filmov
tv
Android Development Course - Build Native Apps with Kotlin Tutorial
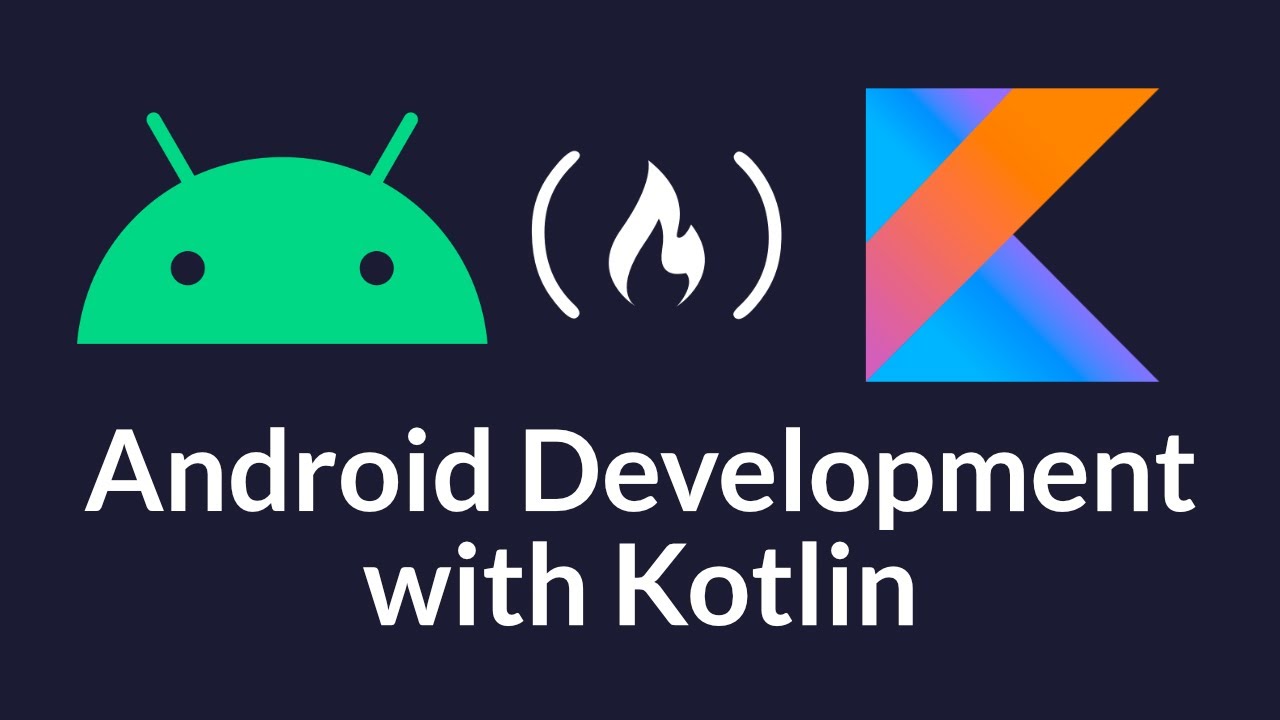
Показать описание
Learn to build native Android apps with Kotlin. You will also learn how to use Android Jetpack, Firebase, Room, MVVM, Navigation, LiveData, and Kotlin Coroutines. This full course explains how to build an entire Android app using best practices.
⭐️ Course Contents ⭐️
1
(0:00) Introduction
2
(5:14) Model View ViewModel (MVVM) Architecture fundamentals and variations
(5:38) Is MVVM the perfect architecture?
(7:41) Overview of MVVM with whiteboard example
(12:11) Pros and Cons of MVVM
(18:58) Package structure
3
(23:22) How to create Vector Drawables
(24:22) Difference between Raster (PNG, JPG, BMP) and Vector (SVG) Images?
(27:55) Uh Oh!
(28:41) How to use Inkscape to create an SVG from a BMP/PNG
(30:07) How to convert an SVG into an Android Studio Vector Asset
(32:29) How to create simple animations using animation lists and fade animations
4
(38:19) Material Design User Interface using ConstraintLayout and Styles
(41:00) Building an XML Layout using ConstraintLayout
(51:09) Using ConstraintLayout Chains
(55:43) Some tips on using Styles to create a material design look
5
(56:51) How to use (and when not to use) the Android Navigation Component from Android Jetpack
(1:00:00) Adding Safe Args to gradle
(1:01:15) Building a Navigation Graph using the Navigation Editor (XML)
(1:11:00) Creating a NavHostFragment to host the Navigation Component and adding it to an Activity
(1:15:29) How to actually change destinations in our Fragments using the Navigation Component (with safe args)
6
(1:20:00) Setting up Fragments as Views in MVVM using Kotlin Synthetic Properties
(1:25:32) Starting point
(1:27:54) Importing an XML layout to an Activity/Fragment using Kotlin Synthetic Properties
(1:32:09) How to reference Views in the Fragment using synthetic properties
(1:33:41) Adding onClickListener to our views
(1:38:03) Intercepting OnBackPressed (NOTE: The API for this has changed since making this video. I have updated the Repository appropriately)
(1:39:40) How to observe the ViewModel using LiveData callbacks
7
(1:43:22) ViewModels in MVVM with Kotlin Coroutines for Concurrency
(1:45:26) A look at my BaseViewModel class which contains part of the Coroutines Implementation (Coroutine Scope)
(1:49:25) Starting point
(2:01:40) Handling events from the View in the ViewModel
(2:05:34) Using the launch Coroutine Builder to access data
(2:08:44) How to update the MutableLiveData fields to publish data to the View
8
(2:19:35) Modern RecyclerView implementation using LiveData to handle ItemTouchListener() callbacks
(2:22:49) Overview of the RecyclerView within the XML View with LinearLayoutManager
(2:26:53) What does the DiffUtil do?
(2:28:48) A look at the source code of ListAdapter to better understand how it works
(2:35:34) Writing the RecyclerView.ViewHolder class
(2:37:23) Why is it actually called a RecyclerView? What gets Recycled?
(2:37:58) Writing our RecyclerView.ListAdapter
(2:46:37) How to use a MutableLiveData object to publish events from the onClickListener in the ViewHolders
(2:48:09) Overview of the Fragment which manages the RecyclerView, and how to avoid memory leaks!
9
(2:53:17) FirebaseAuth for User Authentication using Google Sign In
(2:54:00) How to set up a new Firebase Project using Android Studio
(2:54:36) Enabling Authentication (FirebaseAuth) in the Firebase Console
(2:55:00) Setting up a debug signing certificate for your App in Firebase
(2:56:48) Gradle Configurations for FirebaseAuth
(2:58:00) Setting up GoogleSignInProvider
(2:59:24) Handling the result in onActivityResult
(3:01:06) Back FirebaseAuth Implementation using Coroutines
10
(3:05:31) Local Database with Room Persistence Library and Coroutines
(3:06:17) Entities and Primary Keys in Room
(3:08:50) Setting up a Dao (Data Access Object) in Room
(3:12:28) Overview of RoomDatabase implementation
(3:14:21) How to build your Database and get a reference to you Dao
(3:14:47) How to get data in and out of the Dao using suspend functions
11
(3:16:34) Setting up a Local and Remote Database using Firebase's Firestore NoSQL library
(3:17:33) Communicating with Firestore using a Repository, with Coroutines
12
(3:21:59) Dependency Injection using an AndroidViewModel, and ViewModelProvider.Factory
(3:22:29) What is dependency injection in simple terms?
(3:23:36) Implementing Dependency Injection using AndroidViewModel
(3:24:13) Quick look at using a ViewModelProvider.Factory to create our ViewModel with the Injected Repository
(3:24:27) How to use our Dependency Injector from within a Fragment or Activity
⭐️ Course Contents ⭐️
1
(0:00) Introduction
2
(5:14) Model View ViewModel (MVVM) Architecture fundamentals and variations
(5:38) Is MVVM the perfect architecture?
(7:41) Overview of MVVM with whiteboard example
(12:11) Pros and Cons of MVVM
(18:58) Package structure
3
(23:22) How to create Vector Drawables
(24:22) Difference between Raster (PNG, JPG, BMP) and Vector (SVG) Images?
(27:55) Uh Oh!
(28:41) How to use Inkscape to create an SVG from a BMP/PNG
(30:07) How to convert an SVG into an Android Studio Vector Asset
(32:29) How to create simple animations using animation lists and fade animations
4
(38:19) Material Design User Interface using ConstraintLayout and Styles
(41:00) Building an XML Layout using ConstraintLayout
(51:09) Using ConstraintLayout Chains
(55:43) Some tips on using Styles to create a material design look
5
(56:51) How to use (and when not to use) the Android Navigation Component from Android Jetpack
(1:00:00) Adding Safe Args to gradle
(1:01:15) Building a Navigation Graph using the Navigation Editor (XML)
(1:11:00) Creating a NavHostFragment to host the Navigation Component and adding it to an Activity
(1:15:29) How to actually change destinations in our Fragments using the Navigation Component (with safe args)
6
(1:20:00) Setting up Fragments as Views in MVVM using Kotlin Synthetic Properties
(1:25:32) Starting point
(1:27:54) Importing an XML layout to an Activity/Fragment using Kotlin Synthetic Properties
(1:32:09) How to reference Views in the Fragment using synthetic properties
(1:33:41) Adding onClickListener to our views
(1:38:03) Intercepting OnBackPressed (NOTE: The API for this has changed since making this video. I have updated the Repository appropriately)
(1:39:40) How to observe the ViewModel using LiveData callbacks
7
(1:43:22) ViewModels in MVVM with Kotlin Coroutines for Concurrency
(1:45:26) A look at my BaseViewModel class which contains part of the Coroutines Implementation (Coroutine Scope)
(1:49:25) Starting point
(2:01:40) Handling events from the View in the ViewModel
(2:05:34) Using the launch Coroutine Builder to access data
(2:08:44) How to update the MutableLiveData fields to publish data to the View
8
(2:19:35) Modern RecyclerView implementation using LiveData to handle ItemTouchListener() callbacks
(2:22:49) Overview of the RecyclerView within the XML View with LinearLayoutManager
(2:26:53) What does the DiffUtil do?
(2:28:48) A look at the source code of ListAdapter to better understand how it works
(2:35:34) Writing the RecyclerView.ViewHolder class
(2:37:23) Why is it actually called a RecyclerView? What gets Recycled?
(2:37:58) Writing our RecyclerView.ListAdapter
(2:46:37) How to use a MutableLiveData object to publish events from the onClickListener in the ViewHolders
(2:48:09) Overview of the Fragment which manages the RecyclerView, and how to avoid memory leaks!
9
(2:53:17) FirebaseAuth for User Authentication using Google Sign In
(2:54:00) How to set up a new Firebase Project using Android Studio
(2:54:36) Enabling Authentication (FirebaseAuth) in the Firebase Console
(2:55:00) Setting up a debug signing certificate for your App in Firebase
(2:56:48) Gradle Configurations for FirebaseAuth
(2:58:00) Setting up GoogleSignInProvider
(2:59:24) Handling the result in onActivityResult
(3:01:06) Back FirebaseAuth Implementation using Coroutines
10
(3:05:31) Local Database with Room Persistence Library and Coroutines
(3:06:17) Entities and Primary Keys in Room
(3:08:50) Setting up a Dao (Data Access Object) in Room
(3:12:28) Overview of RoomDatabase implementation
(3:14:21) How to build your Database and get a reference to you Dao
(3:14:47) How to get data in and out of the Dao using suspend functions
11
(3:16:34) Setting up a Local and Remote Database using Firebase's Firestore NoSQL library
(3:17:33) Communicating with Firestore using a Repository, with Coroutines
12
(3:21:59) Dependency Injection using an AndroidViewModel, and ViewModelProvider.Factory
(3:22:29) What is dependency injection in simple terms?
(3:23:36) Implementing Dependency Injection using AndroidViewModel
(3:24:13) Quick look at using a ViewModelProvider.Factory to create our ViewModel with the Injected Repository
(3:24:27) How to use our Dependency Injector from within a Fragment or Activity
Комментарии