filmov
tv
Google Just Hit an Absolute Low - Easy Interview Question - Check if Sentence is Pangram - 1832
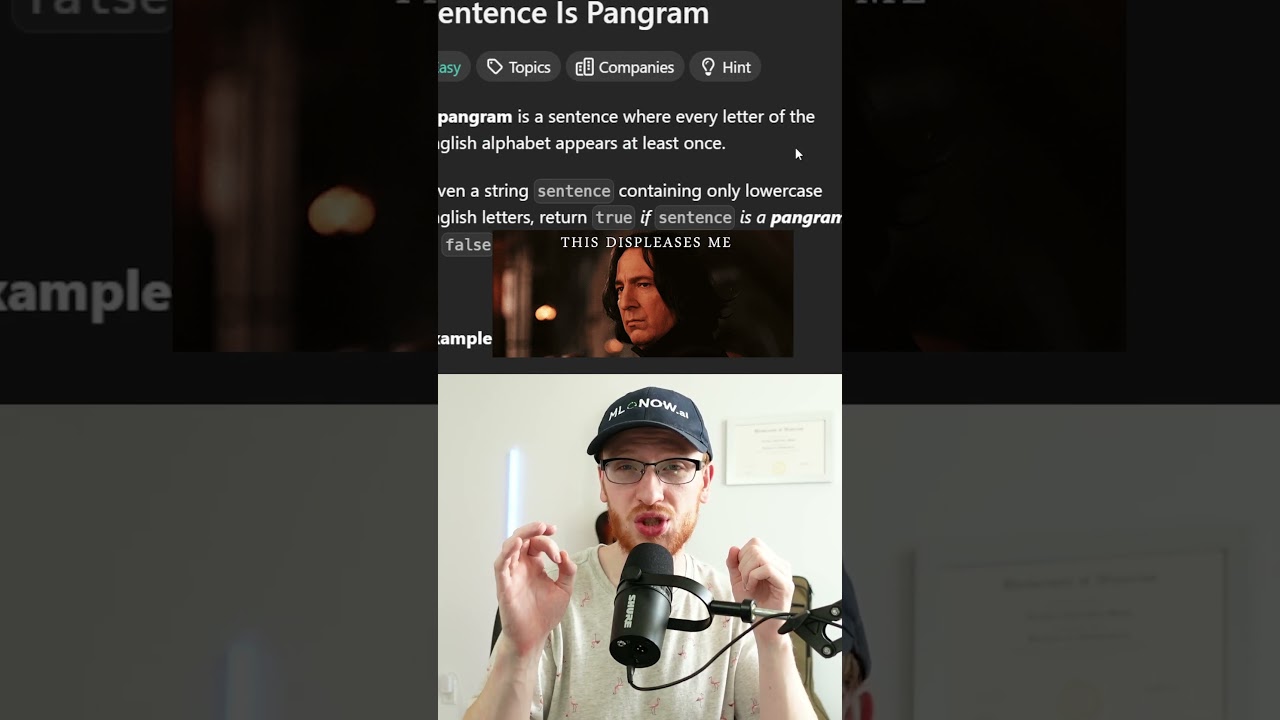
Показать описание
FAANG Coding Interviews / Data Structures and Algorithms / Leetcode
Google Just Hit an Absolute Low - Easy Interview Question - Check if Sentence is Pangram - 1832
7 times Elmo was an Absolute SAVAGE
7 Rewards YOU MUST Unlock after Fortnite LIVE EVENT!
Jack Black as Bob Ross is absolute gold 😂 | SPIN
Fortnite DOOM Live Event Full Cinematic
Fortnite’s *NEW* UPDATE ADDED A Mythic!
Right Now, They're in an Absolute State of Panic!
I Just BROKE Fortnite's HARDEST Record...
Talk To Your AI Assistant: My ABSOLUTE New Favourite AI Efficiency Hack
Excel – Absolute references with a shortcut (F4) to fix the cells in Excel
Fortnite DOOM EVENT is LIVE!
Absolute Cell References in Google Sheets
Absolute and Relative References - Google Sheets
Calculating Averages and Mean Absolute Deviation in Google Spreadsheets
Absolute and Relative References in Excel
MASTERING Stack Column Chart in Excel | SWITCH between PERCENTAGE % and ABSOLUTE VALUES with SLICER
Pickleball Rules | The Definitive Beginner's Resource to How to Play Pickleball
Add Absolute Reference With One Key #excel #shorts @XLLearner_Courses
Arsenal MAKE THEM CRY😢 Goldbridge HITS BACK HARD😲TalkSPORT HATE CALLED OUT & AFTV Massive CLAIM🚨...
ABSOLUTE PEAK BLASTER! #shorts
12 Natural Ways to Get Rid of Cockroaches Permanently
Relative & Absolute References in Google Sheets
What is Absolute Value? | Absolute Value Examples | Math with Mr. J
Still in shock The Rock, Stone Cold AND Hulk Hogan shared a ring together on this day in 2014!
Комментарии