filmov
tv
Visitor: How I Mastered the Toughest Programming Pattern
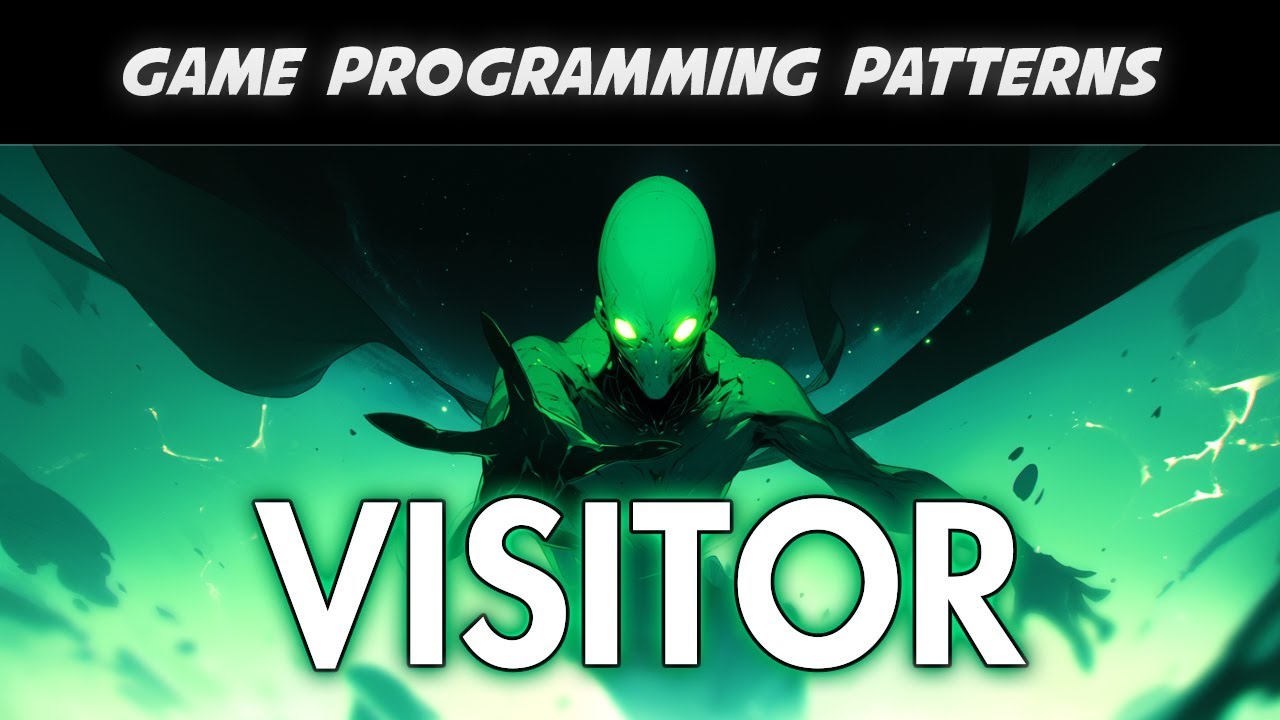
Показать описание
Unity C# Architecture: This is how I finally understood the Visitor Programming Pattern - Building a Power Up System in Unity.
#unity3d #gamedev #indiedev
▬ Contents of this video ▬▬▬▬▬▬▬▬▬▬
0:00 Classic Visitor
5:58 Intrusive Visitor
7:13 Reflective Visitor
9:43 GetOrAdd Extension Method
Extension Methods and Utils
*Assets Shown In This Video* (Affiliate Links)
*Follow me!*
#unity3d #gamedev #indiedev
▬ Contents of this video ▬▬▬▬▬▬▬▬▬▬
0:00 Classic Visitor
5:58 Intrusive Visitor
7:13 Reflective Visitor
9:43 GetOrAdd Extension Method
Extension Methods and Utils
*Assets Shown In This Video* (Affiliate Links)
*Follow me!*
Visitor: How I Mastered the Toughest Programming Pattern
Mastering Java Design Patterns: The Visitor Pattern Explained with Examples | Design Patterns
Master's Program Urban Tourism & Visitor Economy Management
Mastering the Hero Section: Guide Visitors with Clear Next Steps
Mastering Online Store Traffic: Proven Strategies to Drive Massive Visitors to Your Website
Configure a new visitor id Master Installation
Mastering the Visitor Pattern in Java: Your Ultimate Guide!
Mastering Java: Rewriting Singleton, Visitor, and Strategy Design Patterns
How To Fill DS 160 Form for US Visitor Visa | How To Apply for US Visa 2024
Master the Art: Step-by-Step Guide to Your Parents' Visitor Visa Letter!
I sit by my self (own edit) #gamergirl #roblox #robloxgirlsplays
new visitor vip in tanghulu master so cutee #tanghulumaster
Master the Visitor Experience with GPS Mapper
'Mastering Unique Visitor Tracking'
[TOS] Visitor from the afar - Dark *Grand Master
Visitor giving Milk to master chief.
Mastering Visitor Behavior Tracking for B2B Growth
Review by learned visitor 😇#3bhk #flats #luxuryhomes #highrisebuildings #property #3bhkapartment
UK Visitor Visa Secrets: Mastering the Application Process
Visitor taking the music dna master class
I want to seek visitor master
Visitor skin master
Smooth Gateway to Canada | Mastering Visitor Visa Paperwork
1st PLAY #ABBA THE VISITORS HALF SPEED MASTER-REACTION
Комментарии