filmov
tv
How to Access a Variable Outside of a Function in Python
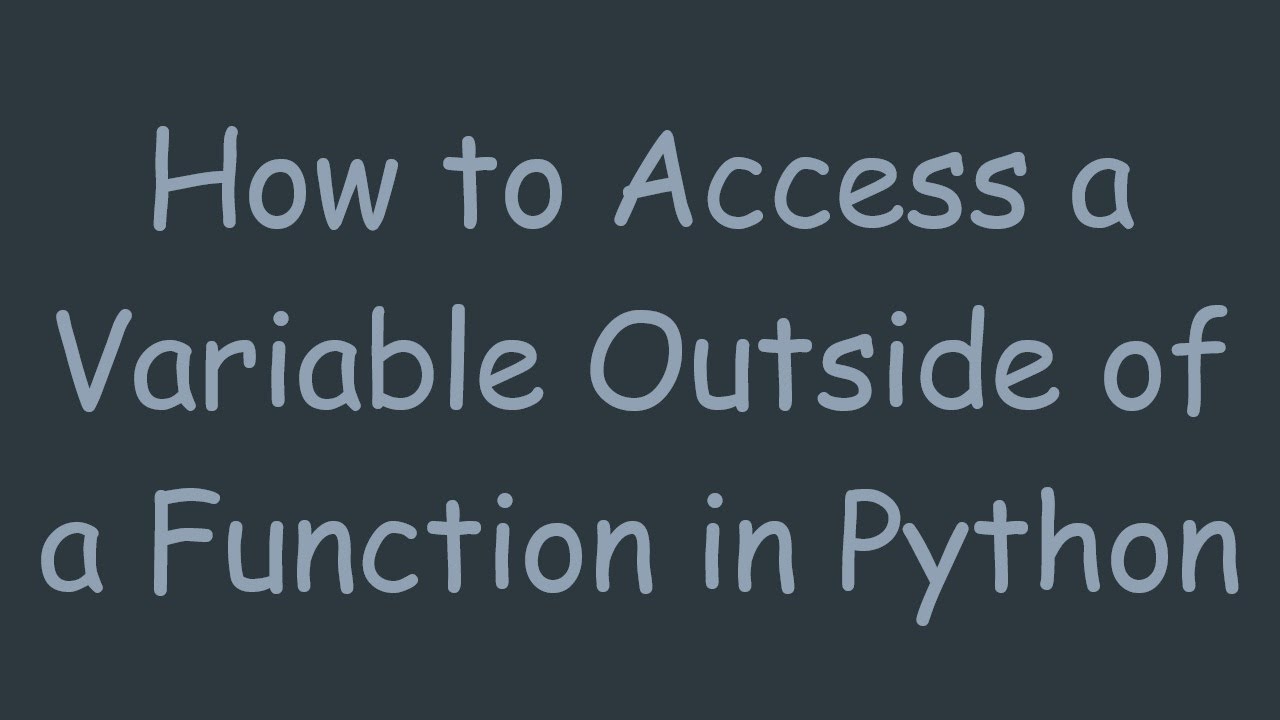
Показать описание
Learn how to properly access variables outside functions in Python, specifically through the use of global variables and restructuring your code.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to access a variable outside of a function (access from a loop)?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Access a Variable Outside of a Function in Python
Python's variable scope can sometimes be confusing, especially when you want to access a variable defined inside a function or loop. If you've ever encountered a NameError while trying to access a variable globally, you are not alone. In this post, we will break down how to correctly access a variable outside of a function using global variables, highlighting key steps to avoid common pitfalls.
Understanding the Problem
In the provided code example, the goal is to access the variable trigger_gmm outside the spatter_tracking_cb function. However, when trying to access this variable, you might encounter the following error message:
[[See Video to Reveal this Text or Code Snippet]]
This error occurs because trigger_gmm has not been defined in the expected scope for the code located outside of the function. Let's explore how to resolve this issue.
Step-by-Step Solution
1. Declare the Global Variable Properly
To use a variable as a global variable inside a function, you need to declare it as global within that function. This informs Python that you intend to use the globally defined version of the variable instead of creating a local one.
However, it’s also important to declare trigger_gmm as global in the main function where it's first assigned a value.
Correct Declaration:
[[See Video to Reveal this Text or Code Snippet]]
2. Removal of Unnecessary Parentheses
In the original code, there is a typo where trigger_gmm() is mistakenly referenced. Since this is meant to assign 0 to trigger_gmm, parentheses should be removed to prevent errors:
[[See Video to Reveal this Text or Code Snippet]]
3. Restructure Code for Global Access
The placement of the if __name__ == "__main__": block is also crucial. This block should be placed outside of any loops or functions and executed first. You need to ensure that the program starts at the main entry point properly before any infinite loops are initiated.
4. Implementing the Changes
Now that we've identified the issues, let's revise the entire code:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following these steps, you can confidently access and manipulate variables outside of functions in Python. Remember to declare your global variables properly and structure your code so that it executes in the correct scope. This knowledge not only aids in troubleshooting but also enhances your overall coding skills.
Whether you're working on a small project or a larger application, understanding variable scope in Python is fundamental. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to access a variable outside of a function (access from a loop)?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Access a Variable Outside of a Function in Python
Python's variable scope can sometimes be confusing, especially when you want to access a variable defined inside a function or loop. If you've ever encountered a NameError while trying to access a variable globally, you are not alone. In this post, we will break down how to correctly access a variable outside of a function using global variables, highlighting key steps to avoid common pitfalls.
Understanding the Problem
In the provided code example, the goal is to access the variable trigger_gmm outside the spatter_tracking_cb function. However, when trying to access this variable, you might encounter the following error message:
[[See Video to Reveal this Text or Code Snippet]]
This error occurs because trigger_gmm has not been defined in the expected scope for the code located outside of the function. Let's explore how to resolve this issue.
Step-by-Step Solution
1. Declare the Global Variable Properly
To use a variable as a global variable inside a function, you need to declare it as global within that function. This informs Python that you intend to use the globally defined version of the variable instead of creating a local one.
However, it’s also important to declare trigger_gmm as global in the main function where it's first assigned a value.
Correct Declaration:
[[See Video to Reveal this Text or Code Snippet]]
2. Removal of Unnecessary Parentheses
In the original code, there is a typo where trigger_gmm() is mistakenly referenced. Since this is meant to assign 0 to trigger_gmm, parentheses should be removed to prevent errors:
[[See Video to Reveal this Text or Code Snippet]]
3. Restructure Code for Global Access
The placement of the if __name__ == "__main__": block is also crucial. This block should be placed outside of any loops or functions and executed first. You need to ensure that the program starts at the main entry point properly before any infinite loops are initiated.
4. Implementing the Changes
Now that we've identified the issues, let's revise the entire code:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following these steps, you can confidently access and manipulate variables outside of functions in Python. Remember to declare your global variables properly and structure your code so that it executes in the correct scope. This knowledge not only aids in troubleshooting but also enhances your overall coding skills.
Whether you're working on a small project or a larger application, understanding variable scope in Python is fundamental. Happy coding!