filmov
tv
Write a Tetris game in JavaScript
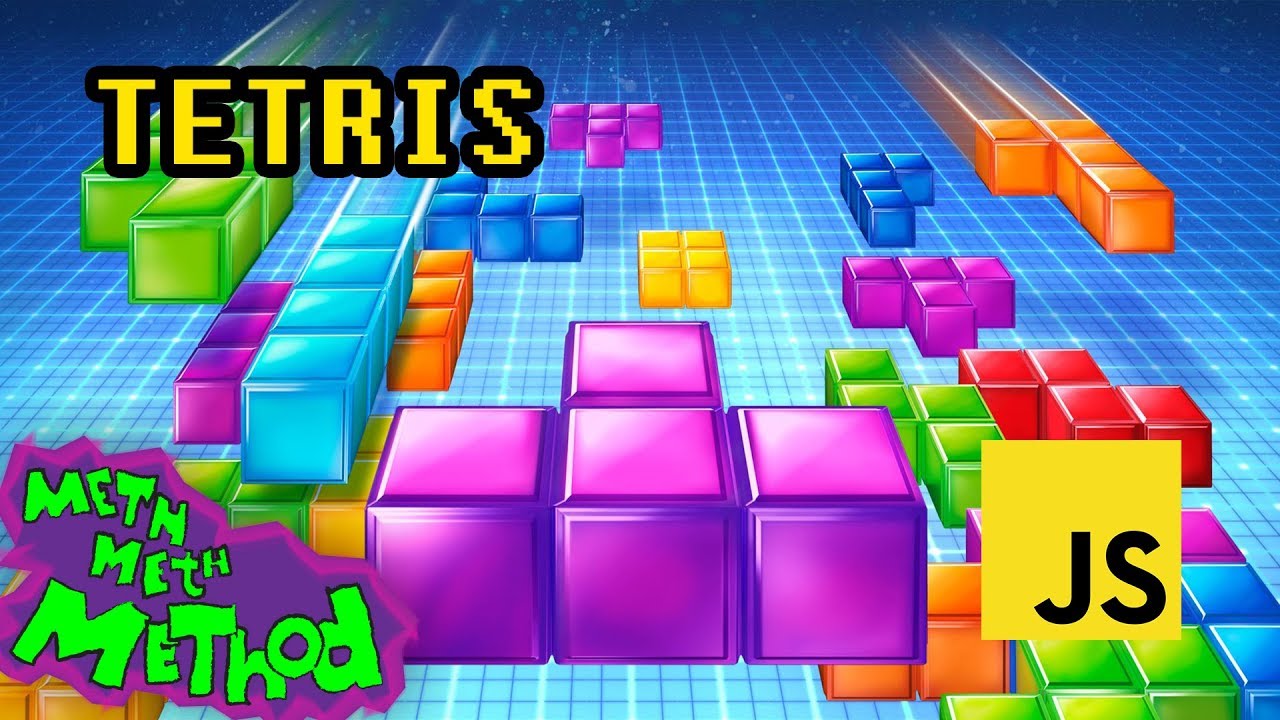
Показать описание
In this episode I write a Tetris game in JavaScript from scratch.
We will look at
* Drawing on a canvas
* Rotating a matrix
* Rudimentary requestAnimationFrame listening
* Handling keyboard input
Since we are writing ES6 this code might not run on all browsers yet. I was running Chrome 53 when I wrote this and if you wish to follow I recommend you do too.
The initial HTML can be found here:
Source code with step-by-step commits:
Music:
Demoscene Time Machine
We will look at
* Drawing on a canvas
* Rotating a matrix
* Rudimentary requestAnimationFrame listening
* Handling keyboard input
Since we are writing ES6 this code might not run on all browsers yet. I was running Chrome 53 when I wrote this and if you wish to follow I recommend you do too.
The initial HTML can be found here:
Source code with step-by-step commits:
Music:
Demoscene Time Machine
Programming a Tetris Game in C# - Full Guide
Coding TETRIS in 3 Minutes
Detailed Tetris Tutorial in Python
Write a Tetris game in JavaScript
Tetris Part 1 - Class Grid
Creating Tetris in Python with pygame - Beginner Tutorial (OOP)
Build a Tetris-game using ChatGPT in 2 minutes #chatgpt #openai
Code-It-Yourself! Tetris - Programming from Scratch (Quick and Simple C++)
【ChatGPT】How to Create a Simple Tetris Game in 3 steps🎮 #pygame #Python
Pygame Tutorial - Creating Tetris
#shorts Tetris Gummy game
Creating Tetris in C++ with raylib - Beginner Tutorial (OOP)
Learn to Code a Tetris game in Python With Me
Let's code Tetris Game in Python
Create Tetris Game Using Vanilla JavaScript
Coding Tetris in JavaScript (HTML and CSS)
ChatGPT creates Tetris
Javascript tutorial: Code a Tetris game, part 1 (setup, moving/rotating pieces)
1 - Unity Game Development - Create A Tetris Game - Game Preview - Unity Tetris Game Tutorial
Learn How to Creating A Tetris Game In 6 Minutes Using C++
Code Tetris: JavaScript Tutorial for Beginners
Building TETRIS in 115 LINES of python code
If Tetris Blocks Had Feelings... #tetris #animation #cartoon
[Java] Create a Tetris Game in Swing with Java 2D - Part 1
Комментарии