs, NumPy provides various tools for reshaping, concatenating, and performing other array manipulations.
### 1. **Universal Functions (ufuncs)**
Ufuncs are functions that operate on **ndarrays** element-wise. They include basic operations like addition, multiplication, and trigonometric functions, but also more complex operations like logarithms, exponents, and rounding functions.
#### Common Examples of Ufuncs:
- **Arithmetic Operations**: `+`, `-`, `*`, `/`, `//`, `**` (exponentiation)
Example:
```python
import numpy as np
# Square root (ufunc)
print(sqrt_arr) # Output: [1. 2. 3. 4.]
# Exponentiation (ufunc)
print(exp_arr) # Output: [2.71828183e+00 5.45981500e+01 8.10308393e+03 8.88611052e+06]
```
Ufuncs are highly optimized for performance, and their operations are executed much faster than using Python loops, especially on large arrays.
### 2. **Other Array Manipulations**
NumPy provides a wide range of functions to manipulate and reshape arrays. Here are a few common ones:
#### a. **Reshaping Arrays**
```python
print(reshaped_arr)
# Output:
# [[1 2 3]
# [4 5 6]]
```
#### b. **Flattening Arrays**
To convert a multi-dimensional array into a 1D array, you can use `.flatten()` or `.ravel()`.
```python
print(flattened_arr) # Output: [1 2 3 4 5 6]
```
#### c. **Concatenating and Stacking Arrays**
```python
# Concatenate two arrays along an axis (default is axis 0)
print(concatenated_arr) # Output: [1 2 3 4 5 6]
# Stack arrays vertically (row-wise)
print(stacked_arr_v)
# Output:
# [[1 2 3]
# [4 5 6]]
# Stack arrays horizontally (column-wise)
print(stacked_arr_h) # Output: [1 2 3 4 5 6]
```
#### d. **Element-wise Comparisons**
You can compare elements in NumPy arrays using relational operators, which return a boolean array.
```python
# Compare if elements are greater than 2
comparison = arr 2
print(comparison) # Output: [False False True True True]
```
#### e. **Broadcasting**
Broadcasting allows NumPy to perform arithmetic operations on arrays of different shapes. For example, you can add a scalar to an array, or perform operations between arrays with different shapes, as long as they are compatible.
```python
result = arr + 5
print(result) # Output: [6 7 8]
```
Similarly, arrays with different dimensions can be broadcast together if their shapes are compatible:
```python
result = arr2d + arr1d
print(result)
# Output:
# [[2 2 2]
# [5 5 5]]
```
### Conclusion:
- **Universal Functions (ufuncs)** are optimized functions for performing element-wise operations on NumPy arrays, which makes them highly efficient and concise.
- **Array Manipulations** in NumPy include reshaping, flattening, stacking, concatenating, and broadcasting, allowing you to transform and combine arrays in various ways to suit your needs.
These features make NumPy extremely versatile for numerical computing, data analysis, and scientific computing.