filmov
tv
Understanding and Fixing IndexError: list index out of range in Python
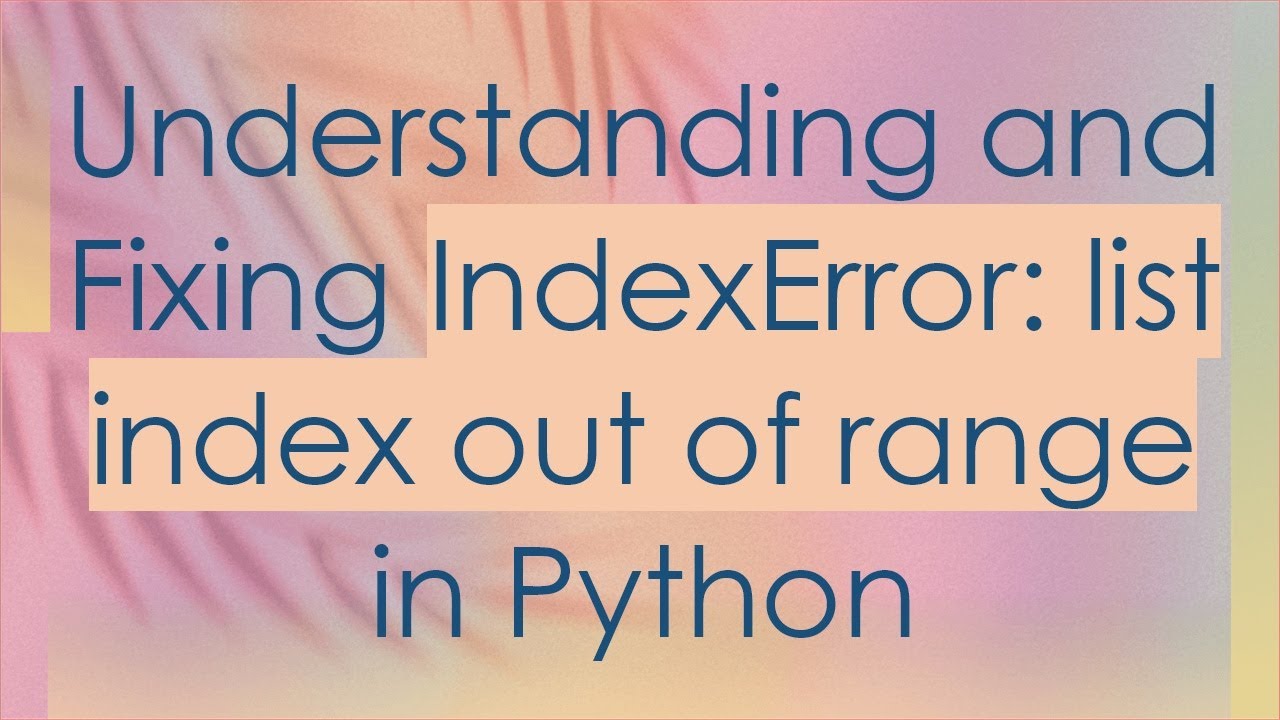
Показать описание
Summary: Learn why you encounter `IndexError: list index out of range` when working with lists in Python and discover strategies to fix it in your code. Explore common scenarios and solutions to avoid this error in Python 3.x.
---
Understanding and Fixing IndexError: list index out of range in Python
If you have worked with lists in Python, you might have encountered the IndexError: list index out of range. This error can be frustrating, especially for those newer to Python. This guide aims to help you understand why you get this error and how to fix it.
What is IndexError: list index out of range?
An IndexError occurs in Python when you try to access an index that is not within the range of the list. Lists in Python are zero-based, meaning the first element is at index 0, the second at index 1, and so on. If you try to access an index that is outside the bounds of the list, Python raises an IndexError.
Example of IndexError
[[See Video to Reveal this Text or Code Snippet]]
In the above example, my_list has elements at indices 0, 1, and 2. Trying to access my_list[3] raises an IndexError because there is no element at index 3.
Common Scenarios for IndexError
Looping Beyond List Range
A common scenario is using a loop to iterate over list indices. If the loop goes beyond the last index of the list, it will result in an IndexError.
[[See Video to Reveal this Text or Code Snippet]]
Hardcoding Indices
Hardcoding an index without ensuring the list length can lead to errors if the list changes.
[[See Video to Reveal this Text or Code Snippet]]
Negative Indexes
Negative indexes in Python refer to elements from the end of the list. However, if the negative index exceeds the list length in the negative direction, Python throws an IndexError.
[[See Video to Reveal this Text or Code Snippet]]
Strategies to Fix IndexError
Check List Length
Before accessing an index, use a condition to check if the index is within the list bounds.
[[See Video to Reveal this Text or Code Snippet]]
Use try-except Block
Catch the IndexError using a try-except block to handle cases where the index is out of range gracefully.
[[See Video to Reveal this Text or Code Snippet]]
Use enumerate in Loops
When iterating over elements in a list, using enumerate can help avoid IndexError.
[[See Video to Reveal this Text or Code Snippet]]
Ensure Proper List Population
Sometimes, list indices might be accessed after erroneous list operations. Ensure lists are populated as expected before accessing elements.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The IndexError: list index out of range is a common error in Python, especially for newcomers. By understanding why it happens and applying the strategies mentioned above, you can avoid and fix this error effectively. Always verify your list operations and indices to ensure your code handles list boundaries correctly.
Happy coding!
---
Understanding and Fixing IndexError: list index out of range in Python
If you have worked with lists in Python, you might have encountered the IndexError: list index out of range. This error can be frustrating, especially for those newer to Python. This guide aims to help you understand why you get this error and how to fix it.
What is IndexError: list index out of range?
An IndexError occurs in Python when you try to access an index that is not within the range of the list. Lists in Python are zero-based, meaning the first element is at index 0, the second at index 1, and so on. If you try to access an index that is outside the bounds of the list, Python raises an IndexError.
Example of IndexError
[[See Video to Reveal this Text or Code Snippet]]
In the above example, my_list has elements at indices 0, 1, and 2. Trying to access my_list[3] raises an IndexError because there is no element at index 3.
Common Scenarios for IndexError
Looping Beyond List Range
A common scenario is using a loop to iterate over list indices. If the loop goes beyond the last index of the list, it will result in an IndexError.
[[See Video to Reveal this Text or Code Snippet]]
Hardcoding Indices
Hardcoding an index without ensuring the list length can lead to errors if the list changes.
[[See Video to Reveal this Text or Code Snippet]]
Negative Indexes
Negative indexes in Python refer to elements from the end of the list. However, if the negative index exceeds the list length in the negative direction, Python throws an IndexError.
[[See Video to Reveal this Text or Code Snippet]]
Strategies to Fix IndexError
Check List Length
Before accessing an index, use a condition to check if the index is within the list bounds.
[[See Video to Reveal this Text or Code Snippet]]
Use try-except Block
Catch the IndexError using a try-except block to handle cases where the index is out of range gracefully.
[[See Video to Reveal this Text or Code Snippet]]
Use enumerate in Loops
When iterating over elements in a list, using enumerate can help avoid IndexError.
[[See Video to Reveal this Text or Code Snippet]]
Ensure Proper List Population
Sometimes, list indices might be accessed after erroneous list operations. Ensure lists are populated as expected before accessing elements.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The IndexError: list index out of range is a common error in Python, especially for newcomers. By understanding why it happens and applying the strategies mentioned above, you can avoid and fix this error effectively. Always verify your list operations and indices to ensure your code handles list boundaries correctly.
Happy coding!