filmov
tv
Troubleshooting 3D Array Access Errors in C#
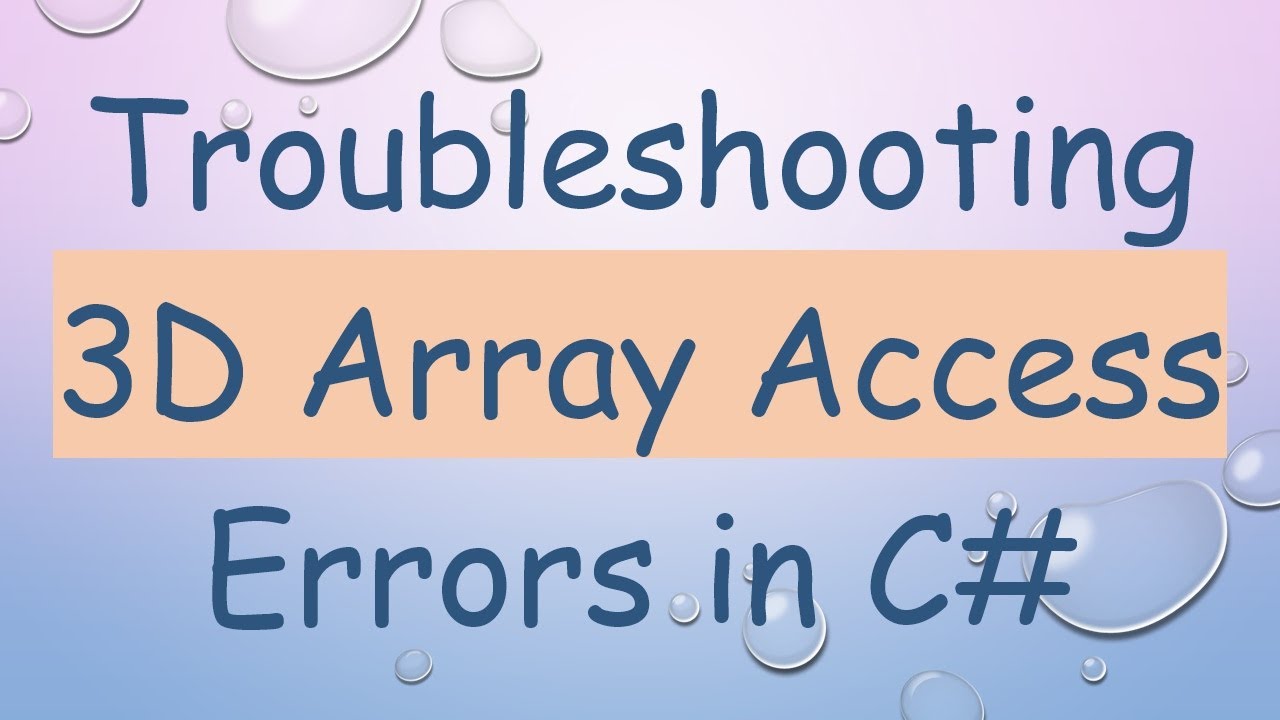
Показать описание
Learn how to resolve common errors when accessing elements in a 3D array using C# with proper syntax and indexing techniques.
---
Disclaimer/Disclosure - Portions of this content were created using Generative AI tools, which may result in inaccuracies or misleading information in the video. Please keep this in mind before making any decisions or taking any actions based on the content. If you have any concerns, don't hesitate to leave a comment. Thanks.
---
Understanding how to work with multi-dimensional arrays in C is essential, especially when dealing with 3D arrays. If you're encountering an error with the access syntax myarray[0,1,1], there are several aspects of multidimensional arrays in C to consider.
Key Concepts of 3D Arrays in C
A 3D array is essentially an array of arrays which can be visualized as a cube of data organized in three dimensions. To effectively work with them, understanding C's syntax and indexing rules is crucial.
Syntax for Declaring a 3D Array
In C, you declare a 3D array as follows:
[[See Video to Reveal this Text or Code Snippet]]
Here, x, y, and z represent the size of the dimensions. A common mistake is not matching the indices with the actual dimensions initialized.
Why myarray[0,1,1] Might Cause an Error
Index Out of Range: Ensure that each index (0, 1, and 1) is within the bounds of the corresponding dimension. For example, if z is less than 2, accessing myarray[0,1,1] will lead to an IndexOutOfRangeException.
Array Initialization: Double-check that the array is initialized properly. Attempting to access an element of an uninitialized array will lead to errors.
Correct Syntax: Ensure your access follows the correct format. If you're transitioning from a different programming language, C syntax might appear slightly different.
Best Practices
Bounds Checking: Always check that the indices you use fall between 0 and length-1 for each dimension.
Error Handling: Implement try-catch blocks to handle potential exceptions gracefully.
Debugging: Use debugging tools or Console.WriteLine statements to verify the dimensional sizes and the validity of indices.
Example Code:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding and correctly using 3D arrays in C boils down to ensuring correct syntax, proper initialization, and careful index management. By being mindful of these nuances, you can avoid common issues and make the best use of C's powerful array handling capabilities.
---
Disclaimer/Disclosure - Portions of this content were created using Generative AI tools, which may result in inaccuracies or misleading information in the video. Please keep this in mind before making any decisions or taking any actions based on the content. If you have any concerns, don't hesitate to leave a comment. Thanks.
---
Understanding how to work with multi-dimensional arrays in C is essential, especially when dealing with 3D arrays. If you're encountering an error with the access syntax myarray[0,1,1], there are several aspects of multidimensional arrays in C to consider.
Key Concepts of 3D Arrays in C
A 3D array is essentially an array of arrays which can be visualized as a cube of data organized in three dimensions. To effectively work with them, understanding C's syntax and indexing rules is crucial.
Syntax for Declaring a 3D Array
In C, you declare a 3D array as follows:
[[See Video to Reveal this Text or Code Snippet]]
Here, x, y, and z represent the size of the dimensions. A common mistake is not matching the indices with the actual dimensions initialized.
Why myarray[0,1,1] Might Cause an Error
Index Out of Range: Ensure that each index (0, 1, and 1) is within the bounds of the corresponding dimension. For example, if z is less than 2, accessing myarray[0,1,1] will lead to an IndexOutOfRangeException.
Array Initialization: Double-check that the array is initialized properly. Attempting to access an element of an uninitialized array will lead to errors.
Correct Syntax: Ensure your access follows the correct format. If you're transitioning from a different programming language, C syntax might appear slightly different.
Best Practices
Bounds Checking: Always check that the indices you use fall between 0 and length-1 for each dimension.
Error Handling: Implement try-catch blocks to handle potential exceptions gracefully.
Debugging: Use debugging tools or Console.WriteLine statements to verify the dimensional sizes and the validity of indices.
Example Code:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding and correctly using 3D arrays in C boils down to ensuring correct syntax, proper initialization, and careful index management. By being mindful of these nuances, you can avoid common issues and make the best use of C's powerful array handling capabilities.