filmov
tv
Python: Palindrome Check - LeetCode Challenge
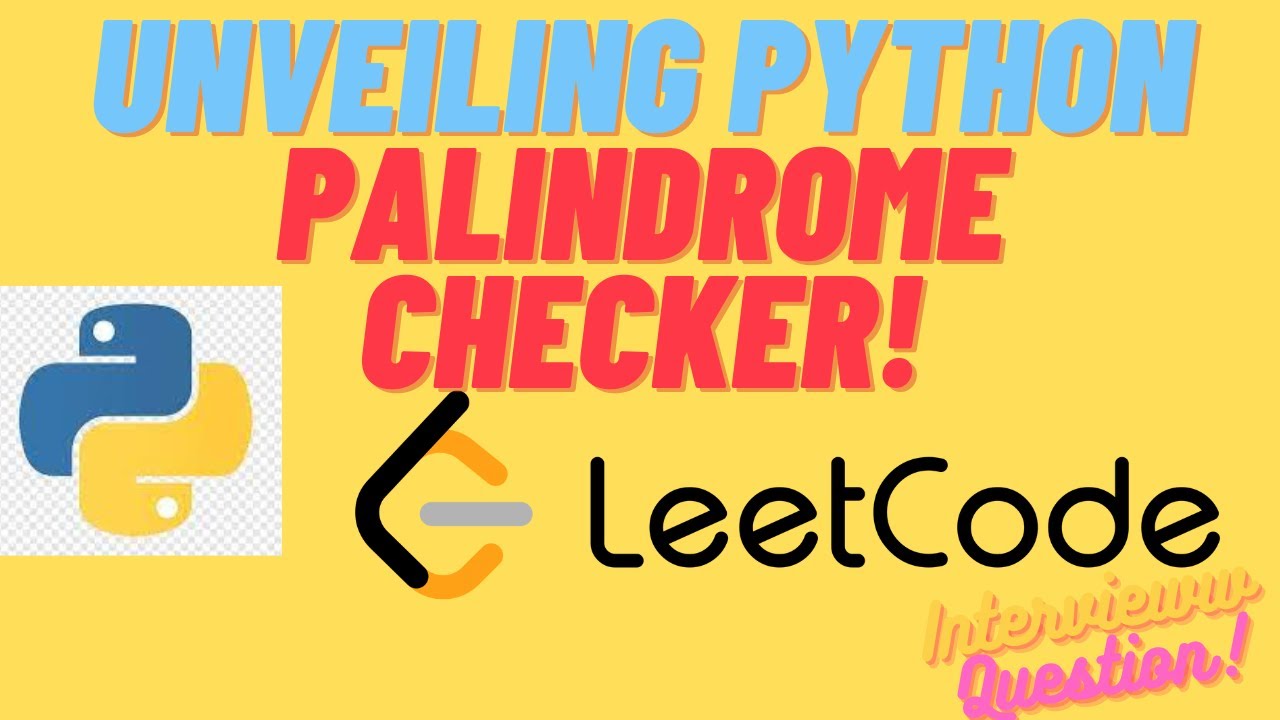
Показать описание
Greetings, my fellow coding enthusiasts! Welcome to an exciting journey through the realm of programming and problem-solving. I'm Vatsal, your guide for today's adventure, and we have a special treat in store. We'll be delving into a Python solution for a LeetCode problem, specifically, the "Valid Palindrome" challenge.
The Significance of Palindromes 🧐
Before we jump into the code, let's discuss why palindromes are relevant in the world of programming. Palindromes aren't just wordplay; they serve as fantastic tools for enhancing problem-solving skills. Whether you're a beginner or an experienced coder, tackling problems related to palindromes can be both enjoyable and instructive. You'll gain a deeper insight into string manipulation, character comparison, and algorithmic thinking.
Our Challenge: Valid Palindrome 📜
So, what's the challenge we're facing? LeetCode presents us with a problem: Given a string s, we need to determine if it's a valid palindrome. But what does that mean?
In this context, a string is considered a palindrome if, after converting all uppercase letters to lowercase and removing all non-alphanumeric characters, it reads the same forwards and backward. Alphanumeric characters encompass both letters and numbers.
This task may seem simple, but it's multifaceted. We must ensure our solution handles mixed-case strings and special characters. Moreover, it should be case-insensitive. For instance, "Radar" and "radar" should both be recognized as valid palindromes.
Our Approach: Cleaning the Input 🧹
Our journey begins by preparing the input. Picture dealing with a string containing uppercase letters, special characters, and spaces. To ensure our palindrome check remains unaffected by these factors, we adopt a two-step process.
In the first step, we use regular expressions to remove all non-alphanumeric characters and underscores from the string. This step ensures that special characters don't interfere with our palindrome check. Next, we convert the entire string to lowercase. This step makes our check case-insensitive.
The Heart of It: Palindrome Check ❤️
Now comes the exciting part - the palindrome check itself. To accomplish this, we employ a two-pointer approach. Visualize having two pointers, one at the beginning of the string (let's call it "left") and another at the end (we'll call it "right"). Initially, "left" points to the first character of the string, and "right" points to the last character.
The magic happens in a while loop. We continue looping as long as "left" is less than "right." This logic is sound because we only need to compare characters until they converge in the middle of the string.
Inside the loop, we compare the characters at "left" and "right." If they don't match, it's a clear sign that the string can't be a palindrome, and we return False. However, if the characters do match, we increment "left" and decrement "right," effectively moving them closer to each other.
Success: Return True! ✅
If the while loop completes without encountering any mismatches, it's a strong indication that our string is indeed a palindrome. In this case, we return True, confirming that the input string passed the palindrome test.
LeetCode Context 🏆
As an added bonus, I must mention that this solution isn't just for learning purposes; it's also an efficient way to solve a LeetCode problem! In fact, it surpasses around 85% of submissions on LeetCode, boasting a runtime of just 48 milliseconds.
By taking on this challenge, you're not only mastering palindrome-checking techniques but also honing your problem-solving skills on a real coding platform. It's a double win – learning and achievement combined!
Conclusion 🎉
And there you have it, folks! We've embarked on a journey through the intricacies of checking for palindromes in Python. From cleaning the input to implementing a two-pointer approach, you've seen it all.
But the adventure doesn't end here. To truly grasp these concepts, nothing beats hands-on practice. I encourage you to try out different strings, test edge cases, and explore variations of this problem. The more you code, the more proficient you'll become.
Until our next coding escapade, keep exploring, keep coding, and most importantly, keep having fun on your programming journey. Happy coding, my friends!
Don't forget to hit that play button, like this video, share it with your fellow coders, and subscribe for more exciting coding adventures. Let's continue to learn and grow together!
#PythonProgramming
#LeetCodeChallenge
#PalindromeChecker
#CodingChallenge
#ProgrammingTutorial
#Algorithm
#StringManipulation
#ProblemSolving
#TechExplained
#ProgrammingJourney
#LearnToCode
#CodingTips
#CodeExplained
#TechEducation
#ProgrammingSkills
#PythonTutorial
#ProgrammingCommunity
#ComputerScience
#CodeWithMe
The Significance of Palindromes 🧐
Before we jump into the code, let's discuss why palindromes are relevant in the world of programming. Palindromes aren't just wordplay; they serve as fantastic tools for enhancing problem-solving skills. Whether you're a beginner or an experienced coder, tackling problems related to palindromes can be both enjoyable and instructive. You'll gain a deeper insight into string manipulation, character comparison, and algorithmic thinking.
Our Challenge: Valid Palindrome 📜
So, what's the challenge we're facing? LeetCode presents us with a problem: Given a string s, we need to determine if it's a valid palindrome. But what does that mean?
In this context, a string is considered a palindrome if, after converting all uppercase letters to lowercase and removing all non-alphanumeric characters, it reads the same forwards and backward. Alphanumeric characters encompass both letters and numbers.
This task may seem simple, but it's multifaceted. We must ensure our solution handles mixed-case strings and special characters. Moreover, it should be case-insensitive. For instance, "Radar" and "radar" should both be recognized as valid palindromes.
Our Approach: Cleaning the Input 🧹
Our journey begins by preparing the input. Picture dealing with a string containing uppercase letters, special characters, and spaces. To ensure our palindrome check remains unaffected by these factors, we adopt a two-step process.
In the first step, we use regular expressions to remove all non-alphanumeric characters and underscores from the string. This step ensures that special characters don't interfere with our palindrome check. Next, we convert the entire string to lowercase. This step makes our check case-insensitive.
The Heart of It: Palindrome Check ❤️
Now comes the exciting part - the palindrome check itself. To accomplish this, we employ a two-pointer approach. Visualize having two pointers, one at the beginning of the string (let's call it "left") and another at the end (we'll call it "right"). Initially, "left" points to the first character of the string, and "right" points to the last character.
The magic happens in a while loop. We continue looping as long as "left" is less than "right." This logic is sound because we only need to compare characters until they converge in the middle of the string.
Inside the loop, we compare the characters at "left" and "right." If they don't match, it's a clear sign that the string can't be a palindrome, and we return False. However, if the characters do match, we increment "left" and decrement "right," effectively moving them closer to each other.
Success: Return True! ✅
If the while loop completes without encountering any mismatches, it's a strong indication that our string is indeed a palindrome. In this case, we return True, confirming that the input string passed the palindrome test.
LeetCode Context 🏆
As an added bonus, I must mention that this solution isn't just for learning purposes; it's also an efficient way to solve a LeetCode problem! In fact, it surpasses around 85% of submissions on LeetCode, boasting a runtime of just 48 milliseconds.
By taking on this challenge, you're not only mastering palindrome-checking techniques but also honing your problem-solving skills on a real coding platform. It's a double win – learning and achievement combined!
Conclusion 🎉
And there you have it, folks! We've embarked on a journey through the intricacies of checking for palindromes in Python. From cleaning the input to implementing a two-pointer approach, you've seen it all.
But the adventure doesn't end here. To truly grasp these concepts, nothing beats hands-on practice. I encourage you to try out different strings, test edge cases, and explore variations of this problem. The more you code, the more proficient you'll become.
Until our next coding escapade, keep exploring, keep coding, and most importantly, keep having fun on your programming journey. Happy coding, my friends!
Don't forget to hit that play button, like this video, share it with your fellow coders, and subscribe for more exciting coding adventures. Let's continue to learn and grow together!
#PythonProgramming
#LeetCodeChallenge
#PalindromeChecker
#CodingChallenge
#ProgrammingTutorial
#Algorithm
#StringManipulation
#ProblemSolving
#TechExplained
#ProgrammingJourney
#LearnToCode
#CodingTips
#CodeExplained
#TechEducation
#ProgrammingSkills
#PythonTutorial
#ProgrammingCommunity
#ComputerScience
#CodeWithMe
Комментарии