filmov
tv
Debugging Your HTTP DELETE Issue in C# API Development: A Step-By-Step Guide
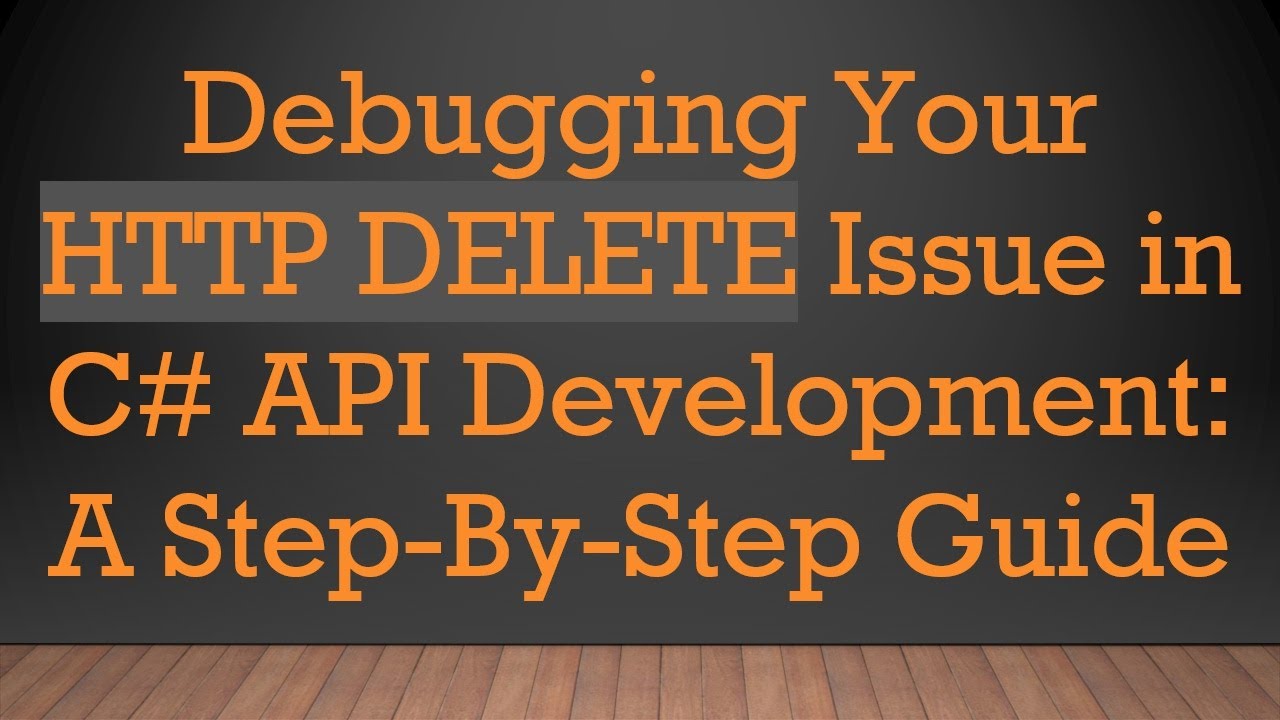
Показать описание
Struggling with the `HTTP DELETE` method in your C# API? Discover why it might be returning a success code without actually removing items from your database and learn how to fix it!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Trying to delete from database using HTTPDELETE does nothing
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Debugging Your HTTP DELETE Issue in C# API Development: A Step-By-Step Guide
When developing an API application in C# , encountering issues with the HTTP DELETE method can be frustrating. You might find that the DELETE request returns a success code (200), yet the item remains untouched in your database. This disconnect can hinder your application's functionality and user experience. In this guide, we’ll explore common pitfalls in implementing the DELETE functionality and provide solutions to ensure your code runs smoothly.
Understanding the Problem
You’ve written an API controller to handle the deletion of database entities, but despite receiving a success response, nothing is actually deleted from the database. The structure of your code seems sound at first glance, but there may be underlying issues related to the way the unit of work pattern and repository pattern are implemented.
Let's walk through your existing setup:
Controller Code Overview
You have a controller method that looks like this:
[[See Video to Reveal this Text or Code Snippet]]
This method appears to request the deletion of an order via the _orderService, with a basic error handling mechanism in place.
Service and Repository Structure
Your OrderService and repository classes follow a general structure for managing database entities successfully, but there are critical items to review:
OrderService calls the Delete method from the repository.
The UnitOfWork handles transactions.
However, there are a couple of important details we must verify.
Solutions to Check
Let’s break down the steps to diagnose and resolve the issue effectively:
1. Ensure Save Changes Method is Called
First, verify that your UnitOfWork implementation correctly invokes SaveChanges() in the CommitAsync() method. Without this step, the changes in your unit of work—including deletes—will not be applied to the database.
[[See Video to Reveal this Text or Code Snippet]]
This line commits any changes made through the unit of work and ensures that deletions are processed.
2. Validate the NorthwindContext Instance
Second, make sure that the NorthwindContext object you pass to your repositories is the same instance used in your UnitOfWork. If different instances are used, your operations may pertain to distinct transactions, leading to discrepancies.
[[See Video to Reveal this Text or Code Snippet]]
By confirming that the same context is used throughout, you can ensure transactional integrity and proper database operations.
Conclusion
Having an effective DELETE method in your API is crucial for managing data integrity. By examining the above areas and ensuring that SaveChanges() is executed and that the same NorthwindContext instance is used across your service and repositories, you can resolve the issue of deletions not being reflected in your database.
If you continue to face challenges after following these guidelines, consider debugging step-by-step or logging more detailed error messages to help pinpoint where the failure occurs.
By addressing these aspects, you can ensure that your DELETE functionality not only returns a success status but also performs as intended, enhancing the robustness of your C# API application.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Trying to delete from database using HTTPDELETE does nothing
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Debugging Your HTTP DELETE Issue in C# API Development: A Step-By-Step Guide
When developing an API application in C# , encountering issues with the HTTP DELETE method can be frustrating. You might find that the DELETE request returns a success code (200), yet the item remains untouched in your database. This disconnect can hinder your application's functionality and user experience. In this guide, we’ll explore common pitfalls in implementing the DELETE functionality and provide solutions to ensure your code runs smoothly.
Understanding the Problem
You’ve written an API controller to handle the deletion of database entities, but despite receiving a success response, nothing is actually deleted from the database. The structure of your code seems sound at first glance, but there may be underlying issues related to the way the unit of work pattern and repository pattern are implemented.
Let's walk through your existing setup:
Controller Code Overview
You have a controller method that looks like this:
[[See Video to Reveal this Text or Code Snippet]]
This method appears to request the deletion of an order via the _orderService, with a basic error handling mechanism in place.
Service and Repository Structure
Your OrderService and repository classes follow a general structure for managing database entities successfully, but there are critical items to review:
OrderService calls the Delete method from the repository.
The UnitOfWork handles transactions.
However, there are a couple of important details we must verify.
Solutions to Check
Let’s break down the steps to diagnose and resolve the issue effectively:
1. Ensure Save Changes Method is Called
First, verify that your UnitOfWork implementation correctly invokes SaveChanges() in the CommitAsync() method. Without this step, the changes in your unit of work—including deletes—will not be applied to the database.
[[See Video to Reveal this Text or Code Snippet]]
This line commits any changes made through the unit of work and ensures that deletions are processed.
2. Validate the NorthwindContext Instance
Second, make sure that the NorthwindContext object you pass to your repositories is the same instance used in your UnitOfWork. If different instances are used, your operations may pertain to distinct transactions, leading to discrepancies.
[[See Video to Reveal this Text or Code Snippet]]
By confirming that the same context is used throughout, you can ensure transactional integrity and proper database operations.
Conclusion
Having an effective DELETE method in your API is crucial for managing data integrity. By examining the above areas and ensuring that SaveChanges() is executed and that the same NorthwindContext instance is used across your service and repositories, you can resolve the issue of deletions not being reflected in your database.
If you continue to face challenges after following these guidelines, consider debugging step-by-step or logging more detailed error messages to help pinpoint where the failure occurs.
By addressing these aspects, you can ensure that your DELETE functionality not only returns a success status but also performs as intended, enhancing the robustness of your C# API application.