filmov
tv
Graphics sliders for Teensy MCU’s
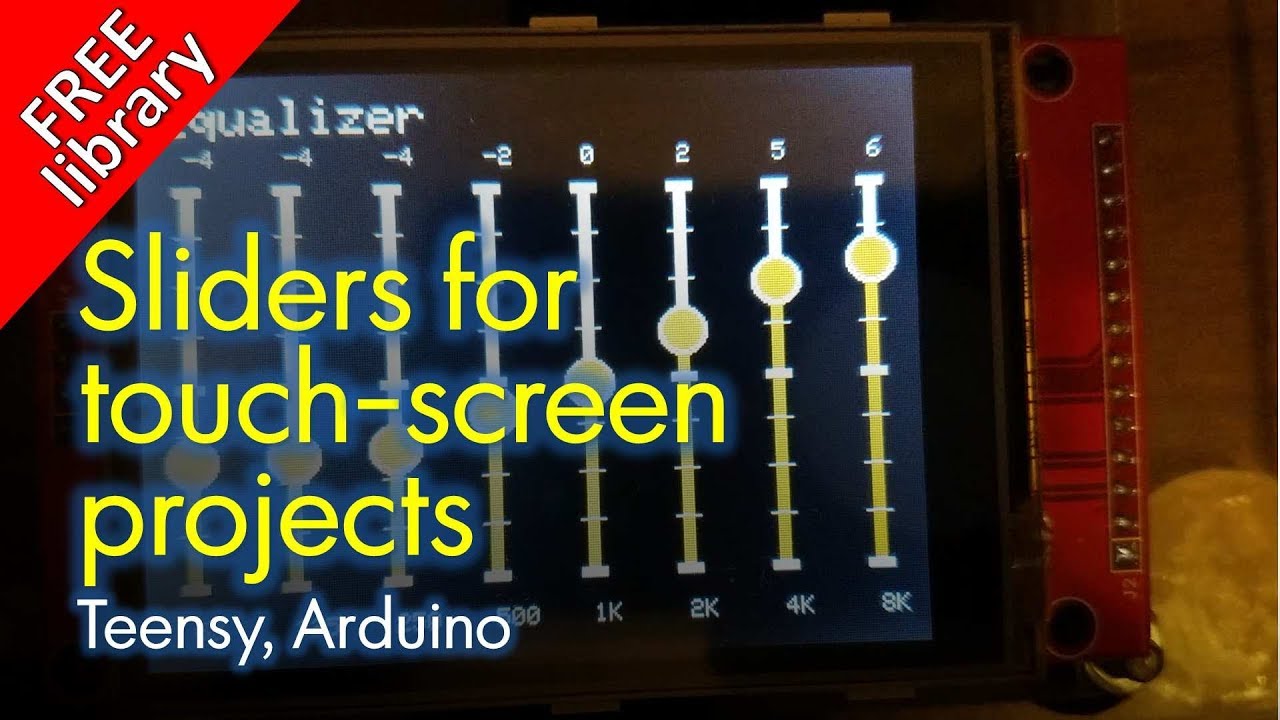
Показать описание
Use this simple library to add slider controls to your touchscreen projects. Code is written for the ILI9341_t3 library but can easily be adapted to any library that leverages the Adafruit_GFX library. Perfect for your Teensy projects.
Library at:
Complete list of methods
class SliderH
// class constructor
SliderH(ILI9341_t3 *disp, uint16_t left, uint16_t top, uint16_t wide, uint16_t sliderColor, uint16_t backColor, uint16_t handleColor);
// initializer
void init(float scaleLow, float scaleHi, float scale = 0.0, float snap = 0.0);
// method to draw complete slider
void draw(float val);
// method to move handle as user drags finger over handle, this method automatically looks for a valid range press, sets changed() to true
float slide(float x, float y);
// property to determine if the slider was just changed, draw and changed methods set to false
bool changed();
// way to reset colors (useful for drawing enabled or disabled)
void setColors(uint16_t sliderColor, uint16_t backColor, uint16_t handleColor);
// method to just set the handle color (useful for showing handle in green for OK value
void setHandleColor(uint16_t handleColor);
// method to just draw the handle (useful for quick update of handle color)
void drawSliderColor(bool val);
class SliderV
// class constructor
SliderV(ILI9341_t3 *disp, uint16_t left, uint16_t top, uint16_t high, uint16_t sliderColor, uint16_t backColor, uint16_t handleColor);
// initializer
void init(float scaleLow, float scaleHi, float scale = 0.0, float snap = 0.0);
// method to draw complete slider
void draw(float val);
// method to move handle as user drags finger over handle, this method automatically looks for a valid range press, sets changed() to true
float slide(uint16_t x, uint16_t y);
// property to determine if the slider was just changed, draw and changed methods set to false
bool changed();
// way to reset colors (useful for drawing enabled or disabled)
void setColors(uint16_t sliderColor, uint16_t backColor, uint16_t handleColor);
// method to just set the handle color (useful for showing handle in green for OK value)
void setHandleColor(uint16_t handleColor);
// method to just draw the handle (useful for quick update of handle color)
void drawSliderColor(bool val);
class SliderOnOff
// class constructor
SliderOnOff(ILI9341_t3 *disp, uint16_t left, uint16_t top, uint16_t width, uint16_t height, uint16_t sliderColor, uint16_t backColor, uint16_t OnColor, uint16_t OffColor);
// method to draw complete slider
void draw(bool val);
// method to move handle as user drags finger over handle, this method automatically looks for a valid range press, sets changed() to true
bool slide(float x,float y);
// property to determine if the slider was just changed, draw and changed methods set to false
bool changed();
Library at:
Complete list of methods
class SliderH
// class constructor
SliderH(ILI9341_t3 *disp, uint16_t left, uint16_t top, uint16_t wide, uint16_t sliderColor, uint16_t backColor, uint16_t handleColor);
// initializer
void init(float scaleLow, float scaleHi, float scale = 0.0, float snap = 0.0);
// method to draw complete slider
void draw(float val);
// method to move handle as user drags finger over handle, this method automatically looks for a valid range press, sets changed() to true
float slide(float x, float y);
// property to determine if the slider was just changed, draw and changed methods set to false
bool changed();
// way to reset colors (useful for drawing enabled or disabled)
void setColors(uint16_t sliderColor, uint16_t backColor, uint16_t handleColor);
// method to just set the handle color (useful for showing handle in green for OK value
void setHandleColor(uint16_t handleColor);
// method to just draw the handle (useful for quick update of handle color)
void drawSliderColor(bool val);
class SliderV
// class constructor
SliderV(ILI9341_t3 *disp, uint16_t left, uint16_t top, uint16_t high, uint16_t sliderColor, uint16_t backColor, uint16_t handleColor);
// initializer
void init(float scaleLow, float scaleHi, float scale = 0.0, float snap = 0.0);
// method to draw complete slider
void draw(float val);
// method to move handle as user drags finger over handle, this method automatically looks for a valid range press, sets changed() to true
float slide(uint16_t x, uint16_t y);
// property to determine if the slider was just changed, draw and changed methods set to false
bool changed();
// way to reset colors (useful for drawing enabled or disabled)
void setColors(uint16_t sliderColor, uint16_t backColor, uint16_t handleColor);
// method to just set the handle color (useful for showing handle in green for OK value)
void setHandleColor(uint16_t handleColor);
// method to just draw the handle (useful for quick update of handle color)
void drawSliderColor(bool val);
class SliderOnOff
// class constructor
SliderOnOff(ILI9341_t3 *disp, uint16_t left, uint16_t top, uint16_t width, uint16_t height, uint16_t sliderColor, uint16_t backColor, uint16_t OnColor, uint16_t OffColor);
// method to draw complete slider
void draw(bool val);
// method to move handle as user drags finger over handle, this method automatically looks for a valid range press, sets changed() to true
bool slide(float x,float y);
// property to determine if the slider was just changed, draw and changed methods set to false
bool changed();
Комментарии