filmov
tv
How to check Palindrome Number || Basic Programming Questions Series
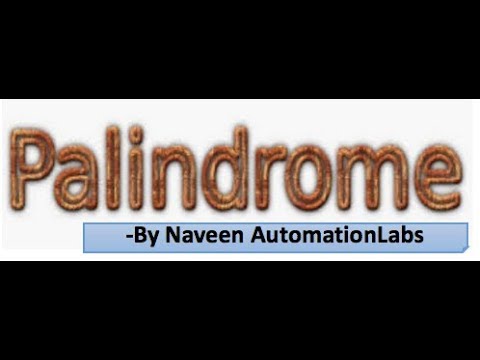
Показать описание
In this video, we will learn how to write a program to check the given number is Palindrome or not.
Learn:
1. check the given number is Palindrome or not.
2. write different test cases
~~~Subscribe to this channel, and press bell icon to get some interesting videos on Selenium and Automation:
Follow me on my Facebook Page:
Let's join our Automation community for some amazing knowledge sharing and group discussion on Telegram:
WebServices API Automation Tutorials:
Follow me on my Facebook Page:
Let's join our Automation community for some amazing knowledge sharing and group discussion on Telegram:
Paid courses (Recorded) videos:
📗 Get My Paid Courses at
Paid courses (Recorded) videos:
-------------------------------
✔️SOCIAL NETWORKS
--------------------------------
Support My Channel✔️Or Buy Me A Coffee
--------------------------------
✔️Thanks for watching!
देखने के लिए धन्यवाद
Благодаря за гледането
感谢您观看
Merci d'avoir regardé
Grazie per la visione
Gracias por ver
شكرا للمشاهدة
Learn:
1. check the given number is Palindrome or not.
2. write different test cases
~~~Subscribe to this channel, and press bell icon to get some interesting videos on Selenium and Automation:
Follow me on my Facebook Page:
Let's join our Automation community for some amazing knowledge sharing and group discussion on Telegram:
WebServices API Automation Tutorials:
Follow me on my Facebook Page:
Let's join our Automation community for some amazing knowledge sharing and group discussion on Telegram:
Paid courses (Recorded) videos:
📗 Get My Paid Courses at
Paid courses (Recorded) videos:
-------------------------------
✔️SOCIAL NETWORKS
--------------------------------
Support My Channel✔️Or Buy Me A Coffee
--------------------------------
✔️Thanks for watching!
देखने के लिए धन्यवाद
Благодаря за гледането
感谢您观看
Merci d'avoir regardé
Grazie per la visione
Gracias por ver
شكرا للمشاهدة
Комментарии