filmov
tv
Palindrome Number - Leetcode 9 - Python
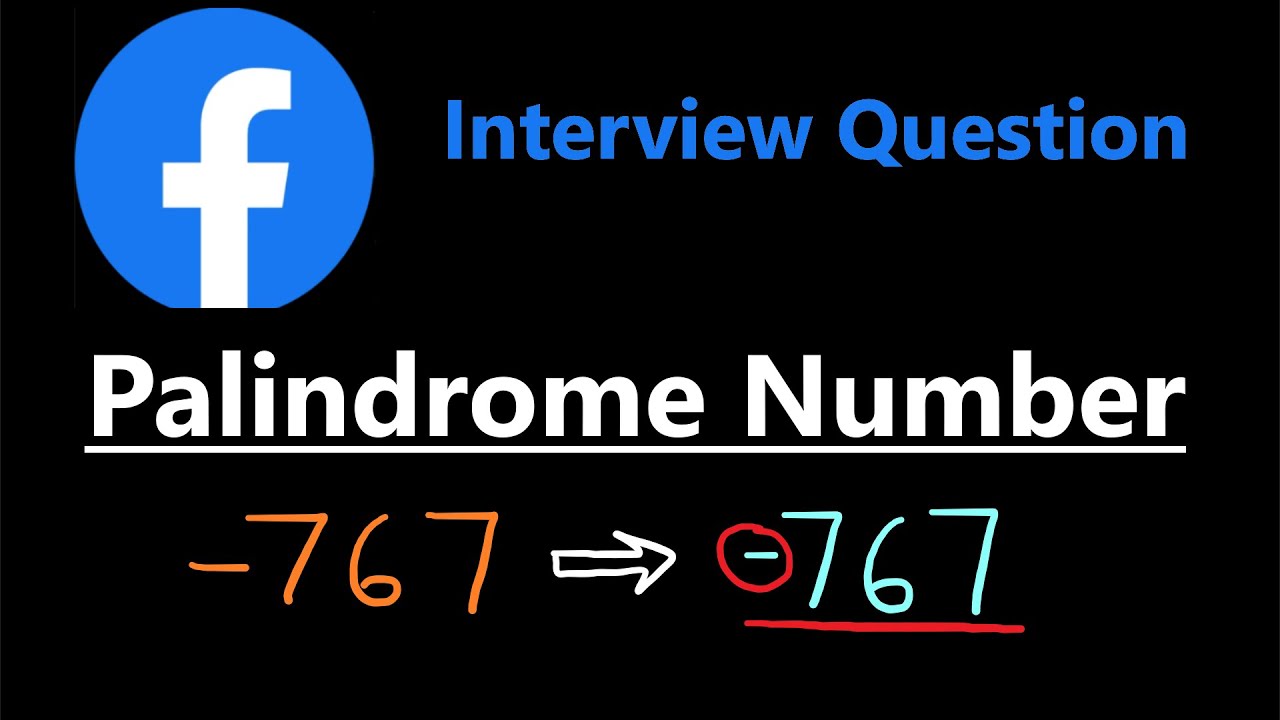
Показать описание
0:00 - Read the problem
1:10 - Drawing Explanation
8:23 - Coding Explanation
leetcode 9
#palindrome #number #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Palindrome Number - Leetcode 9 - Python
Palindrome Number | Leetcode problem 9
9. Palindrome Number - Leetcode (Python)
LeetCode #9: Palindrome Number
LeetCode Palindrome Number Solution Explained - Java
Palindrome Number - Leetcode 9 - Java
Leetcode 9. Palindrome Number [Java]
Leetcode | 9. Palindrome Number | Easy | Java Solution
LeetCode 9. Palindrome Number
Cracking LeetCode's 9 | Palindrome Number Challenge in C
Leetcode 9. Palindrome Number
LeetCode #9 - Palindrome Number
Mastering Go with LeetCode: Palindrome Number Problem | 9
Leetcode 9 Palindrome Number | JavaScript Solution | Easy Math
Python Programming Practice: LeetCode #9 -- Palindrome Number
LeetCode solutions explanation JavaScript. Problem #9 - Palindrome Number
LeetCode Problem: 9. Palindrome Number | Java Solution
Palindrome Number - Leetcode 9 - C++ Solution Explained
50% Fail this Programming Interview Question (Palindrome Numbers aka Leetcode 9)
Palindrome Number - LeetCode 9 - Coding Interview Questions
LeetCode 9 | Palindrome Number | Day 1 | 100_Days_LeetCode_Challenge | Master DSA with edSlash
Leetcode 9 Palindrome Number - Python Solution
LeetCode in C# | 9. Palindrome Number
Palindrome Number - Leetcode 9
Комментарии