filmov
tv
8 Find an Element in a Rotated Sorted Array
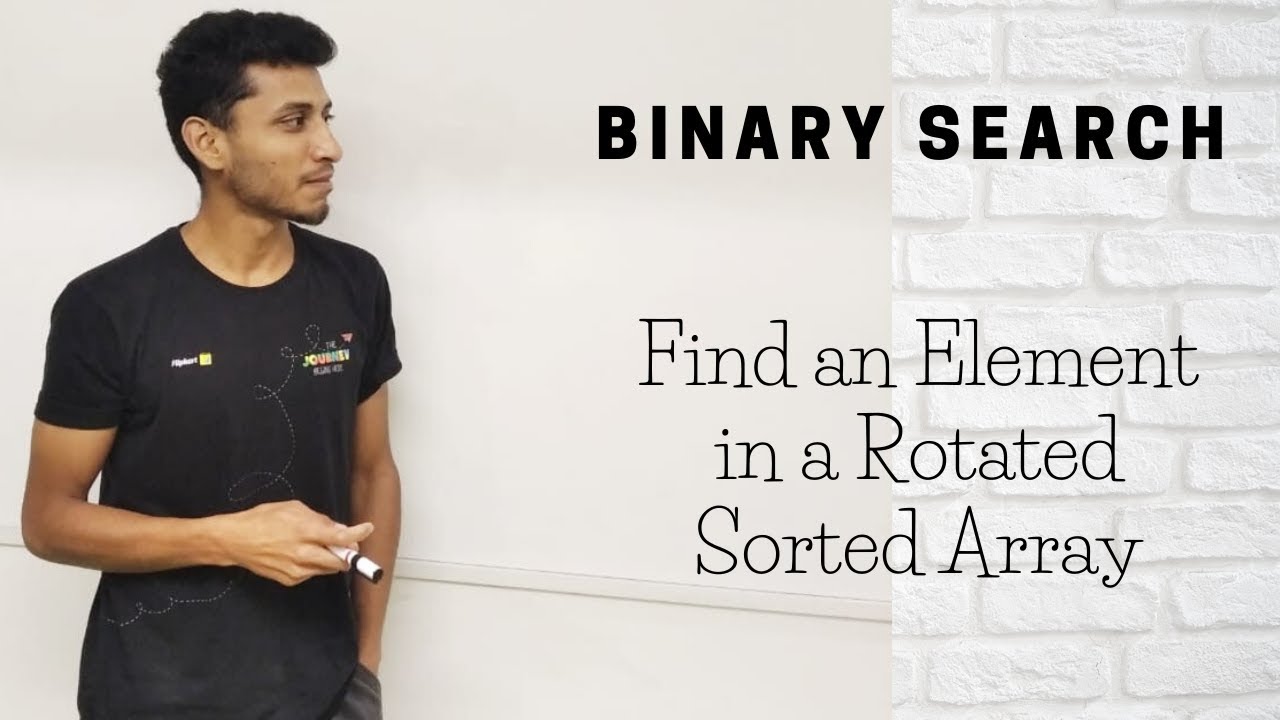
Показать описание
FIND AN ELEMENT IN A ROTATED SORTED ARRAY:
Suppose an array sorted in ascending order is rotated at some pivot unknown to you beforehand.
(i.e., [0,1,2,4,5,6,7] might become [4,5,6,7,0,1,2]).
You are given a target value to search. If found in the array return its index, otherwise return -1.
You may assume no duplicate exists in the array.
Your algorithm's runtime complexity must be in the order of O(log n).
Example:
Input: nums = [4,5,6,7,0,1,2], target = 0
Output: 4
------------------------------------------------------------------------------------------
Here are some of the gears that I use almost everyday:
PS: While having good gears help you perform efficiently, don’t get under the impression that they will make you successful without any hard work.
Suppose an array sorted in ascending order is rotated at some pivot unknown to you beforehand.
(i.e., [0,1,2,4,5,6,7] might become [4,5,6,7,0,1,2]).
You are given a target value to search. If found in the array return its index, otherwise return -1.
You may assume no duplicate exists in the array.
Your algorithm's runtime complexity must be in the order of O(log n).
Example:
Input: nums = [4,5,6,7,0,1,2], target = 0
Output: 4
------------------------------------------------------------------------------------------
Here are some of the gears that I use almost everyday:
PS: While having good gears help you perform efficiently, don’t get under the impression that they will make you successful without any hard work.
8 Find an Element in a Rotated Sorted Array
BS-8. Single Element in Sorted Array
Find an element in a sorted rotated array
Find maximum element in an array (Largest element)
Find minimum (Smallest) element in Array
Find Second Largest Element in list/array | Java8 Stream Tutorial
LeetCode Find First and Last Position of Element in Sorted Array Solution Explained - Java
BS-9. Find Peak Element
Finding, Timing, and Buying Growth Stocks on the thinkorswim platform | Trading Growth & Value
Find Majority Element in an Array
Search an element in a Sorted & Rotated Array | Binary Search, Part 3 | DSA-One Course #24
How to find the Protons Neutrons and Electrons of an element on the Periodic table
Search an element in an Infinite Sorted array | Binary Search, Part 2 | DSA-One Course #23
What Is Your Soul Element? Cool Personality Test
Search an element in sorted and rotated array( Find PIVOT)
How to Write the Electron Configuration for an Element in Each Block
Find the element that appears once in an array
BS-4. Search Element in Rotated Sorted Array - I
Find Peak Element - Leetcode 162 - Python
#8. Search Element In Array Using Linear Search - Step By Step Logic Explained
How to find Group, Period and Block of an element?
Find min and max element in a binary search tree
How to search an element in an array in C++
Frequency of each element in an integer array | Brute force | JAVA interview question
Комментарии