filmov
tv
Lecture 8 : OOPS in Python | Object Oriented Programming | Classes & Objects | Python Full Course
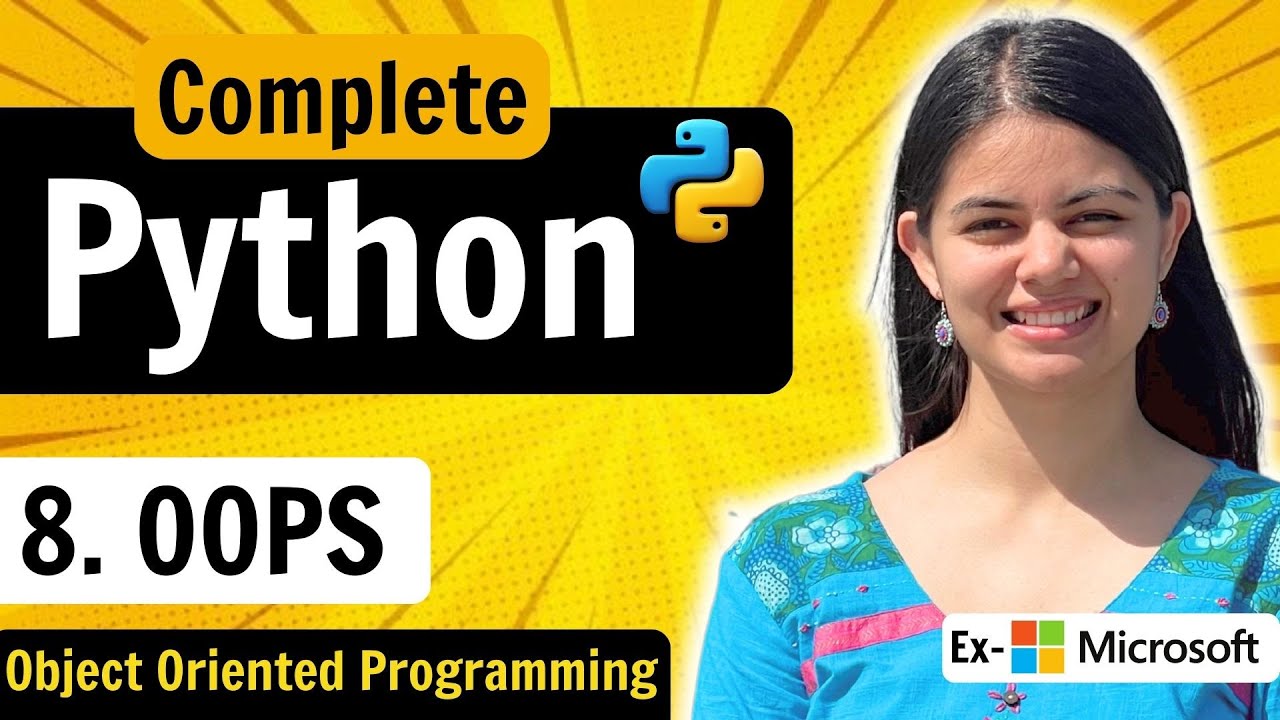
Показать описание
This lecture was made with a lot of love❤️
Lecture 8 : OOPS in Python | Object Oriented Programming | Classes & Objects | Python Full Cours...
8. Object Oriented Programming
The Pain of OOP, Lecture #8: Inheritance [object oriented programming crash course]
OOP in Java - Lecture 8
Lecture 9 : OOPS Part 2 | Object Oriented Programming | Python Full Course
Java OOPs in One Shot | Object Oriented Programming | Java Language | Placement Course
C# Expert OOP - Lecture 8: Class Inheritance Tutorial/Example & How To Use Dynamic-Link Library ...
How to Answer Any Question on a Test
Lecture 2 - Access Specifier in Inheritance | SPPU OOPs Series | SPPU | SoloScholar
Lecture 8 | Module 4 | OOPS with C++ Training | KEC Ghaziabad
Lecture 8 - Constructors | SPPU OOPs Series | SPPU | SoloScholar
Lecture 8 (part I) || CS304 (OOP) || Short lecture
Java Basic OOP Concepts | Features of OOPs in Java | Learn Coding
1: Introduction To OOP PHP | Object Oriented PHP Tutorial For Beginners | PHP Tutorial | mmtuts
Java OOPs Concepts in 120 minutes |Object Oriented Programming | Java Placement Course | Simplilearn
OOPs Interview Questions | Object-Oriented Programming Interview Questions And Answers | Intellipaat
OCJA (1Z0 - 808 ) || Java SE 8 Programmer I || OOPS - Inheritance Introduction || Durga Sir
Have you heard of the OOPS Method? | Rubik's Cube #shorts
Lecture 8 (Part II) || CS304 (OOP) || Short lecture
*I HAD TO TAKE CONTROL* #driving #lesson #manual #car #gear #oops #ohno #fail
Learn Java 8 - Full Tutorial for Beginners
Object Oriented Programming (OOPs) Concepts In Java || by Durga sir
Java OOPs Concepts in just 60 minutes | Object Oriented Programming | Java Tutorial For Beginners
Classes and Objects explained - OOP programming #shorts
Комментарии