filmov
tv
What are JavaScript PROMISES? 🤞
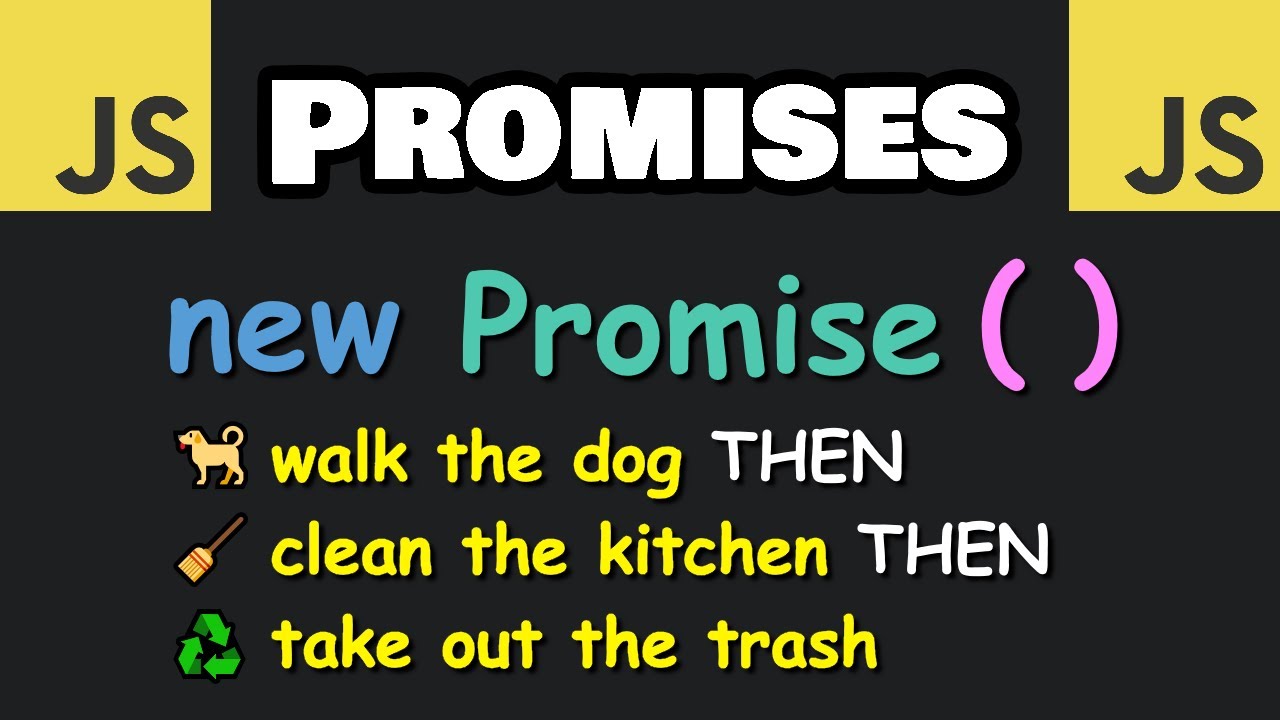
Показать описание
#JavaScript #tutorial #courses
// Promise = An Object that manages asynchronous operations.
// Wrap a Promise Object around {asynchronous code}
// "I promise to return a value"
// DO THESE CHORES IN ORDER
// 1. WALK THE DOG
// 2. CLEAN THE KITCHEN
// 3. TAKE OUT THE TRASH
// Promise = An Object that manages asynchronous operations.
// Wrap a Promise Object around {asynchronous code}
// "I promise to return a value"
// DO THESE CHORES IN ORDER
// 1. WALK THE DOG
// 2. CLEAN THE KITCHEN
// 3. TAKE OUT THE TRASH
JavaScript Promise in 100 Seconds
JavaScript Promises In 10 Minutes
What are JavaScript PROMISES? 🤞
JavaScript Promises Crash Course
JavaScript Promises for Complete Beginners
JavaScript Promises -- Tutorial for Beginners
Async JS Crash Course - Callbacks, Promises, Async Await
The Async Await Episode I Promised
[Lesson] Callback/Promise/async in JS
Asynchronous JavaScript Tutorial #7 - Promises
Promises | Ep 02 Season 02 - Namaste JavaScript
Asynchronous JavaScript in ~10 Minutes - Callbacks, Promises, and Async/Await
javaScript promises explained tutorial
JavaScript Promises | JavaScript Promises Explained | JavaScript Tutorial For Beginners |Simplilearn
Learn JavaScript Promises in 19 minutes (For Beginners)
JavaScript Promises Tutorial: Asynchrone Operationen ohne Callback Hell mit Promises
Asynchronous JavaScript Course (Async/Await, Promises, Callbacks)
🕗Javascript Promises Explained in 60 Seconds! 🔥 #javascript #tutorial #beginner #code
Introduction to Promises | JavaScript Tutorial in Hindi #54
JavaScript Promises 👨🏻💻in 1 Minute #shorts
Promises in Javascript
Javascript Interview Questions ( Promises ) - Polyfills, Callbacks, Async/await, Output Based, etc
16.11: Promises Part 1 - Topics of JavaScript/ES6
Asynchronous Javascript Tutorial - Promises and Async Await Explained
Комментарии