filmov
tv
how to modify a list while iterating (intermediate) anthony explains #402
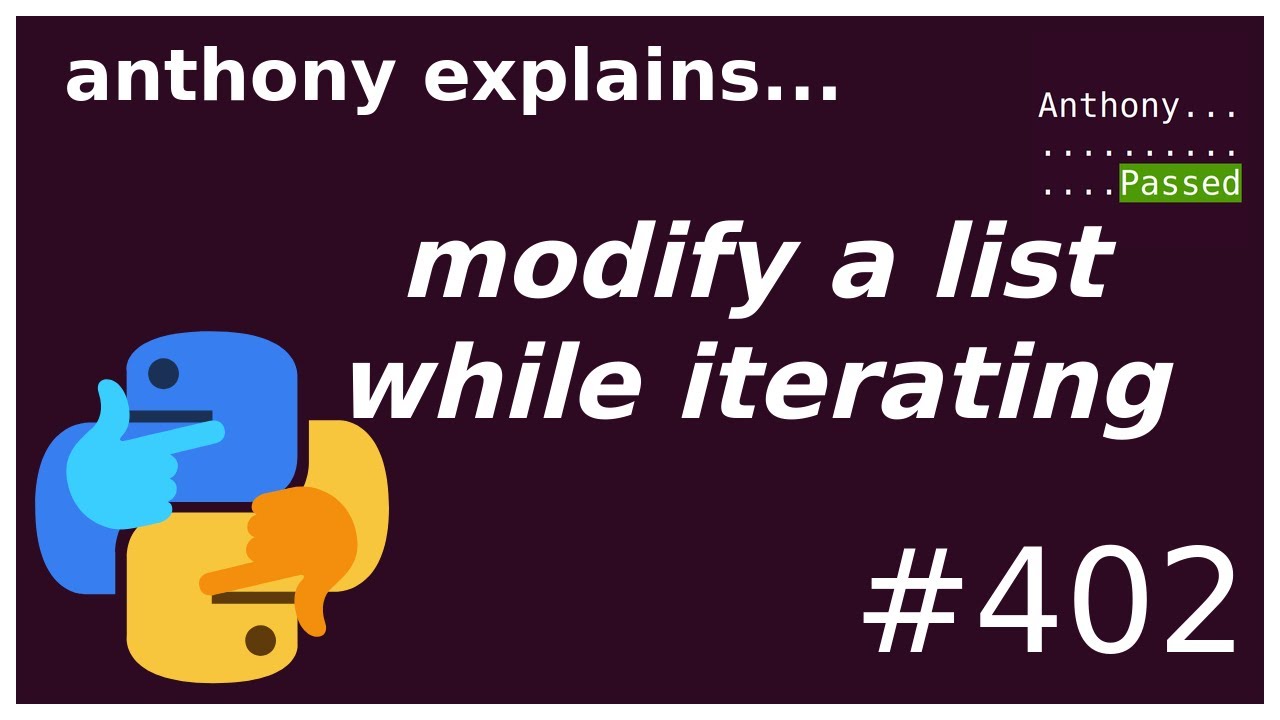
Показать описание
*normally* you can't modify a list while iterating over it but I show a little trick that makes it possible. I also show how you might refactor "iterating a dict while modifying" as well!
==========
I won't ask for subscriptions / likes / comments in videos but it really helps the channel. If you have any suggestions or things you'd like to see please comment below!
==========
I won't ask for subscriptions / likes / comments in videos but it really helps the channel. If you have any suggestions or things you'd like to see please comment below!
The Fastest Way to Modify a List in C# | Coding Demo
how to modify a list while iterating (intermediate) anthony explains #402
How to modify Office 365 distribution list from outlook
Modify a List Using List Slicing
How to modify the list
How to modify the elements in a Python list | Python Crash Course - Exercise 3.4 & 3.5
Python | Modify List Item
How to modify a Query
How to Modify Money & Power up Items in High Sea Saga DX with GameGuardian - HIGH SEA SAGA DX
How to Create and Modify Numbered Lists in Microsoft Word
How to Modify SharePoint List Toolbar Buttons with List Formatting
How to modify list in python with examples | List Part 2
6 Ways to Edit Any Scene — Essential Film & Video Editing Techniques Explained [Shot List Ep. 10...
How Can I Modify My Python Function to Output a List in a Single Line with and?
Word for Dissertations: Modify Spacing in the Table of Contents
How to Modify Python Script to Format JSON Output as a List?
How To Create List In Python and How To Modify List
How to Modify Related List Quick Links in Salesforce
Mathematica: How to modify a position on a list?
How to create & modify Multilevel list in Word: Step by step tutorial on heading numbering in Wo...
How To Modify The List View In Square Takeoff
How to Add and Modify Heading Numbers in Microsoft Word (PC & Mac)
Drop-down lists in Word: Insert, modify, use a format to style contents
RRB NTPC Form Correction Kaise Kare 2024 | How to modify RRB NTPC Graduate Level Form 2024
Комментарии